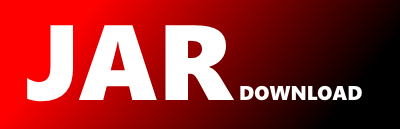
com.pulumi.azurenative.media.inputs.CommonEncryptionCbcsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.inputs.CbcsDrmConfigurationArgs;
import com.pulumi.azurenative.media.inputs.ClearKeyEncryptionConfigurationArgs;
import com.pulumi.azurenative.media.inputs.EnabledProtocolsArgs;
import com.pulumi.azurenative.media.inputs.StreamingPolicyContentKeysArgs;
import com.pulumi.azurenative.media.inputs.TrackSelectionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Class for CommonEncryptionCbcs encryption scheme
*
*/
public final class CommonEncryptionCbcsArgs extends com.pulumi.resources.ResourceArgs {
public static final CommonEncryptionCbcsArgs Empty = new CommonEncryptionCbcsArgs();
/**
* Optional configuration supporting ClearKey in CommonEncryptionCbcs encryption scheme.
*
*/
@Import(name="clearKeyEncryptionConfiguration")
private @Nullable Output clearKeyEncryptionConfiguration;
/**
* @return Optional configuration supporting ClearKey in CommonEncryptionCbcs encryption scheme.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy