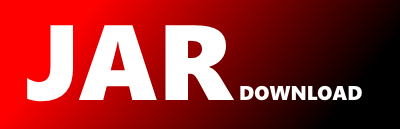
com.pulumi.azurenative.machinelearningservices.outputs.AzureDataLakeSectionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureDataLakeSectionResponse {
/**
* @return The authority URL used for authentication.
*
*/
private @Nullable String authorityUrl;
/**
* @return The content of the certificate used for authentication.
*
*/
private @Nullable String certificate;
/**
* @return The Client ID/Application ID
*
*/
private @Nullable String clientId;
/**
* @return The client secret.
*
*/
private @Nullable String clientSecret;
/**
* @return The Azure Data Lake credential type.
*
*/
private @Nullable String credentialType;
/**
* @return Is it using certificate to authenticate. If false then use client secret.
*
*/
private @Nullable Boolean isCertAuth;
/**
* @return Resource Group.
*
*/
private @Nullable String resourceGroup;
/**
* @return The resource the service principal/app has access to.
*
*/
private @Nullable String resourceUri;
/**
* @return Indicates which identity to use to authenticate service data access to customer's storage.
*
*/
private @Nullable String serviceDataAccessAuthIdentity;
/**
* @return The Azure Data Lake store name.
*
*/
private @Nullable String storeName;
/**
* @return Subscription ID.
*
*/
private @Nullable String subscriptionId;
/**
* @return The ID of the tenant the service principal/app belongs to.
*
*/
private @Nullable String tenantId;
/**
* @return The thumbprint of the certificate above.
*
*/
private @Nullable String thumbprint;
private AzureDataLakeSectionResponse() {}
/**
* @return The authority URL used for authentication.
*
*/
public Optional authorityUrl() {
return Optional.ofNullable(this.authorityUrl);
}
/**
* @return The content of the certificate used for authentication.
*
*/
public Optional certificate() {
return Optional.ofNullable(this.certificate);
}
/**
* @return The Client ID/Application ID
*
*/
public Optional clientId() {
return Optional.ofNullable(this.clientId);
}
/**
* @return The client secret.
*
*/
public Optional clientSecret() {
return Optional.ofNullable(this.clientSecret);
}
/**
* @return The Azure Data Lake credential type.
*
*/
public Optional credentialType() {
return Optional.ofNullable(this.credentialType);
}
/**
* @return Is it using certificate to authenticate. If false then use client secret.
*
*/
public Optional isCertAuth() {
return Optional.ofNullable(this.isCertAuth);
}
/**
* @return Resource Group.
*
*/
public Optional resourceGroup() {
return Optional.ofNullable(this.resourceGroup);
}
/**
* @return The resource the service principal/app has access to.
*
*/
public Optional resourceUri() {
return Optional.ofNullable(this.resourceUri);
}
/**
* @return Indicates which identity to use to authenticate service data access to customer's storage.
*
*/
public Optional serviceDataAccessAuthIdentity() {
return Optional.ofNullable(this.serviceDataAccessAuthIdentity);
}
/**
* @return The Azure Data Lake store name.
*
*/
public Optional storeName() {
return Optional.ofNullable(this.storeName);
}
/**
* @return Subscription ID.
*
*/
public Optional subscriptionId() {
return Optional.ofNullable(this.subscriptionId);
}
/**
* @return The ID of the tenant the service principal/app belongs to.
*
*/
public Optional tenantId() {
return Optional.ofNullable(this.tenantId);
}
/**
* @return The thumbprint of the certificate above.
*
*/
public Optional thumbprint() {
return Optional.ofNullable(this.thumbprint);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureDataLakeSectionResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String authorityUrl;
private @Nullable String certificate;
private @Nullable String clientId;
private @Nullable String clientSecret;
private @Nullable String credentialType;
private @Nullable Boolean isCertAuth;
private @Nullable String resourceGroup;
private @Nullable String resourceUri;
private @Nullable String serviceDataAccessAuthIdentity;
private @Nullable String storeName;
private @Nullable String subscriptionId;
private @Nullable String tenantId;
private @Nullable String thumbprint;
public Builder() {}
public Builder(AzureDataLakeSectionResponse defaults) {
Objects.requireNonNull(defaults);
this.authorityUrl = defaults.authorityUrl;
this.certificate = defaults.certificate;
this.clientId = defaults.clientId;
this.clientSecret = defaults.clientSecret;
this.credentialType = defaults.credentialType;
this.isCertAuth = defaults.isCertAuth;
this.resourceGroup = defaults.resourceGroup;
this.resourceUri = defaults.resourceUri;
this.serviceDataAccessAuthIdentity = defaults.serviceDataAccessAuthIdentity;
this.storeName = defaults.storeName;
this.subscriptionId = defaults.subscriptionId;
this.tenantId = defaults.tenantId;
this.thumbprint = defaults.thumbprint;
}
@CustomType.Setter
public Builder authorityUrl(@Nullable String authorityUrl) {
this.authorityUrl = authorityUrl;
return this;
}
@CustomType.Setter
public Builder certificate(@Nullable String certificate) {
this.certificate = certificate;
return this;
}
@CustomType.Setter
public Builder clientId(@Nullable String clientId) {
this.clientId = clientId;
return this;
}
@CustomType.Setter
public Builder clientSecret(@Nullable String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
@CustomType.Setter
public Builder credentialType(@Nullable String credentialType) {
this.credentialType = credentialType;
return this;
}
@CustomType.Setter
public Builder isCertAuth(@Nullable Boolean isCertAuth) {
this.isCertAuth = isCertAuth;
return this;
}
@CustomType.Setter
public Builder resourceGroup(@Nullable String resourceGroup) {
this.resourceGroup = resourceGroup;
return this;
}
@CustomType.Setter
public Builder resourceUri(@Nullable String resourceUri) {
this.resourceUri = resourceUri;
return this;
}
@CustomType.Setter
public Builder serviceDataAccessAuthIdentity(@Nullable String serviceDataAccessAuthIdentity) {
this.serviceDataAccessAuthIdentity = serviceDataAccessAuthIdentity;
return this;
}
@CustomType.Setter
public Builder storeName(@Nullable String storeName) {
this.storeName = storeName;
return this;
}
@CustomType.Setter
public Builder subscriptionId(@Nullable String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
@CustomType.Setter
public Builder tenantId(@Nullable String tenantId) {
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder thumbprint(@Nullable String thumbprint) {
this.thumbprint = thumbprint;
return this;
}
public AzureDataLakeSectionResponse build() {
final var _resultValue = new AzureDataLakeSectionResponse();
_resultValue.authorityUrl = authorityUrl;
_resultValue.certificate = certificate;
_resultValue.clientId = clientId;
_resultValue.clientSecret = clientSecret;
_resultValue.credentialType = credentialType;
_resultValue.isCertAuth = isCertAuth;
_resultValue.resourceGroup = resourceGroup;
_resultValue.resourceUri = resourceUri;
_resultValue.serviceDataAccessAuthIdentity = serviceDataAccessAuthIdentity;
_resultValue.storeName = storeName;
_resultValue.subscriptionId = subscriptionId;
_resultValue.tenantId = tenantId;
_resultValue.thumbprint = thumbprint;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy