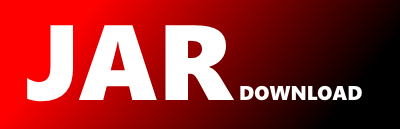
com.pulumi.azurenative.machinelearning.outputs.WebServicePropertiesForGraphResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearning.outputs;
import com.pulumi.azurenative.machinelearning.outputs.AssetItemResponse;
import com.pulumi.azurenative.machinelearning.outputs.BlobLocationResponse;
import com.pulumi.azurenative.machinelearning.outputs.CommitmentPlanResponse;
import com.pulumi.azurenative.machinelearning.outputs.DiagnosticsConfigurationResponse;
import com.pulumi.azurenative.machinelearning.outputs.ExampleRequestResponse;
import com.pulumi.azurenative.machinelearning.outputs.GraphPackageResponse;
import com.pulumi.azurenative.machinelearning.outputs.MachineLearningWorkspaceResponse;
import com.pulumi.azurenative.machinelearning.outputs.RealtimeConfigurationResponse;
import com.pulumi.azurenative.machinelearning.outputs.ServiceInputOutputSpecificationResponse;
import com.pulumi.azurenative.machinelearning.outputs.StorageAccountResponse;
import com.pulumi.azurenative.machinelearning.outputs.WebServiceKeysResponse;
import com.pulumi.azurenative.machinelearning.outputs.WebServiceParameterResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class WebServicePropertiesForGraphResponse {
/**
* @return Contains user defined properties describing web service assets. Properties are expressed as Key/Value pairs.
*
*/
private @Nullable Map assets;
/**
* @return Contains the commitment plan associated with this web service. Set at creation time. Once set, this value cannot be changed. Note: The commitment plan is not returned from calls to GET operations.
*
*/
private @Nullable CommitmentPlanResponse commitmentPlan;
/**
* @return Read Only: The date and time when the web service was created.
*
*/
private String createdOn;
/**
* @return The description of the web service.
*
*/
private @Nullable String description;
/**
* @return Settings controlling the diagnostics traces collection for the web service.
*
*/
private @Nullable DiagnosticsConfigurationResponse diagnostics;
/**
* @return Defines sample input data for one or more of the service's inputs.
*
*/
private @Nullable ExampleRequestResponse exampleRequest;
/**
* @return When set to true, sample data is included in the web service's swagger definition. The default value is true.
*
*/
private @Nullable Boolean exposeSampleData;
/**
* @return Contains the Swagger 2.0 schema describing one or more of the web service's inputs. For more information, see the Swagger specification.
*
*/
private @Nullable ServiceInputOutputSpecificationResponse input;
/**
* @return Contains the web service provisioning keys. If you do not specify provisioning keys, the Azure Machine Learning system generates them for you. Note: The keys are not returned from calls to GET operations.
*
*/
private @Nullable WebServiceKeysResponse keys;
/**
* @return Specifies the Machine Learning workspace containing the experiment that is source for the web service.
*
*/
private @Nullable MachineLearningWorkspaceResponse machineLearningWorkspace;
/**
* @return Read Only: The date and time when the web service was last modified.
*
*/
private String modifiedOn;
/**
* @return Contains the Swagger 2.0 schema describing one or more of the web service's outputs. For more information, see the Swagger specification.
*
*/
private @Nullable ServiceInputOutputSpecificationResponse output;
/**
* @return The definition of the graph package making up this web service.
*
*/
private @Nullable GraphPackageResponse package_;
/**
* @return Specifies the package type. Valid values are Graph (Specifies a web service published through the Machine Learning Studio) and Code (Specifies a web service published using code such as Python). Note: Code is not supported at this time.
* Expected value is 'Graph'.
*
*/
private String packageType;
/**
* @return The set of global parameters values defined for the web service, given as a global parameter name to default value map. If no default value is specified, the parameter is considered to be required.
*
*/
private @Nullable Map parameters;
/**
* @return When set to true, indicates that the payload size is larger than 3 MB. Otherwise false. If the payload size exceed 3 MB, the payload is stored in a blob and the PayloadsLocation parameter contains the URI of the blob. Otherwise, this will be set to false and Assets, Input, Output, Package, Parameters, ExampleRequest are inline. The Payload sizes is determined by adding the size of the Assets, Input, Output, Package, Parameters, and the ExampleRequest.
*
*/
private @Nullable Boolean payloadsInBlobStorage;
/**
* @return The URI of the payload blob. This parameter contains a value only if the payloadsInBlobStorage parameter is set to true. Otherwise is set to null.
*
*/
private @Nullable BlobLocationResponse payloadsLocation;
/**
* @return Read Only: The provision state of the web service. Valid values are Unknown, Provisioning, Succeeded, and Failed.
*
*/
private String provisioningState;
/**
* @return When set to true, indicates that the web service is read-only and can no longer be updated or patched, only removed. Default, is false. Note: Once set to true, you cannot change its value.
*
*/
private @Nullable Boolean readOnly;
/**
* @return Contains the configuration settings for the web service endpoint.
*
*/
private @Nullable RealtimeConfigurationResponse realtimeConfiguration;
/**
* @return Specifies the storage account that Azure Machine Learning uses to store information about the web service. Only the name of the storage account is returned from calls to GET operations. When updating the storage account information, you must ensure that all necessary assets are available in the new storage account or calls to your web service will fail.
*
*/
private @Nullable StorageAccountResponse storageAccount;
/**
* @return Read Only: Contains the URI of the swagger spec associated with this web service.
*
*/
private String swaggerLocation;
/**
* @return The title of the web service.
*
*/
private @Nullable String title;
private WebServicePropertiesForGraphResponse() {}
/**
* @return Contains user defined properties describing web service assets. Properties are expressed as Key/Value pairs.
*
*/
public Map assets() {
return this.assets == null ? Map.of() : this.assets;
}
/**
* @return Contains the commitment plan associated with this web service. Set at creation time. Once set, this value cannot be changed. Note: The commitment plan is not returned from calls to GET operations.
*
*/
public Optional commitmentPlan() {
return Optional.ofNullable(this.commitmentPlan);
}
/**
* @return Read Only: The date and time when the web service was created.
*
*/
public String createdOn() {
return this.createdOn;
}
/**
* @return The description of the web service.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Settings controlling the diagnostics traces collection for the web service.
*
*/
public Optional diagnostics() {
return Optional.ofNullable(this.diagnostics);
}
/**
* @return Defines sample input data for one or more of the service's inputs.
*
*/
public Optional exampleRequest() {
return Optional.ofNullable(this.exampleRequest);
}
/**
* @return When set to true, sample data is included in the web service's swagger definition. The default value is true.
*
*/
public Optional exposeSampleData() {
return Optional.ofNullable(this.exposeSampleData);
}
/**
* @return Contains the Swagger 2.0 schema describing one or more of the web service's inputs. For more information, see the Swagger specification.
*
*/
public Optional input() {
return Optional.ofNullable(this.input);
}
/**
* @return Contains the web service provisioning keys. If you do not specify provisioning keys, the Azure Machine Learning system generates them for you. Note: The keys are not returned from calls to GET operations.
*
*/
public Optional keys() {
return Optional.ofNullable(this.keys);
}
/**
* @return Specifies the Machine Learning workspace containing the experiment that is source for the web service.
*
*/
public Optional machineLearningWorkspace() {
return Optional.ofNullable(this.machineLearningWorkspace);
}
/**
* @return Read Only: The date and time when the web service was last modified.
*
*/
public String modifiedOn() {
return this.modifiedOn;
}
/**
* @return Contains the Swagger 2.0 schema describing one or more of the web service's outputs. For more information, see the Swagger specification.
*
*/
public Optional output() {
return Optional.ofNullable(this.output);
}
/**
* @return The definition of the graph package making up this web service.
*
*/
public Optional package_() {
return Optional.ofNullable(this.package_);
}
/**
* @return Specifies the package type. Valid values are Graph (Specifies a web service published through the Machine Learning Studio) and Code (Specifies a web service published using code such as Python). Note: Code is not supported at this time.
* Expected value is 'Graph'.
*
*/
public String packageType() {
return this.packageType;
}
/**
* @return The set of global parameters values defined for the web service, given as a global parameter name to default value map. If no default value is specified, the parameter is considered to be required.
*
*/
public Map parameters() {
return this.parameters == null ? Map.of() : this.parameters;
}
/**
* @return When set to true, indicates that the payload size is larger than 3 MB. Otherwise false. If the payload size exceed 3 MB, the payload is stored in a blob and the PayloadsLocation parameter contains the URI of the blob. Otherwise, this will be set to false and Assets, Input, Output, Package, Parameters, ExampleRequest are inline. The Payload sizes is determined by adding the size of the Assets, Input, Output, Package, Parameters, and the ExampleRequest.
*
*/
public Optional payloadsInBlobStorage() {
return Optional.ofNullable(this.payloadsInBlobStorage);
}
/**
* @return The URI of the payload blob. This parameter contains a value only if the payloadsInBlobStorage parameter is set to true. Otherwise is set to null.
*
*/
public Optional payloadsLocation() {
return Optional.ofNullable(this.payloadsLocation);
}
/**
* @return Read Only: The provision state of the web service. Valid values are Unknown, Provisioning, Succeeded, and Failed.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return When set to true, indicates that the web service is read-only and can no longer be updated or patched, only removed. Default, is false. Note: Once set to true, you cannot change its value.
*
*/
public Optional readOnly() {
return Optional.ofNullable(this.readOnly);
}
/**
* @return Contains the configuration settings for the web service endpoint.
*
*/
public Optional realtimeConfiguration() {
return Optional.ofNullable(this.realtimeConfiguration);
}
/**
* @return Specifies the storage account that Azure Machine Learning uses to store information about the web service. Only the name of the storage account is returned from calls to GET operations. When updating the storage account information, you must ensure that all necessary assets are available in the new storage account or calls to your web service will fail.
*
*/
public Optional storageAccount() {
return Optional.ofNullable(this.storageAccount);
}
/**
* @return Read Only: Contains the URI of the swagger spec associated with this web service.
*
*/
public String swaggerLocation() {
return this.swaggerLocation;
}
/**
* @return The title of the web service.
*
*/
public Optional title() {
return Optional.ofNullable(this.title);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WebServicePropertiesForGraphResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Map assets;
private @Nullable CommitmentPlanResponse commitmentPlan;
private String createdOn;
private @Nullable String description;
private @Nullable DiagnosticsConfigurationResponse diagnostics;
private @Nullable ExampleRequestResponse exampleRequest;
private @Nullable Boolean exposeSampleData;
private @Nullable ServiceInputOutputSpecificationResponse input;
private @Nullable WebServiceKeysResponse keys;
private @Nullable MachineLearningWorkspaceResponse machineLearningWorkspace;
private String modifiedOn;
private @Nullable ServiceInputOutputSpecificationResponse output;
private @Nullable GraphPackageResponse package_;
private String packageType;
private @Nullable Map parameters;
private @Nullable Boolean payloadsInBlobStorage;
private @Nullable BlobLocationResponse payloadsLocation;
private String provisioningState;
private @Nullable Boolean readOnly;
private @Nullable RealtimeConfigurationResponse realtimeConfiguration;
private @Nullable StorageAccountResponse storageAccount;
private String swaggerLocation;
private @Nullable String title;
public Builder() {}
public Builder(WebServicePropertiesForGraphResponse defaults) {
Objects.requireNonNull(defaults);
this.assets = defaults.assets;
this.commitmentPlan = defaults.commitmentPlan;
this.createdOn = defaults.createdOn;
this.description = defaults.description;
this.diagnostics = defaults.diagnostics;
this.exampleRequest = defaults.exampleRequest;
this.exposeSampleData = defaults.exposeSampleData;
this.input = defaults.input;
this.keys = defaults.keys;
this.machineLearningWorkspace = defaults.machineLearningWorkspace;
this.modifiedOn = defaults.modifiedOn;
this.output = defaults.output;
this.package_ = defaults.package_;
this.packageType = defaults.packageType;
this.parameters = defaults.parameters;
this.payloadsInBlobStorage = defaults.payloadsInBlobStorage;
this.payloadsLocation = defaults.payloadsLocation;
this.provisioningState = defaults.provisioningState;
this.readOnly = defaults.readOnly;
this.realtimeConfiguration = defaults.realtimeConfiguration;
this.storageAccount = defaults.storageAccount;
this.swaggerLocation = defaults.swaggerLocation;
this.title = defaults.title;
}
@CustomType.Setter
public Builder assets(@Nullable Map assets) {
this.assets = assets;
return this;
}
@CustomType.Setter
public Builder commitmentPlan(@Nullable CommitmentPlanResponse commitmentPlan) {
this.commitmentPlan = commitmentPlan;
return this;
}
@CustomType.Setter
public Builder createdOn(String createdOn) {
if (createdOn == null) {
throw new MissingRequiredPropertyException("WebServicePropertiesForGraphResponse", "createdOn");
}
this.createdOn = createdOn;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder diagnostics(@Nullable DiagnosticsConfigurationResponse diagnostics) {
this.diagnostics = diagnostics;
return this;
}
@CustomType.Setter
public Builder exampleRequest(@Nullable ExampleRequestResponse exampleRequest) {
this.exampleRequest = exampleRequest;
return this;
}
@CustomType.Setter
public Builder exposeSampleData(@Nullable Boolean exposeSampleData) {
this.exposeSampleData = exposeSampleData;
return this;
}
@CustomType.Setter
public Builder input(@Nullable ServiceInputOutputSpecificationResponse input) {
this.input = input;
return this;
}
@CustomType.Setter
public Builder keys(@Nullable WebServiceKeysResponse keys) {
this.keys = keys;
return this;
}
@CustomType.Setter
public Builder machineLearningWorkspace(@Nullable MachineLearningWorkspaceResponse machineLearningWorkspace) {
this.machineLearningWorkspace = machineLearningWorkspace;
return this;
}
@CustomType.Setter
public Builder modifiedOn(String modifiedOn) {
if (modifiedOn == null) {
throw new MissingRequiredPropertyException("WebServicePropertiesForGraphResponse", "modifiedOn");
}
this.modifiedOn = modifiedOn;
return this;
}
@CustomType.Setter
public Builder output(@Nullable ServiceInputOutputSpecificationResponse output) {
this.output = output;
return this;
}
@CustomType.Setter("package")
public Builder package_(@Nullable GraphPackageResponse package_) {
this.package_ = package_;
return this;
}
@CustomType.Setter
public Builder packageType(String packageType) {
if (packageType == null) {
throw new MissingRequiredPropertyException("WebServicePropertiesForGraphResponse", "packageType");
}
this.packageType = packageType;
return this;
}
@CustomType.Setter
public Builder parameters(@Nullable Map parameters) {
this.parameters = parameters;
return this;
}
@CustomType.Setter
public Builder payloadsInBlobStorage(@Nullable Boolean payloadsInBlobStorage) {
this.payloadsInBlobStorage = payloadsInBlobStorage;
return this;
}
@CustomType.Setter
public Builder payloadsLocation(@Nullable BlobLocationResponse payloadsLocation) {
this.payloadsLocation = payloadsLocation;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("WebServicePropertiesForGraphResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder readOnly(@Nullable Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
@CustomType.Setter
public Builder realtimeConfiguration(@Nullable RealtimeConfigurationResponse realtimeConfiguration) {
this.realtimeConfiguration = realtimeConfiguration;
return this;
}
@CustomType.Setter
public Builder storageAccount(@Nullable StorageAccountResponse storageAccount) {
this.storageAccount = storageAccount;
return this;
}
@CustomType.Setter
public Builder swaggerLocation(String swaggerLocation) {
if (swaggerLocation == null) {
throw new MissingRequiredPropertyException("WebServicePropertiesForGraphResponse", "swaggerLocation");
}
this.swaggerLocation = swaggerLocation;
return this;
}
@CustomType.Setter
public Builder title(@Nullable String title) {
this.title = title;
return this;
}
public WebServicePropertiesForGraphResponse build() {
final var _resultValue = new WebServicePropertiesForGraphResponse();
_resultValue.assets = assets;
_resultValue.commitmentPlan = commitmentPlan;
_resultValue.createdOn = createdOn;
_resultValue.description = description;
_resultValue.diagnostics = diagnostics;
_resultValue.exampleRequest = exampleRequest;
_resultValue.exposeSampleData = exposeSampleData;
_resultValue.input = input;
_resultValue.keys = keys;
_resultValue.machineLearningWorkspace = machineLearningWorkspace;
_resultValue.modifiedOn = modifiedOn;
_resultValue.output = output;
_resultValue.package_ = package_;
_resultValue.packageType = packageType;
_resultValue.parameters = parameters;
_resultValue.payloadsInBlobStorage = payloadsInBlobStorage;
_resultValue.payloadsLocation = payloadsLocation;
_resultValue.provisioningState = provisioningState;
_resultValue.readOnly = readOnly;
_resultValue.realtimeConfiguration = realtimeConfiguration;
_resultValue.storageAccount = storageAccount;
_resultValue.swaggerLocation = swaggerLocation;
_resultValue.title = title;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy