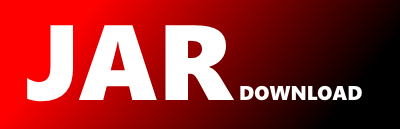
com.pulumi.azurenative.logic.outputs.RosettaNetPipActivityBehaviorResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class RosettaNetPipActivityBehaviorResponse {
/**
* @return The value indicating whether the RosettaNet PIP is used for a single action.
*
*/
private String actionType;
/**
* @return The value indicating whether authorization is required.
*
*/
private Boolean isAuthorizationRequired;
/**
* @return The value indicating whether secured transport is required.
*
*/
private Boolean isSecuredTransportRequired;
/**
* @return The value indicating whether non-repudiation is for origin and content.
*
*/
private Boolean nonRepudiationOfOriginAndContent;
/**
* @return The persistent confidentiality encryption scope.
*
*/
private String persistentConfidentialityScope;
/**
* @return The value indicating whether the RosettaNet PIP communication is synchronous.
*
*/
private String responseType;
/**
* @return The value indicating retry count.
*
*/
private Integer retryCount;
/**
* @return The time to perform in seconds.
*
*/
private Integer timeToPerformInSeconds;
private RosettaNetPipActivityBehaviorResponse() {}
/**
* @return The value indicating whether the RosettaNet PIP is used for a single action.
*
*/
public String actionType() {
return this.actionType;
}
/**
* @return The value indicating whether authorization is required.
*
*/
public Boolean isAuthorizationRequired() {
return this.isAuthorizationRequired;
}
/**
* @return The value indicating whether secured transport is required.
*
*/
public Boolean isSecuredTransportRequired() {
return this.isSecuredTransportRequired;
}
/**
* @return The value indicating whether non-repudiation is for origin and content.
*
*/
public Boolean nonRepudiationOfOriginAndContent() {
return this.nonRepudiationOfOriginAndContent;
}
/**
* @return The persistent confidentiality encryption scope.
*
*/
public String persistentConfidentialityScope() {
return this.persistentConfidentialityScope;
}
/**
* @return The value indicating whether the RosettaNet PIP communication is synchronous.
*
*/
public String responseType() {
return this.responseType;
}
/**
* @return The value indicating retry count.
*
*/
public Integer retryCount() {
return this.retryCount;
}
/**
* @return The time to perform in seconds.
*
*/
public Integer timeToPerformInSeconds() {
return this.timeToPerformInSeconds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RosettaNetPipActivityBehaviorResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String actionType;
private Boolean isAuthorizationRequired;
private Boolean isSecuredTransportRequired;
private Boolean nonRepudiationOfOriginAndContent;
private String persistentConfidentialityScope;
private String responseType;
private Integer retryCount;
private Integer timeToPerformInSeconds;
public Builder() {}
public Builder(RosettaNetPipActivityBehaviorResponse defaults) {
Objects.requireNonNull(defaults);
this.actionType = defaults.actionType;
this.isAuthorizationRequired = defaults.isAuthorizationRequired;
this.isSecuredTransportRequired = defaults.isSecuredTransportRequired;
this.nonRepudiationOfOriginAndContent = defaults.nonRepudiationOfOriginAndContent;
this.persistentConfidentialityScope = defaults.persistentConfidentialityScope;
this.responseType = defaults.responseType;
this.retryCount = defaults.retryCount;
this.timeToPerformInSeconds = defaults.timeToPerformInSeconds;
}
@CustomType.Setter
public Builder actionType(String actionType) {
if (actionType == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "actionType");
}
this.actionType = actionType;
return this;
}
@CustomType.Setter
public Builder isAuthorizationRequired(Boolean isAuthorizationRequired) {
if (isAuthorizationRequired == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "isAuthorizationRequired");
}
this.isAuthorizationRequired = isAuthorizationRequired;
return this;
}
@CustomType.Setter
public Builder isSecuredTransportRequired(Boolean isSecuredTransportRequired) {
if (isSecuredTransportRequired == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "isSecuredTransportRequired");
}
this.isSecuredTransportRequired = isSecuredTransportRequired;
return this;
}
@CustomType.Setter
public Builder nonRepudiationOfOriginAndContent(Boolean nonRepudiationOfOriginAndContent) {
if (nonRepudiationOfOriginAndContent == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "nonRepudiationOfOriginAndContent");
}
this.nonRepudiationOfOriginAndContent = nonRepudiationOfOriginAndContent;
return this;
}
@CustomType.Setter
public Builder persistentConfidentialityScope(String persistentConfidentialityScope) {
if (persistentConfidentialityScope == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "persistentConfidentialityScope");
}
this.persistentConfidentialityScope = persistentConfidentialityScope;
return this;
}
@CustomType.Setter
public Builder responseType(String responseType) {
if (responseType == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "responseType");
}
this.responseType = responseType;
return this;
}
@CustomType.Setter
public Builder retryCount(Integer retryCount) {
if (retryCount == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "retryCount");
}
this.retryCount = retryCount;
return this;
}
@CustomType.Setter
public Builder timeToPerformInSeconds(Integer timeToPerformInSeconds) {
if (timeToPerformInSeconds == null) {
throw new MissingRequiredPropertyException("RosettaNetPipActivityBehaviorResponse", "timeToPerformInSeconds");
}
this.timeToPerformInSeconds = timeToPerformInSeconds;
return this;
}
public RosettaNetPipActivityBehaviorResponse build() {
final var _resultValue = new RosettaNetPipActivityBehaviorResponse();
_resultValue.actionType = actionType;
_resultValue.isAuthorizationRequired = isAuthorizationRequired;
_resultValue.isSecuredTransportRequired = isSecuredTransportRequired;
_resultValue.nonRepudiationOfOriginAndContent = nonRepudiationOfOriginAndContent;
_resultValue.persistentConfidentialityScope = persistentConfidentialityScope;
_resultValue.responseType = responseType;
_resultValue.retryCount = retryCount;
_resultValue.timeToPerformInSeconds = timeToPerformInSeconds;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy