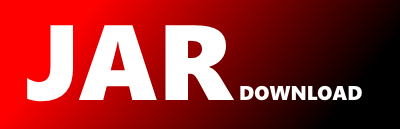
com.pulumi.azurenative.logic.outputs.GetRosettaNetProcessConfigurationResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.outputs;
import com.pulumi.azurenative.logic.outputs.RosettaNetPipActivitySettingsResponse;
import com.pulumi.azurenative.logic.outputs.RosettaNetPipRoleSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetRosettaNetProcessConfigurationResult {
/**
* @return The RosettaNet process configuration activity settings.
*
*/
private RosettaNetPipActivitySettingsResponse activitySettings;
/**
* @return The changed time.
*
*/
private String changedTime;
/**
* @return The created time.
*
*/
private String createdTime;
/**
* @return The integration account RosettaNet ProcessConfiguration properties.
*
*/
private @Nullable String description;
/**
* @return The resource id.
*
*/
private String id;
/**
* @return The RosettaNet initiator role settings.
*
*/
private RosettaNetPipRoleSettingsResponse initiatorRoleSettings;
/**
* @return The resource location.
*
*/
private @Nullable String location;
/**
* @return The metadata.
*
*/
private @Nullable Map metadata;
/**
* @return Gets the resource name.
*
*/
private String name;
/**
* @return The integration account RosettaNet process code.
*
*/
private String processCode;
/**
* @return The integration account RosettaNet process name.
*
*/
private String processName;
/**
* @return The integration account RosettaNet process version.
*
*/
private String processVersion;
/**
* @return The RosettaNet responder role settings.
*
*/
private RosettaNetPipRoleSettingsResponse responderRoleSettings;
/**
* @return The resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Gets the resource type.
*
*/
private String type;
private GetRosettaNetProcessConfigurationResult() {}
/**
* @return The RosettaNet process configuration activity settings.
*
*/
public RosettaNetPipActivitySettingsResponse activitySettings() {
return this.activitySettings;
}
/**
* @return The changed time.
*
*/
public String changedTime() {
return this.changedTime;
}
/**
* @return The created time.
*
*/
public String createdTime() {
return this.createdTime;
}
/**
* @return The integration account RosettaNet ProcessConfiguration properties.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The resource id.
*
*/
public String id() {
return this.id;
}
/**
* @return The RosettaNet initiator role settings.
*
*/
public RosettaNetPipRoleSettingsResponse initiatorRoleSettings() {
return this.initiatorRoleSettings;
}
/**
* @return The resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The metadata.
*
*/
public Map metadata() {
return this.metadata == null ? Map.of() : this.metadata;
}
/**
* @return Gets the resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The integration account RosettaNet process code.
*
*/
public String processCode() {
return this.processCode;
}
/**
* @return The integration account RosettaNet process name.
*
*/
public String processName() {
return this.processName;
}
/**
* @return The integration account RosettaNet process version.
*
*/
public String processVersion() {
return this.processVersion;
}
/**
* @return The RosettaNet responder role settings.
*
*/
public RosettaNetPipRoleSettingsResponse responderRoleSettings() {
return this.responderRoleSettings;
}
/**
* @return The resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Gets the resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRosettaNetProcessConfigurationResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private RosettaNetPipActivitySettingsResponse activitySettings;
private String changedTime;
private String createdTime;
private @Nullable String description;
private String id;
private RosettaNetPipRoleSettingsResponse initiatorRoleSettings;
private @Nullable String location;
private @Nullable Map metadata;
private String name;
private String processCode;
private String processName;
private String processVersion;
private RosettaNetPipRoleSettingsResponse responderRoleSettings;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetRosettaNetProcessConfigurationResult defaults) {
Objects.requireNonNull(defaults);
this.activitySettings = defaults.activitySettings;
this.changedTime = defaults.changedTime;
this.createdTime = defaults.createdTime;
this.description = defaults.description;
this.id = defaults.id;
this.initiatorRoleSettings = defaults.initiatorRoleSettings;
this.location = defaults.location;
this.metadata = defaults.metadata;
this.name = defaults.name;
this.processCode = defaults.processCode;
this.processName = defaults.processName;
this.processVersion = defaults.processVersion;
this.responderRoleSettings = defaults.responderRoleSettings;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder activitySettings(RosettaNetPipActivitySettingsResponse activitySettings) {
if (activitySettings == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "activitySettings");
}
this.activitySettings = activitySettings;
return this;
}
@CustomType.Setter
public Builder changedTime(String changedTime) {
if (changedTime == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "changedTime");
}
this.changedTime = changedTime;
return this;
}
@CustomType.Setter
public Builder createdTime(String createdTime) {
if (createdTime == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "createdTime");
}
this.createdTime = createdTime;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder initiatorRoleSettings(RosettaNetPipRoleSettingsResponse initiatorRoleSettings) {
if (initiatorRoleSettings == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "initiatorRoleSettings");
}
this.initiatorRoleSettings = initiatorRoleSettings;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder metadata(@Nullable Map metadata) {
this.metadata = metadata;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder processCode(String processCode) {
if (processCode == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "processCode");
}
this.processCode = processCode;
return this;
}
@CustomType.Setter
public Builder processName(String processName) {
if (processName == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "processName");
}
this.processName = processName;
return this;
}
@CustomType.Setter
public Builder processVersion(String processVersion) {
if (processVersion == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "processVersion");
}
this.processVersion = processVersion;
return this;
}
@CustomType.Setter
public Builder responderRoleSettings(RosettaNetPipRoleSettingsResponse responderRoleSettings) {
if (responderRoleSettings == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "responderRoleSettings");
}
this.responderRoleSettings = responderRoleSettings;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetRosettaNetProcessConfigurationResult", "type");
}
this.type = type;
return this;
}
public GetRosettaNetProcessConfigurationResult build() {
final var _resultValue = new GetRosettaNetProcessConfigurationResult();
_resultValue.activitySettings = activitySettings;
_resultValue.changedTime = changedTime;
_resultValue.createdTime = createdTime;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.initiatorRoleSettings = initiatorRoleSettings;
_resultValue.location = location;
_resultValue.metadata = metadata;
_resultValue.name = name;
_resultValue.processCode = processCode;
_resultValue.processName = processName;
_resultValue.processVersion = processVersion;
_resultValue.responderRoleSettings = responderRoleSettings;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy