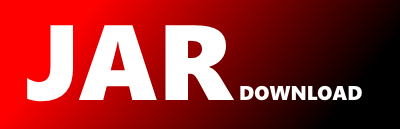
com.pulumi.azurenative.media.outputs.GetLiveOutputResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.HlsResponse;
import com.pulumi.azurenative.media.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLiveOutputResult {
/**
* @return ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*
*/
private String archiveWindowLength;
/**
* @return The asset that the live output will write to.
*
*/
private String assetName;
/**
* @return The creation time the live output.
*
*/
private String created;
/**
* @return The description of the live output.
*
*/
private @Nullable String description;
/**
* @return HTTP Live Streaming (HLS) packing setting for the live output.
*
*/
private @Nullable HlsResponse hls;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The time the live output was last modified.
*
*/
private String lastModified;
/**
* @return The manifest file name. If not provided, the service will generate one automatically.
*
*/
private @Nullable String manifestName;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The initial timestamp that the live output will start at, any content before this value will not be archived.
*
*/
private @Nullable Double outputSnapTime;
/**
* @return The provisioning state of the live output.
*
*/
private String provisioningState;
/**
* @return The resource state of the live output.
*
*/
private String resourceState;
/**
* @return ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*
*/
private @Nullable String rewindWindowLength;
/**
* @return The system metadata relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetLiveOutputResult() {}
/**
* @return ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*
*/
public String archiveWindowLength() {
return this.archiveWindowLength;
}
/**
* @return The asset that the live output will write to.
*
*/
public String assetName() {
return this.assetName;
}
/**
* @return The creation time the live output.
*
*/
public String created() {
return this.created;
}
/**
* @return The description of the live output.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return HTTP Live Streaming (HLS) packing setting for the live output.
*
*/
public Optional hls() {
return Optional.ofNullable(this.hls);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The time the live output was last modified.
*
*/
public String lastModified() {
return this.lastModified;
}
/**
* @return The manifest file name. If not provided, the service will generate one automatically.
*
*/
public Optional manifestName() {
return Optional.ofNullable(this.manifestName);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The initial timestamp that the live output will start at, any content before this value will not be archived.
*
*/
public Optional outputSnapTime() {
return Optional.ofNullable(this.outputSnapTime);
}
/**
* @return The provisioning state of the live output.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The resource state of the live output.
*
*/
public String resourceState() {
return this.resourceState;
}
/**
* @return ISO 8601 time between 1 minute to the duration of archiveWindowLength to control seek-able window length during Live. The service won't use this property once LiveOutput stops. The archived VOD will have full content with original ArchiveWindowLength. For example, use PT1H30M to indicate 1 hour and 30 minutes of rewind window length. Service will use implicit default value 30m only if Live Event enables LL.
*
*/
public Optional rewindWindowLength() {
return Optional.ofNullable(this.rewindWindowLength);
}
/**
* @return The system metadata relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLiveOutputResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String archiveWindowLength;
private String assetName;
private String created;
private @Nullable String description;
private @Nullable HlsResponse hls;
private String id;
private String lastModified;
private @Nullable String manifestName;
private String name;
private @Nullable Double outputSnapTime;
private String provisioningState;
private String resourceState;
private @Nullable String rewindWindowLength;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetLiveOutputResult defaults) {
Objects.requireNonNull(defaults);
this.archiveWindowLength = defaults.archiveWindowLength;
this.assetName = defaults.assetName;
this.created = defaults.created;
this.description = defaults.description;
this.hls = defaults.hls;
this.id = defaults.id;
this.lastModified = defaults.lastModified;
this.manifestName = defaults.manifestName;
this.name = defaults.name;
this.outputSnapTime = defaults.outputSnapTime;
this.provisioningState = defaults.provisioningState;
this.resourceState = defaults.resourceState;
this.rewindWindowLength = defaults.rewindWindowLength;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder archiveWindowLength(String archiveWindowLength) {
if (archiveWindowLength == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "archiveWindowLength");
}
this.archiveWindowLength = archiveWindowLength;
return this;
}
@CustomType.Setter
public Builder assetName(String assetName) {
if (assetName == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "assetName");
}
this.assetName = assetName;
return this;
}
@CustomType.Setter
public Builder created(String created) {
if (created == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "created");
}
this.created = created;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder hls(@Nullable HlsResponse hls) {
this.hls = hls;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastModified(String lastModified) {
if (lastModified == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "lastModified");
}
this.lastModified = lastModified;
return this;
}
@CustomType.Setter
public Builder manifestName(@Nullable String manifestName) {
this.manifestName = manifestName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder outputSnapTime(@Nullable Double outputSnapTime) {
this.outputSnapTime = outputSnapTime;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceState(String resourceState) {
if (resourceState == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "resourceState");
}
this.resourceState = resourceState;
return this;
}
@CustomType.Setter
public Builder rewindWindowLength(@Nullable String rewindWindowLength) {
this.rewindWindowLength = rewindWindowLength;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLiveOutputResult", "type");
}
this.type = type;
return this;
}
public GetLiveOutputResult build() {
final var _resultValue = new GetLiveOutputResult();
_resultValue.archiveWindowLength = archiveWindowLength;
_resultValue.assetName = assetName;
_resultValue.created = created;
_resultValue.description = description;
_resultValue.hls = hls;
_resultValue.id = id;
_resultValue.lastModified = lastModified;
_resultValue.manifestName = manifestName;
_resultValue.name = name;
_resultValue.outputSnapTime = outputSnapTime;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceState = resourceState;
_resultValue.rewindWindowLength = rewindWindowLength;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy