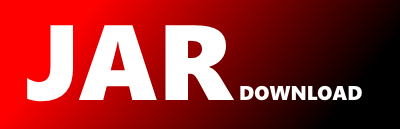
com.pulumi.azurenative.managednetworkfabric.inputs.Layer3ConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managednetworkfabric.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* layer3Configuration
*
*/
public final class Layer3ConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final Layer3ConfigurationArgs Empty = new Layer3ConfigurationArgs();
/**
* exportRoutePolicyId
*
*/
@Import(name="exportRoutePolicyId")
private @Nullable Output exportRoutePolicyId;
/**
* @return exportRoutePolicyId
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy