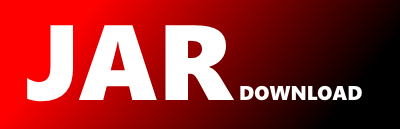
com.pulumi.azurenative.media.inputs.JobInputHttpArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.inputs.AbsoluteClipTimeArgs;
import com.pulumi.azurenative.media.inputs.FromAllInputFileArgs;
import com.pulumi.azurenative.media.inputs.FromEachInputFileArgs;
import com.pulumi.azurenative.media.inputs.InputFileArgs;
import com.pulumi.azurenative.media.inputs.UtcClipTimeArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Represents HTTPS job input.
*
*/
public final class JobInputHttpArgs extends com.pulumi.resources.ResourceArgs {
public static final JobInputHttpArgs Empty = new JobInputHttpArgs();
/**
* Base URI for HTTPS job input. It will be concatenated with provided file names. If no base uri is given, then the provided file list is assumed to be fully qualified uris. Maximum length of 4000 characters. The query strings will not be returned in service responses to prevent sensitive data exposure.
*
*/
@Import(name="baseUri")
private @Nullable Output baseUri;
/**
* @return Base URI for HTTPS job input. It will be concatenated with provided file names. If no base uri is given, then the provided file list is assumed to be fully qualified uris. Maximum length of 4000 characters. The query strings will not be returned in service responses to prevent sensitive data exposure.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy