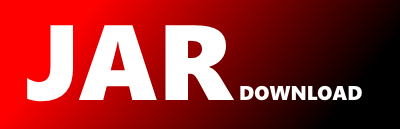
com.pulumi.azurenative.logic.outputs.X12EnvelopeOverrideResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.logic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class X12EnvelopeOverrideResponse {
/**
* @return The date format.
*
*/
private String dateFormat;
/**
* @return The functional identifier code.
*
*/
private @Nullable String functionalIdentifierCode;
/**
* @return The header version.
*
*/
private String headerVersion;
/**
* @return The message id on which this envelope settings has to be applied.
*
*/
private String messageId;
/**
* @return The protocol version on which this envelope settings has to be applied.
*
*/
private String protocolVersion;
/**
* @return The receiver application id.
*
*/
private String receiverApplicationId;
/**
* @return The responsible agency code.
*
*/
private String responsibleAgencyCode;
/**
* @return The sender application id.
*
*/
private String senderApplicationId;
/**
* @return The target namespace on which this envelope settings has to be applied.
*
*/
private String targetNamespace;
/**
* @return The time format.
*
*/
private String timeFormat;
private X12EnvelopeOverrideResponse() {}
/**
* @return The date format.
*
*/
public String dateFormat() {
return this.dateFormat;
}
/**
* @return The functional identifier code.
*
*/
public Optional functionalIdentifierCode() {
return Optional.ofNullable(this.functionalIdentifierCode);
}
/**
* @return The header version.
*
*/
public String headerVersion() {
return this.headerVersion;
}
/**
* @return The message id on which this envelope settings has to be applied.
*
*/
public String messageId() {
return this.messageId;
}
/**
* @return The protocol version on which this envelope settings has to be applied.
*
*/
public String protocolVersion() {
return this.protocolVersion;
}
/**
* @return The receiver application id.
*
*/
public String receiverApplicationId() {
return this.receiverApplicationId;
}
/**
* @return The responsible agency code.
*
*/
public String responsibleAgencyCode() {
return this.responsibleAgencyCode;
}
/**
* @return The sender application id.
*
*/
public String senderApplicationId() {
return this.senderApplicationId;
}
/**
* @return The target namespace on which this envelope settings has to be applied.
*
*/
public String targetNamespace() {
return this.targetNamespace;
}
/**
* @return The time format.
*
*/
public String timeFormat() {
return this.timeFormat;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(X12EnvelopeOverrideResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String dateFormat;
private @Nullable String functionalIdentifierCode;
private String headerVersion;
private String messageId;
private String protocolVersion;
private String receiverApplicationId;
private String responsibleAgencyCode;
private String senderApplicationId;
private String targetNamespace;
private String timeFormat;
public Builder() {}
public Builder(X12EnvelopeOverrideResponse defaults) {
Objects.requireNonNull(defaults);
this.dateFormat = defaults.dateFormat;
this.functionalIdentifierCode = defaults.functionalIdentifierCode;
this.headerVersion = defaults.headerVersion;
this.messageId = defaults.messageId;
this.protocolVersion = defaults.protocolVersion;
this.receiverApplicationId = defaults.receiverApplicationId;
this.responsibleAgencyCode = defaults.responsibleAgencyCode;
this.senderApplicationId = defaults.senderApplicationId;
this.targetNamespace = defaults.targetNamespace;
this.timeFormat = defaults.timeFormat;
}
@CustomType.Setter
public Builder dateFormat(String dateFormat) {
if (dateFormat == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "dateFormat");
}
this.dateFormat = dateFormat;
return this;
}
@CustomType.Setter
public Builder functionalIdentifierCode(@Nullable String functionalIdentifierCode) {
this.functionalIdentifierCode = functionalIdentifierCode;
return this;
}
@CustomType.Setter
public Builder headerVersion(String headerVersion) {
if (headerVersion == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "headerVersion");
}
this.headerVersion = headerVersion;
return this;
}
@CustomType.Setter
public Builder messageId(String messageId) {
if (messageId == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "messageId");
}
this.messageId = messageId;
return this;
}
@CustomType.Setter
public Builder protocolVersion(String protocolVersion) {
if (protocolVersion == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "protocolVersion");
}
this.protocolVersion = protocolVersion;
return this;
}
@CustomType.Setter
public Builder receiverApplicationId(String receiverApplicationId) {
if (receiverApplicationId == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "receiverApplicationId");
}
this.receiverApplicationId = receiverApplicationId;
return this;
}
@CustomType.Setter
public Builder responsibleAgencyCode(String responsibleAgencyCode) {
if (responsibleAgencyCode == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "responsibleAgencyCode");
}
this.responsibleAgencyCode = responsibleAgencyCode;
return this;
}
@CustomType.Setter
public Builder senderApplicationId(String senderApplicationId) {
if (senderApplicationId == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "senderApplicationId");
}
this.senderApplicationId = senderApplicationId;
return this;
}
@CustomType.Setter
public Builder targetNamespace(String targetNamespace) {
if (targetNamespace == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "targetNamespace");
}
this.targetNamespace = targetNamespace;
return this;
}
@CustomType.Setter
public Builder timeFormat(String timeFormat) {
if (timeFormat == null) {
throw new MissingRequiredPropertyException("X12EnvelopeOverrideResponse", "timeFormat");
}
this.timeFormat = timeFormat;
return this;
}
public X12EnvelopeOverrideResponse build() {
final var _resultValue = new X12EnvelopeOverrideResponse();
_resultValue.dateFormat = dateFormat;
_resultValue.functionalIdentifierCode = functionalIdentifierCode;
_resultValue.headerVersion = headerVersion;
_resultValue.messageId = messageId;
_resultValue.protocolVersion = protocolVersion;
_resultValue.receiverApplicationId = receiverApplicationId;
_resultValue.responsibleAgencyCode = responsibleAgencyCode;
_resultValue.senderApplicationId = senderApplicationId;
_resultValue.targetNamespace = targetNamespace;
_resultValue.timeFormat = timeFormat;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy