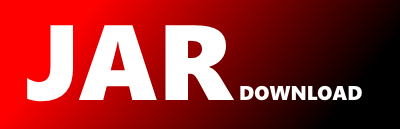
com.pulumi.azurenative.kusto.EventGridDataConnectionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto;
import com.pulumi.azurenative.kusto.enums.BlobStorageEventType;
import com.pulumi.azurenative.kusto.enums.DatabaseRouting;
import com.pulumi.azurenative.kusto.enums.EventGridDataFormat;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EventGridDataConnectionArgs extends com.pulumi.resources.ResourceArgs {
public static final EventGridDataConnectionArgs Empty = new EventGridDataConnectionArgs();
/**
* The name of blob storage event type to process.
*
*/
@Import(name="blobStorageEventType")
private @Nullable Output> blobStorageEventType;
/**
* @return The name of blob storage event type to process.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy