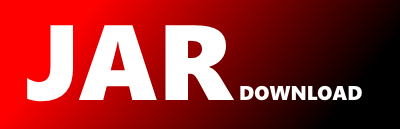
com.pulumi.azurenative.media.inputs.SelectVideoTrackByAttributeArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.AttributeFilter;
import com.pulumi.azurenative.media.enums.TrackAttribute;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Select video tracks from the input by specifying an attribute and an attribute filter.
*
*/
public final class SelectVideoTrackByAttributeArgs extends com.pulumi.resources.ResourceArgs {
public static final SelectVideoTrackByAttributeArgs Empty = new SelectVideoTrackByAttributeArgs();
/**
* The TrackAttribute to filter the tracks by.
*
*/
@Import(name="attribute", required=true)
private Output> attribute;
/**
* @return The TrackAttribute to filter the tracks by.
*
*/
public Output> attribute() {
return this.attribute;
}
/**
* The type of AttributeFilter to apply to the TrackAttribute in order to select the tracks.
*
*/
@Import(name="filter", required=true)
private Output> filter;
/**
* @return The type of AttributeFilter to apply to the TrackAttribute in order to select the tracks.
*
*/
public Output> filter() {
return this.filter;
}
/**
* The value to filter the tracks by. Only used when AttributeFilter.ValueEquals is specified for the Filter property. For TrackAttribute.Bitrate, this should be an integer value in bits per second (e.g: '1500000'). The TrackAttribute.Language is not supported for video tracks.
*
*/
@Import(name="filterValue")
private @Nullable Output filterValue;
/**
* @return The value to filter the tracks by. Only used when AttributeFilter.ValueEquals is specified for the Filter property. For TrackAttribute.Bitrate, this should be an integer value in bits per second (e.g: '1500000'). The TrackAttribute.Language is not supported for video tracks.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy