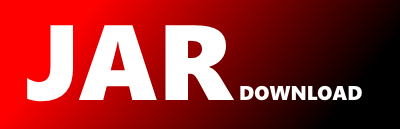
com.pulumi.azurenative.logic.Workflow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.logic.WorkflowArgs;
import com.pulumi.azurenative.logic.outputs.FlowAccessControlConfigurationResponse;
import com.pulumi.azurenative.logic.outputs.FlowEndpointsConfigurationResponse;
import com.pulumi.azurenative.logic.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.logic.outputs.ResourceReferenceResponse;
import com.pulumi.azurenative.logic.outputs.SkuResponse;
import com.pulumi.azurenative.logic.outputs.WorkflowParameterResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The workflow type.
* Azure REST API version: 2019-05-01. Prior API version in Azure Native 1.x: 2019-05-01.
*
* Other available API versions: 2015-02-01-preview, 2016-06-01, 2018-07-01-preview.
*
* ## Example Usage
* ### Create or update a workflow
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.logic.Workflow;
* import com.pulumi.azurenative.logic.WorkflowArgs;
* import com.pulumi.azurenative.logic.inputs.ResourceReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var workflow = new Workflow("workflow", WorkflowArgs.builder()
* .definition(Map.ofEntries(
* Map.entry("$schema", "https://schema.management.azure.com/providers/Microsoft.Logic/schemas/2016-06-01/workflowdefinition.json#"),
* Map.entry("actions", Map.of("Find_pet_by_ID", Map.ofEntries(
* Map.entry("inputs", Map.ofEntries(
* Map.entry("host", Map.of("connection", Map.of("name", "}{@literal @}{@code parameters('$connections')['test-custom-connector']['connectionId']"))),
* Map.entry("method", "get"),
* Map.entry("path", "/pet/}{@literal @}{{@code encodeURIComponent('1')}}{@code ")
* )),
* Map.entry("runAfter", ),
* Map.entry("type", "ApiConnection")
* ))),
* Map.entry("contentVersion", "1.0.0.0"),
* Map.entry("outputs", ),
* Map.entry("parameters", Map.of("$connections", Map.ofEntries(
* Map.entry("defaultValue", ),
* Map.entry("type", "Object")
* ))),
* Map.entry("triggers", Map.of("manual", Map.ofEntries(
* Map.entry("inputs", Map.of("schema", )),
* Map.entry("kind", "Http"),
* Map.entry("type", "Request")
* )))
* ))
* .integrationAccount(ResourceReferenceArgs.builder()
* .id("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Logic/integrationAccounts/test-integration-account")
* .build())
* .location("brazilsouth")
* .parameters(Map.of("$connections", Map.of("value", Map.of("test-custom-connector", Map.ofEntries(
* Map.entry("connectionId", "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-resource-group/providers/Microsoft.Web/connections/test-custom-connector"),
* Map.entry("connectionName", "test-custom-connector"),
* Map.entry("id", "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/providers/Microsoft.Web/locations/brazilsouth/managedApis/test-custom-connector")
* )))))
* .resourceGroupName("test-resource-group")
* .tags()
* .workflowName("test-workflow")
* .build());
*
* }}{@code
* }}{@code
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:logic:Workflow myresource1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Logic/workflows/{workflowName}
* ```
*
*/
@ResourceType(type="azure-native:logic:Workflow")
public class Workflow extends com.pulumi.resources.CustomResource {
/**
* The access control configuration.
*
*/
@Export(name="accessControl", refs={FlowAccessControlConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ FlowAccessControlConfigurationResponse> accessControl;
/**
* @return The access control configuration.
*
*/
public Output> accessControl() {
return Codegen.optional(this.accessControl);
}
/**
* Gets the access endpoint.
*
*/
@Export(name="accessEndpoint", refs={String.class}, tree="[0]")
private Output accessEndpoint;
/**
* @return Gets the access endpoint.
*
*/
public Output accessEndpoint() {
return this.accessEndpoint;
}
/**
* Gets the changed time.
*
*/
@Export(name="changedTime", refs={String.class}, tree="[0]")
private Output changedTime;
/**
* @return Gets the changed time.
*
*/
public Output changedTime() {
return this.changedTime;
}
/**
* Gets the created time.
*
*/
@Export(name="createdTime", refs={String.class}, tree="[0]")
private Output createdTime;
/**
* @return Gets the created time.
*
*/
public Output createdTime() {
return this.createdTime;
}
/**
* The definition.
*
*/
@Export(name="definition", refs={Object.class}, tree="[0]")
private Output* @Nullable */ Object> definition;
/**
* @return The definition.
*
*/
public Output> definition() {
return Codegen.optional(this.definition);
}
/**
* The endpoints configuration.
*
*/
@Export(name="endpointsConfiguration", refs={FlowEndpointsConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ FlowEndpointsConfigurationResponse> endpointsConfiguration;
/**
* @return The endpoints configuration.
*
*/
public Output> endpointsConfiguration() {
return Codegen.optional(this.endpointsConfiguration);
}
/**
* Managed service identity properties.
*
*/
@Export(name="identity", refs={ManagedServiceIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ ManagedServiceIdentityResponse> identity;
/**
* @return Managed service identity properties.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The integration account.
*
*/
@Export(name="integrationAccount", refs={ResourceReferenceResponse.class}, tree="[0]")
private Output* @Nullable */ ResourceReferenceResponse> integrationAccount;
/**
* @return The integration account.
*
*/
public Output> integrationAccount() {
return Codegen.optional(this.integrationAccount);
}
/**
* The integration service environment.
*
*/
@Export(name="integrationServiceEnvironment", refs={ResourceReferenceResponse.class}, tree="[0]")
private Output* @Nullable */ ResourceReferenceResponse> integrationServiceEnvironment;
/**
* @return The integration service environment.
*
*/
public Output> integrationServiceEnvironment() {
return Codegen.optional(this.integrationServiceEnvironment);
}
/**
* The resource location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The resource location.
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* Gets the resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Gets the resource name.
*
*/
public Output name() {
return this.name;
}
/**
* The parameters.
*
*/
@Export(name="parameters", refs={Map.class,String.class,WorkflowParameterResponse.class}, tree="[0,1,2]")
private Output* @Nullable */ Map> parameters;
/**
* @return The parameters.
*
*/
public Output>> parameters() {
return Codegen.optional(this.parameters);
}
/**
* Gets the provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Gets the provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The sku.
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output sku;
/**
* @return The sku.
*
*/
public Output sku() {
return this.sku;
}
/**
* The state.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> state;
/**
* @return The state.
*
*/
public Output> state() {
return Codegen.optional(this.state);
}
/**
* The resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return The resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Gets the resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Gets the resource type.
*
*/
public Output type() {
return this.type;
}
/**
* Gets the version.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output version;
/**
* @return Gets the version.
*
*/
public Output version() {
return this.version;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Workflow(java.lang.String name) {
this(name, WorkflowArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Workflow(java.lang.String name, WorkflowArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Workflow(java.lang.String name, WorkflowArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:logic:Workflow", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Workflow(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:logic:Workflow", name, null, makeResourceOptions(options, id), false);
}
private static WorkflowArgs makeArgs(WorkflowArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? WorkflowArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:logic/v20150201preview:Workflow").build()),
Output.of(Alias.builder().type("azure-native:logic/v20160601:Workflow").build()),
Output.of(Alias.builder().type("azure-native:logic/v20180701preview:Workflow").build()),
Output.of(Alias.builder().type("azure-native:logic/v20190501:Workflow").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Workflow get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Workflow(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy