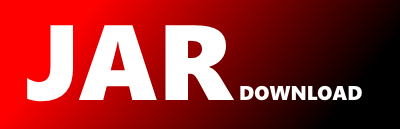
com.pulumi.azurenative.media.outputs.H264LayerResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class H264LayerResponse {
/**
* @return Whether or not adaptive B-frames are to be used when encoding this layer. If not specified, the encoder will turn it on whenever the video profile permits its use.
*
*/
private @Nullable Boolean adaptiveBFrame;
/**
* @return The number of B-frames to be used when encoding this layer. If not specified, the encoder chooses an appropriate number based on the video profile and level.
*
*/
private @Nullable Integer bFrames;
/**
* @return The average bitrate in bits per second at which to encode the input video when generating this layer. This is a required field.
*
*/
private Integer bitrate;
/**
* @return The VBV buffer window length. The value should be in ISO 8601 format. The value should be in the range [0.1-100] seconds. The default is 5 seconds (for example, PT5S).
*
*/
private @Nullable String bufferWindow;
/**
* @return The value of CRF to be used when encoding this layer. This setting takes effect when RateControlMode of video codec is set at CRF mode. The range of CRF value is between 0 and 51, where lower values would result in better quality, at the expense of higher file sizes. Higher values mean more compression, but at some point quality degradation will be noticed. Default value is 23.
*
*/
private @Nullable Double crf;
/**
* @return The entropy mode to be used for this layer. If not specified, the encoder chooses the mode that is appropriate for the profile and level.
*
*/
private @Nullable String entropyMode;
/**
* @return The frame rate (in frames per second) at which to encode this layer. The value can be in the form of M/N where M and N are integers (For example, 30000/1001), or in the form of a number (For example, 30, or 29.97). The encoder enforces constraints on allowed frame rates based on the profile and level. If it is not specified, the encoder will use the same frame rate as the input video.
*
*/
private @Nullable String frameRate;
/**
* @return The height of the output video for this layer. The value can be absolute (in pixels) or relative (in percentage). For example 50% means the output video has half as many pixels in height as the input.
*
*/
private @Nullable String height;
/**
* @return The alphanumeric label for this layer, which can be used in multiplexing different video and audio layers, or in naming the output file.
*
*/
private @Nullable String label;
/**
* @return We currently support Level up to 6.2. The value can be Auto, or a number that matches the H.264 profile. If not specified, the default is Auto, which lets the encoder choose the Level that is appropriate for this layer.
*
*/
private @Nullable String level;
/**
* @return The maximum bitrate (in bits per second), at which the VBV buffer should be assumed to refill. If not specified, defaults to the same value as bitrate.
*
*/
private @Nullable Integer maxBitrate;
/**
* @return We currently support Baseline, Main, High, High422, High444. Default is Auto.
*
*/
private @Nullable String profile;
/**
* @return The number of reference frames to be used when encoding this layer. If not specified, the encoder determines an appropriate number based on the encoder complexity setting.
*
*/
private @Nullable Integer referenceFrames;
/**
* @return The number of slices to be used when encoding this layer. If not specified, default is zero, which means that encoder will use a single slice for each frame.
*
*/
private @Nullable Integer slices;
/**
* @return The width of the output video for this layer. The value can be absolute (in pixels) or relative (in percentage). For example 50% means the output video has half as many pixels in width as the input.
*
*/
private @Nullable String width;
private H264LayerResponse() {}
/**
* @return Whether or not adaptive B-frames are to be used when encoding this layer. If not specified, the encoder will turn it on whenever the video profile permits its use.
*
*/
public Optional adaptiveBFrame() {
return Optional.ofNullable(this.adaptiveBFrame);
}
/**
* @return The number of B-frames to be used when encoding this layer. If not specified, the encoder chooses an appropriate number based on the video profile and level.
*
*/
public Optional bFrames() {
return Optional.ofNullable(this.bFrames);
}
/**
* @return The average bitrate in bits per second at which to encode the input video when generating this layer. This is a required field.
*
*/
public Integer bitrate() {
return this.bitrate;
}
/**
* @return The VBV buffer window length. The value should be in ISO 8601 format. The value should be in the range [0.1-100] seconds. The default is 5 seconds (for example, PT5S).
*
*/
public Optional bufferWindow() {
return Optional.ofNullable(this.bufferWindow);
}
/**
* @return The value of CRF to be used when encoding this layer. This setting takes effect when RateControlMode of video codec is set at CRF mode. The range of CRF value is between 0 and 51, where lower values would result in better quality, at the expense of higher file sizes. Higher values mean more compression, but at some point quality degradation will be noticed. Default value is 23.
*
*/
public Optional crf() {
return Optional.ofNullable(this.crf);
}
/**
* @return The entropy mode to be used for this layer. If not specified, the encoder chooses the mode that is appropriate for the profile and level.
*
*/
public Optional entropyMode() {
return Optional.ofNullable(this.entropyMode);
}
/**
* @return The frame rate (in frames per second) at which to encode this layer. The value can be in the form of M/N where M and N are integers (For example, 30000/1001), or in the form of a number (For example, 30, or 29.97). The encoder enforces constraints on allowed frame rates based on the profile and level. If it is not specified, the encoder will use the same frame rate as the input video.
*
*/
public Optional frameRate() {
return Optional.ofNullable(this.frameRate);
}
/**
* @return The height of the output video for this layer. The value can be absolute (in pixels) or relative (in percentage). For example 50% means the output video has half as many pixels in height as the input.
*
*/
public Optional height() {
return Optional.ofNullable(this.height);
}
/**
* @return The alphanumeric label for this layer, which can be used in multiplexing different video and audio layers, or in naming the output file.
*
*/
public Optional label() {
return Optional.ofNullable(this.label);
}
/**
* @return We currently support Level up to 6.2. The value can be Auto, or a number that matches the H.264 profile. If not specified, the default is Auto, which lets the encoder choose the Level that is appropriate for this layer.
*
*/
public Optional level() {
return Optional.ofNullable(this.level);
}
/**
* @return The maximum bitrate (in bits per second), at which the VBV buffer should be assumed to refill. If not specified, defaults to the same value as bitrate.
*
*/
public Optional maxBitrate() {
return Optional.ofNullable(this.maxBitrate);
}
/**
* @return We currently support Baseline, Main, High, High422, High444. Default is Auto.
*
*/
public Optional profile() {
return Optional.ofNullable(this.profile);
}
/**
* @return The number of reference frames to be used when encoding this layer. If not specified, the encoder determines an appropriate number based on the encoder complexity setting.
*
*/
public Optional referenceFrames() {
return Optional.ofNullable(this.referenceFrames);
}
/**
* @return The number of slices to be used when encoding this layer. If not specified, default is zero, which means that encoder will use a single slice for each frame.
*
*/
public Optional slices() {
return Optional.ofNullable(this.slices);
}
/**
* @return The width of the output video for this layer. The value can be absolute (in pixels) or relative (in percentage). For example 50% means the output video has half as many pixels in width as the input.
*
*/
public Optional width() {
return Optional.ofNullable(this.width);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(H264LayerResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean adaptiveBFrame;
private @Nullable Integer bFrames;
private Integer bitrate;
private @Nullable String bufferWindow;
private @Nullable Double crf;
private @Nullable String entropyMode;
private @Nullable String frameRate;
private @Nullable String height;
private @Nullable String label;
private @Nullable String level;
private @Nullable Integer maxBitrate;
private @Nullable String profile;
private @Nullable Integer referenceFrames;
private @Nullable Integer slices;
private @Nullable String width;
public Builder() {}
public Builder(H264LayerResponse defaults) {
Objects.requireNonNull(defaults);
this.adaptiveBFrame = defaults.adaptiveBFrame;
this.bFrames = defaults.bFrames;
this.bitrate = defaults.bitrate;
this.bufferWindow = defaults.bufferWindow;
this.crf = defaults.crf;
this.entropyMode = defaults.entropyMode;
this.frameRate = defaults.frameRate;
this.height = defaults.height;
this.label = defaults.label;
this.level = defaults.level;
this.maxBitrate = defaults.maxBitrate;
this.profile = defaults.profile;
this.referenceFrames = defaults.referenceFrames;
this.slices = defaults.slices;
this.width = defaults.width;
}
@CustomType.Setter
public Builder adaptiveBFrame(@Nullable Boolean adaptiveBFrame) {
this.adaptiveBFrame = adaptiveBFrame;
return this;
}
@CustomType.Setter
public Builder bFrames(@Nullable Integer bFrames) {
this.bFrames = bFrames;
return this;
}
@CustomType.Setter
public Builder bitrate(Integer bitrate) {
if (bitrate == null) {
throw new MissingRequiredPropertyException("H264LayerResponse", "bitrate");
}
this.bitrate = bitrate;
return this;
}
@CustomType.Setter
public Builder bufferWindow(@Nullable String bufferWindow) {
this.bufferWindow = bufferWindow;
return this;
}
@CustomType.Setter
public Builder crf(@Nullable Double crf) {
this.crf = crf;
return this;
}
@CustomType.Setter
public Builder entropyMode(@Nullable String entropyMode) {
this.entropyMode = entropyMode;
return this;
}
@CustomType.Setter
public Builder frameRate(@Nullable String frameRate) {
this.frameRate = frameRate;
return this;
}
@CustomType.Setter
public Builder height(@Nullable String height) {
this.height = height;
return this;
}
@CustomType.Setter
public Builder label(@Nullable String label) {
this.label = label;
return this;
}
@CustomType.Setter
public Builder level(@Nullable String level) {
this.level = level;
return this;
}
@CustomType.Setter
public Builder maxBitrate(@Nullable Integer maxBitrate) {
this.maxBitrate = maxBitrate;
return this;
}
@CustomType.Setter
public Builder profile(@Nullable String profile) {
this.profile = profile;
return this;
}
@CustomType.Setter
public Builder referenceFrames(@Nullable Integer referenceFrames) {
this.referenceFrames = referenceFrames;
return this;
}
@CustomType.Setter
public Builder slices(@Nullable Integer slices) {
this.slices = slices;
return this;
}
@CustomType.Setter
public Builder width(@Nullable String width) {
this.width = width;
return this;
}
public H264LayerResponse build() {
final var _resultValue = new H264LayerResponse();
_resultValue.adaptiveBFrame = adaptiveBFrame;
_resultValue.bFrames = bFrames;
_resultValue.bitrate = bitrate;
_resultValue.bufferWindow = bufferWindow;
_resultValue.crf = crf;
_resultValue.entropyMode = entropyMode;
_resultValue.frameRate = frameRate;
_resultValue.height = height;
_resultValue.label = label;
_resultValue.level = level;
_resultValue.maxBitrate = maxBitrate;
_resultValue.profile = profile;
_resultValue.referenceFrames = referenceFrames;
_resultValue.slices = slices;
_resultValue.width = width;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy