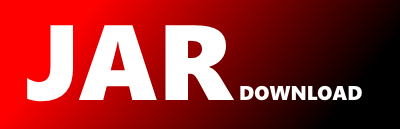
com.pulumi.azurenative.logic.outputs.AS2SecuritySettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AS2SecuritySettingsResponse {
/**
* @return The value indicating whether to enable NRR for inbound decoded messages.
*
*/
private Boolean enableNRRForInboundDecodedMessages;
/**
* @return The value indicating whether to enable NRR for inbound encoded messages.
*
*/
private Boolean enableNRRForInboundEncodedMessages;
/**
* @return The value indicating whether to enable NRR for inbound MDN.
*
*/
private Boolean enableNRRForInboundMDN;
/**
* @return The value indicating whether to enable NRR for outbound decoded messages.
*
*/
private Boolean enableNRRForOutboundDecodedMessages;
/**
* @return The value indicating whether to enable NRR for outbound encoded messages.
*
*/
private Boolean enableNRRForOutboundEncodedMessages;
/**
* @return The value indicating whether to enable NRR for outbound MDN.
*
*/
private Boolean enableNRRForOutboundMDN;
/**
* @return The name of the encryption certificate.
*
*/
private @Nullable String encryptionCertificateName;
/**
* @return The value indicating whether to send or request a MDN.
*
*/
private Boolean overrideGroupSigningCertificate;
/**
* @return The Sha2 algorithm format. Valid values are Sha2, ShaHashSize, ShaHyphenHashSize, Sha2UnderscoreHashSize.
*
*/
private @Nullable String sha2AlgorithmFormat;
/**
* @return The name of the signing certificate.
*
*/
private @Nullable String signingCertificateName;
private AS2SecuritySettingsResponse() {}
/**
* @return The value indicating whether to enable NRR for inbound decoded messages.
*
*/
public Boolean enableNRRForInboundDecodedMessages() {
return this.enableNRRForInboundDecodedMessages;
}
/**
* @return The value indicating whether to enable NRR for inbound encoded messages.
*
*/
public Boolean enableNRRForInboundEncodedMessages() {
return this.enableNRRForInboundEncodedMessages;
}
/**
* @return The value indicating whether to enable NRR for inbound MDN.
*
*/
public Boolean enableNRRForInboundMDN() {
return this.enableNRRForInboundMDN;
}
/**
* @return The value indicating whether to enable NRR for outbound decoded messages.
*
*/
public Boolean enableNRRForOutboundDecodedMessages() {
return this.enableNRRForOutboundDecodedMessages;
}
/**
* @return The value indicating whether to enable NRR for outbound encoded messages.
*
*/
public Boolean enableNRRForOutboundEncodedMessages() {
return this.enableNRRForOutboundEncodedMessages;
}
/**
* @return The value indicating whether to enable NRR for outbound MDN.
*
*/
public Boolean enableNRRForOutboundMDN() {
return this.enableNRRForOutboundMDN;
}
/**
* @return The name of the encryption certificate.
*
*/
public Optional encryptionCertificateName() {
return Optional.ofNullable(this.encryptionCertificateName);
}
/**
* @return The value indicating whether to send or request a MDN.
*
*/
public Boolean overrideGroupSigningCertificate() {
return this.overrideGroupSigningCertificate;
}
/**
* @return The Sha2 algorithm format. Valid values are Sha2, ShaHashSize, ShaHyphenHashSize, Sha2UnderscoreHashSize.
*
*/
public Optional sha2AlgorithmFormat() {
return Optional.ofNullable(this.sha2AlgorithmFormat);
}
/**
* @return The name of the signing certificate.
*
*/
public Optional signingCertificateName() {
return Optional.ofNullable(this.signingCertificateName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AS2SecuritySettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean enableNRRForInboundDecodedMessages;
private Boolean enableNRRForInboundEncodedMessages;
private Boolean enableNRRForInboundMDN;
private Boolean enableNRRForOutboundDecodedMessages;
private Boolean enableNRRForOutboundEncodedMessages;
private Boolean enableNRRForOutboundMDN;
private @Nullable String encryptionCertificateName;
private Boolean overrideGroupSigningCertificate;
private @Nullable String sha2AlgorithmFormat;
private @Nullable String signingCertificateName;
public Builder() {}
public Builder(AS2SecuritySettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.enableNRRForInboundDecodedMessages = defaults.enableNRRForInboundDecodedMessages;
this.enableNRRForInboundEncodedMessages = defaults.enableNRRForInboundEncodedMessages;
this.enableNRRForInboundMDN = defaults.enableNRRForInboundMDN;
this.enableNRRForOutboundDecodedMessages = defaults.enableNRRForOutboundDecodedMessages;
this.enableNRRForOutboundEncodedMessages = defaults.enableNRRForOutboundEncodedMessages;
this.enableNRRForOutboundMDN = defaults.enableNRRForOutboundMDN;
this.encryptionCertificateName = defaults.encryptionCertificateName;
this.overrideGroupSigningCertificate = defaults.overrideGroupSigningCertificate;
this.sha2AlgorithmFormat = defaults.sha2AlgorithmFormat;
this.signingCertificateName = defaults.signingCertificateName;
}
@CustomType.Setter
public Builder enableNRRForInboundDecodedMessages(Boolean enableNRRForInboundDecodedMessages) {
if (enableNRRForInboundDecodedMessages == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "enableNRRForInboundDecodedMessages");
}
this.enableNRRForInboundDecodedMessages = enableNRRForInboundDecodedMessages;
return this;
}
@CustomType.Setter
public Builder enableNRRForInboundEncodedMessages(Boolean enableNRRForInboundEncodedMessages) {
if (enableNRRForInboundEncodedMessages == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "enableNRRForInboundEncodedMessages");
}
this.enableNRRForInboundEncodedMessages = enableNRRForInboundEncodedMessages;
return this;
}
@CustomType.Setter
public Builder enableNRRForInboundMDN(Boolean enableNRRForInboundMDN) {
if (enableNRRForInboundMDN == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "enableNRRForInboundMDN");
}
this.enableNRRForInboundMDN = enableNRRForInboundMDN;
return this;
}
@CustomType.Setter
public Builder enableNRRForOutboundDecodedMessages(Boolean enableNRRForOutboundDecodedMessages) {
if (enableNRRForOutboundDecodedMessages == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "enableNRRForOutboundDecodedMessages");
}
this.enableNRRForOutboundDecodedMessages = enableNRRForOutboundDecodedMessages;
return this;
}
@CustomType.Setter
public Builder enableNRRForOutboundEncodedMessages(Boolean enableNRRForOutboundEncodedMessages) {
if (enableNRRForOutboundEncodedMessages == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "enableNRRForOutboundEncodedMessages");
}
this.enableNRRForOutboundEncodedMessages = enableNRRForOutboundEncodedMessages;
return this;
}
@CustomType.Setter
public Builder enableNRRForOutboundMDN(Boolean enableNRRForOutboundMDN) {
if (enableNRRForOutboundMDN == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "enableNRRForOutboundMDN");
}
this.enableNRRForOutboundMDN = enableNRRForOutboundMDN;
return this;
}
@CustomType.Setter
public Builder encryptionCertificateName(@Nullable String encryptionCertificateName) {
this.encryptionCertificateName = encryptionCertificateName;
return this;
}
@CustomType.Setter
public Builder overrideGroupSigningCertificate(Boolean overrideGroupSigningCertificate) {
if (overrideGroupSigningCertificate == null) {
throw new MissingRequiredPropertyException("AS2SecuritySettingsResponse", "overrideGroupSigningCertificate");
}
this.overrideGroupSigningCertificate = overrideGroupSigningCertificate;
return this;
}
@CustomType.Setter
public Builder sha2AlgorithmFormat(@Nullable String sha2AlgorithmFormat) {
this.sha2AlgorithmFormat = sha2AlgorithmFormat;
return this;
}
@CustomType.Setter
public Builder signingCertificateName(@Nullable String signingCertificateName) {
this.signingCertificateName = signingCertificateName;
return this;
}
public AS2SecuritySettingsResponse build() {
final var _resultValue = new AS2SecuritySettingsResponse();
_resultValue.enableNRRForInboundDecodedMessages = enableNRRForInboundDecodedMessages;
_resultValue.enableNRRForInboundEncodedMessages = enableNRRForInboundEncodedMessages;
_resultValue.enableNRRForInboundMDN = enableNRRForInboundMDN;
_resultValue.enableNRRForOutboundDecodedMessages = enableNRRForOutboundDecodedMessages;
_resultValue.enableNRRForOutboundEncodedMessages = enableNRRForOutboundEncodedMessages;
_resultValue.enableNRRForOutboundMDN = enableNRRForOutboundMDN;
_resultValue.encryptionCertificateName = encryptionCertificateName;
_resultValue.overrideGroupSigningCertificate = overrideGroupSigningCertificate;
_resultValue.sha2AlgorithmFormat = sha2AlgorithmFormat;
_resultValue.signingCertificateName = signingCertificateName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy