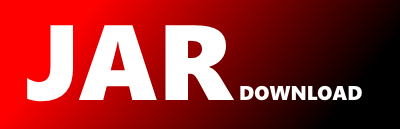
com.pulumi.azurenative.logic.inputs.X12ProcessingSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.util.Objects;
/**
* The X12 processing settings.
*
*/
public final class X12ProcessingSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final X12ProcessingSettingsArgs Empty = new X12ProcessingSettingsArgs();
/**
* The value indicating whether to convert numerical type to implied decimal.
*
*/
@Import(name="convertImpliedDecimal", required=true)
private Output convertImpliedDecimal;
/**
* @return The value indicating whether to convert numerical type to implied decimal.
*
*/
public Output convertImpliedDecimal() {
return this.convertImpliedDecimal;
}
/**
* The value indicating whether to create empty xml tags for trailing separators.
*
*/
@Import(name="createEmptyXmlTagsForTrailingSeparators", required=true)
private Output createEmptyXmlTagsForTrailingSeparators;
/**
* @return The value indicating whether to create empty xml tags for trailing separators.
*
*/
public Output createEmptyXmlTagsForTrailingSeparators() {
return this.createEmptyXmlTagsForTrailingSeparators;
}
/**
* The value indicating whether to mask security information.
*
*/
@Import(name="maskSecurityInfo", required=true)
private Output maskSecurityInfo;
/**
* @return The value indicating whether to mask security information.
*
*/
public Output maskSecurityInfo() {
return this.maskSecurityInfo;
}
/**
* The value indicating whether to preserve interchange.
*
*/
@Import(name="preserveInterchange", required=true)
private Output preserveInterchange;
/**
* @return The value indicating whether to preserve interchange.
*
*/
public Output preserveInterchange() {
return this.preserveInterchange;
}
/**
* The value indicating whether to suspend interchange on error.
*
*/
@Import(name="suspendInterchangeOnError", required=true)
private Output suspendInterchangeOnError;
/**
* @return The value indicating whether to suspend interchange on error.
*
*/
public Output suspendInterchangeOnError() {
return this.suspendInterchangeOnError;
}
/**
* The value indicating whether to use dot as decimal separator.
*
*/
@Import(name="useDotAsDecimalSeparator", required=true)
private Output useDotAsDecimalSeparator;
/**
* @return The value indicating whether to use dot as decimal separator.
*
*/
public Output useDotAsDecimalSeparator() {
return this.useDotAsDecimalSeparator;
}
private X12ProcessingSettingsArgs() {}
private X12ProcessingSettingsArgs(X12ProcessingSettingsArgs $) {
this.convertImpliedDecimal = $.convertImpliedDecimal;
this.createEmptyXmlTagsForTrailingSeparators = $.createEmptyXmlTagsForTrailingSeparators;
this.maskSecurityInfo = $.maskSecurityInfo;
this.preserveInterchange = $.preserveInterchange;
this.suspendInterchangeOnError = $.suspendInterchangeOnError;
this.useDotAsDecimalSeparator = $.useDotAsDecimalSeparator;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(X12ProcessingSettingsArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private X12ProcessingSettingsArgs $;
public Builder() {
$ = new X12ProcessingSettingsArgs();
}
public Builder(X12ProcessingSettingsArgs defaults) {
$ = new X12ProcessingSettingsArgs(Objects.requireNonNull(defaults));
}
/**
* @param convertImpliedDecimal The value indicating whether to convert numerical type to implied decimal.
*
* @return builder
*
*/
public Builder convertImpliedDecimal(Output convertImpliedDecimal) {
$.convertImpliedDecimal = convertImpliedDecimal;
return this;
}
/**
* @param convertImpliedDecimal The value indicating whether to convert numerical type to implied decimal.
*
* @return builder
*
*/
public Builder convertImpliedDecimal(Boolean convertImpliedDecimal) {
return convertImpliedDecimal(Output.of(convertImpliedDecimal));
}
/**
* @param createEmptyXmlTagsForTrailingSeparators The value indicating whether to create empty xml tags for trailing separators.
*
* @return builder
*
*/
public Builder createEmptyXmlTagsForTrailingSeparators(Output createEmptyXmlTagsForTrailingSeparators) {
$.createEmptyXmlTagsForTrailingSeparators = createEmptyXmlTagsForTrailingSeparators;
return this;
}
/**
* @param createEmptyXmlTagsForTrailingSeparators The value indicating whether to create empty xml tags for trailing separators.
*
* @return builder
*
*/
public Builder createEmptyXmlTagsForTrailingSeparators(Boolean createEmptyXmlTagsForTrailingSeparators) {
return createEmptyXmlTagsForTrailingSeparators(Output.of(createEmptyXmlTagsForTrailingSeparators));
}
/**
* @param maskSecurityInfo The value indicating whether to mask security information.
*
* @return builder
*
*/
public Builder maskSecurityInfo(Output maskSecurityInfo) {
$.maskSecurityInfo = maskSecurityInfo;
return this;
}
/**
* @param maskSecurityInfo The value indicating whether to mask security information.
*
* @return builder
*
*/
public Builder maskSecurityInfo(Boolean maskSecurityInfo) {
return maskSecurityInfo(Output.of(maskSecurityInfo));
}
/**
* @param preserveInterchange The value indicating whether to preserve interchange.
*
* @return builder
*
*/
public Builder preserveInterchange(Output preserveInterchange) {
$.preserveInterchange = preserveInterchange;
return this;
}
/**
* @param preserveInterchange The value indicating whether to preserve interchange.
*
* @return builder
*
*/
public Builder preserveInterchange(Boolean preserveInterchange) {
return preserveInterchange(Output.of(preserveInterchange));
}
/**
* @param suspendInterchangeOnError The value indicating whether to suspend interchange on error.
*
* @return builder
*
*/
public Builder suspendInterchangeOnError(Output suspendInterchangeOnError) {
$.suspendInterchangeOnError = suspendInterchangeOnError;
return this;
}
/**
* @param suspendInterchangeOnError The value indicating whether to suspend interchange on error.
*
* @return builder
*
*/
public Builder suspendInterchangeOnError(Boolean suspendInterchangeOnError) {
return suspendInterchangeOnError(Output.of(suspendInterchangeOnError));
}
/**
* @param useDotAsDecimalSeparator The value indicating whether to use dot as decimal separator.
*
* @return builder
*
*/
public Builder useDotAsDecimalSeparator(Output useDotAsDecimalSeparator) {
$.useDotAsDecimalSeparator = useDotAsDecimalSeparator;
return this;
}
/**
* @param useDotAsDecimalSeparator The value indicating whether to use dot as decimal separator.
*
* @return builder
*
*/
public Builder useDotAsDecimalSeparator(Boolean useDotAsDecimalSeparator) {
return useDotAsDecimalSeparator(Output.of(useDotAsDecimalSeparator));
}
public X12ProcessingSettingsArgs build() {
if ($.convertImpliedDecimal == null) {
throw new MissingRequiredPropertyException("X12ProcessingSettingsArgs", "convertImpliedDecimal");
}
if ($.createEmptyXmlTagsForTrailingSeparators == null) {
throw new MissingRequiredPropertyException("X12ProcessingSettingsArgs", "createEmptyXmlTagsForTrailingSeparators");
}
if ($.maskSecurityInfo == null) {
throw new MissingRequiredPropertyException("X12ProcessingSettingsArgs", "maskSecurityInfo");
}
if ($.preserveInterchange == null) {
throw new MissingRequiredPropertyException("X12ProcessingSettingsArgs", "preserveInterchange");
}
if ($.suspendInterchangeOnError == null) {
throw new MissingRequiredPropertyException("X12ProcessingSettingsArgs", "suspendInterchangeOnError");
}
if ($.useDotAsDecimalSeparator == null) {
throw new MissingRequiredPropertyException("X12ProcessingSettingsArgs", "useDotAsDecimalSeparator");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy