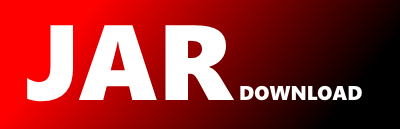
com.pulumi.azurenative.media.outputs.FadeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FadeResponse {
/**
* @return The Duration of the fade effect in the video. The value can be in ISO 8601 format (For example, PT05S to fade In/Out a color during 5 seconds), or a frame count (For example, 10 to fade 10 frames from the start time), or a relative value to stream duration (For example, 10% to fade 10% of stream duration)
*
*/
private String duration;
/**
* @return The Color for the fade In/Out. it can be on the CSS Level1 colors https://developer.mozilla.org/en-US/docs/Web/CSS/color_value/color_keywords or an RGB/hex value: e.g: rgb(255,0,0), 0xFF0000 or #FF0000
*
*/
private String fadeColor;
/**
* @return The position in the input video from where to start fade. The value can be in ISO 8601 format (For example, PT05S to start at 5 seconds), or a frame count (For example, 10 to start at the 10th frame), or a relative value to stream duration (For example, 10% to start at 10% of stream duration). Default is 0
*
*/
private @Nullable String start;
private FadeResponse() {}
/**
* @return The Duration of the fade effect in the video. The value can be in ISO 8601 format (For example, PT05S to fade In/Out a color during 5 seconds), or a frame count (For example, 10 to fade 10 frames from the start time), or a relative value to stream duration (For example, 10% to fade 10% of stream duration)
*
*/
public String duration() {
return this.duration;
}
/**
* @return The Color for the fade In/Out. it can be on the CSS Level1 colors https://developer.mozilla.org/en-US/docs/Web/CSS/color_value/color_keywords or an RGB/hex value: e.g: rgb(255,0,0), 0xFF0000 or #FF0000
*
*/
public String fadeColor() {
return this.fadeColor;
}
/**
* @return The position in the input video from where to start fade. The value can be in ISO 8601 format (For example, PT05S to start at 5 seconds), or a frame count (For example, 10 to start at the 10th frame), or a relative value to stream duration (For example, 10% to start at 10% of stream duration). Default is 0
*
*/
public Optional start() {
return Optional.ofNullable(this.start);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FadeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String duration;
private String fadeColor;
private @Nullable String start;
public Builder() {}
public Builder(FadeResponse defaults) {
Objects.requireNonNull(defaults);
this.duration = defaults.duration;
this.fadeColor = defaults.fadeColor;
this.start = defaults.start;
}
@CustomType.Setter
public Builder duration(String duration) {
if (duration == null) {
throw new MissingRequiredPropertyException("FadeResponse", "duration");
}
this.duration = duration;
return this;
}
@CustomType.Setter
public Builder fadeColor(String fadeColor) {
if (fadeColor == null) {
throw new MissingRequiredPropertyException("FadeResponse", "fadeColor");
}
this.fadeColor = fadeColor;
return this;
}
@CustomType.Setter
public Builder start(@Nullable String start) {
this.start = start;
return this;
}
public FadeResponse build() {
final var _resultValue = new FadeResponse();
_resultValue.duration = duration;
_resultValue.fadeColor = fadeColor;
_resultValue.start = start;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy