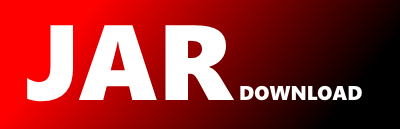
com.pulumi.azurenative.media.outputs.JobInputHttpResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.AbsoluteClipTimeResponse;
import com.pulumi.azurenative.media.outputs.FromAllInputFileResponse;
import com.pulumi.azurenative.media.outputs.FromEachInputFileResponse;
import com.pulumi.azurenative.media.outputs.InputFileResponse;
import com.pulumi.azurenative.media.outputs.UtcClipTimeResponse;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class JobInputHttpResponse {
/**
* @return Base URI for HTTPS job input. It will be concatenated with provided file names. If no base uri is given, then the provided file list is assumed to be fully qualified uris. Maximum length of 4000 characters. The query strings will not be returned in service responses to prevent sensitive data exposure.
*
*/
private @Nullable String baseUri;
/**
* @return Defines a point on the timeline of the input media at which processing will end. Defaults to the end of the input media.
*
*/
private @Nullable Either end;
/**
* @return List of files. Required for JobInputHttp. Maximum of 4000 characters each. Query strings will not be returned in service responses to prevent sensitive data exposure.
*
*/
private @Nullable List files;
/**
* @return Defines a list of InputDefinitions. For each InputDefinition, it defines a list of track selections and related metadata.
*
*/
private @Nullable List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy