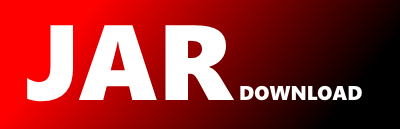
com.pulumi.azurenative.machinelearningservices.outputs.UserInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class UserInfoResponse {
/**
* @return A user alternate sec id. This represents the user in a different identity provider system Eg.1:live.com:puid
*
*/
private @Nullable String userAltSecId;
/**
* @return A user identity provider. Eg live.com
*
*/
private @Nullable String userIdp;
/**
* @return The issuer which issued the token for this user.
*
*/
private @Nullable String userIss;
/**
* @return A user's full name or a service principal's app ID.
*
*/
private @Nullable String userName;
/**
* @return A user or service principal's object ID..
*
*/
private @Nullable String userObjectId;
/**
* @return A user or service principal's PuID.
*
*/
private @Nullable String userPuId;
/**
* @return A user or service principal's tenant ID.
*
*/
private @Nullable String userTenantId;
private UserInfoResponse() {}
/**
* @return A user alternate sec id. This represents the user in a different identity provider system Eg.1:live.com:puid
*
*/
public Optional userAltSecId() {
return Optional.ofNullable(this.userAltSecId);
}
/**
* @return A user identity provider. Eg live.com
*
*/
public Optional userIdp() {
return Optional.ofNullable(this.userIdp);
}
/**
* @return The issuer which issued the token for this user.
*
*/
public Optional userIss() {
return Optional.ofNullable(this.userIss);
}
/**
* @return A user's full name or a service principal's app ID.
*
*/
public Optional userName() {
return Optional.ofNullable(this.userName);
}
/**
* @return A user or service principal's object ID..
*
*/
public Optional userObjectId() {
return Optional.ofNullable(this.userObjectId);
}
/**
* @return A user or service principal's PuID.
*
*/
public Optional userPuId() {
return Optional.ofNullable(this.userPuId);
}
/**
* @return A user or service principal's tenant ID.
*
*/
public Optional userTenantId() {
return Optional.ofNullable(this.userTenantId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(UserInfoResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String userAltSecId;
private @Nullable String userIdp;
private @Nullable String userIss;
private @Nullable String userName;
private @Nullable String userObjectId;
private @Nullable String userPuId;
private @Nullable String userTenantId;
public Builder() {}
public Builder(UserInfoResponse defaults) {
Objects.requireNonNull(defaults);
this.userAltSecId = defaults.userAltSecId;
this.userIdp = defaults.userIdp;
this.userIss = defaults.userIss;
this.userName = defaults.userName;
this.userObjectId = defaults.userObjectId;
this.userPuId = defaults.userPuId;
this.userTenantId = defaults.userTenantId;
}
@CustomType.Setter
public Builder userAltSecId(@Nullable String userAltSecId) {
this.userAltSecId = userAltSecId;
return this;
}
@CustomType.Setter
public Builder userIdp(@Nullable String userIdp) {
this.userIdp = userIdp;
return this;
}
@CustomType.Setter
public Builder userIss(@Nullable String userIss) {
this.userIss = userIss;
return this;
}
@CustomType.Setter
public Builder userName(@Nullable String userName) {
this.userName = userName;
return this;
}
@CustomType.Setter
public Builder userObjectId(@Nullable String userObjectId) {
this.userObjectId = userObjectId;
return this;
}
@CustomType.Setter
public Builder userPuId(@Nullable String userPuId) {
this.userPuId = userPuId;
return this;
}
@CustomType.Setter
public Builder userTenantId(@Nullable String userTenantId) {
this.userTenantId = userTenantId;
return this;
}
public UserInfoResponse build() {
final var _resultValue = new UserInfoResponse();
_resultValue.userAltSecId = userAltSecId;
_resultValue.userIdp = userIdp;
_resultValue.userIss = userIss;
_resultValue.userName = userName;
_resultValue.userObjectId = userObjectId;
_resultValue.userPuId = userPuId;
_resultValue.userTenantId = userTenantId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy