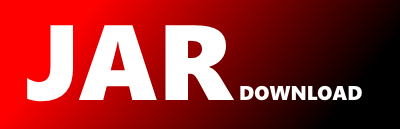
com.pulumi.azurenative.media.outputs.LiveEventTrackEventDataResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class LiveEventTrackEventDataResponse {
/**
* @return Bitrate of the track.
*
*/
private @Nullable Double bitrate;
/**
* @return Number of discontinuities detected in the last 20 seconds.
*
*/
private @Nullable Double discontinuityCount;
/**
* @return Indicates whether ingest is healthy.
*
*/
private @Nullable Boolean healthy;
/**
* @return Calculated bitrate based on data chunks coming from encoder.
*
*/
private @Nullable Double incomingBitrate;
/**
* @return Indicates the speed of delay, in seconds-per-minute, of the incoming audio or video data during the last minute. The value is greater than zero if data is arriving to the live event slower than expected in the last minute; zero if data arrived with no delay; and "n/a" if no audio or video data was received. For example, if you have a contribution encoder sending in live content, and it is slowing down due to processing issues, or network latency, it may be only able to deliver a total of 58 seconds of audio or video in a one-minute period. This would be reported as two seconds-per-minute of drift. If the encoder is able to catch up and send all 60 seconds or more of data every minute, you will see this value reported as 0. If there was a disconnection or discontinuity from the encoder, this value may still display as 0, as it does not account for breaks in the data - only data that is delayed in timestamps.
*
*/
private @Nullable String ingestDriftValue;
/**
* @return The last timestamp in UTC that a fragment arrived at the ingest endpoint.
*
*/
private @Nullable String lastFragmentArrivalTime;
/**
* @return Latest timestamp received for a track in last 20 seconds.
*
*/
private @Nullable String lastTimestamp;
/**
* @return Number of data chunks with timestamps in the past that were received in last 20 seconds.
*
*/
private @Nullable Double nonincreasingCount;
/**
* @return Number of data chunks that had overlapped timestamps in last 20 seconds.
*
*/
private @Nullable Double overlapCount;
/**
* @return State of the live event.
*
*/
private @Nullable String state;
/**
* @return Timescale in which timestamps are expressed.
*
*/
private @Nullable String timescale;
/**
* @return Name of the track.
*
*/
private @Nullable String trackName;
/**
* @return Type of the track.
*
*/
private @Nullable String trackType;
/**
* @return The language code (in BCP-47 format) of the transcription language. For example, "de-de" indicates German (Germany). The value is empty for the video track heartbeats, or when live transcription is turned off.
*
*/
private @Nullable String transcriptionLanguage;
/**
* @return This value is "On" for audio track heartbeats if live transcription is turned on, otherwise you will see an empty string. This state is only applicable to track type of "audio" for Live transcription. All other tracks will have an empty value.
*
*/
private @Nullable String transcriptionState;
/**
* @return If expected and actual bitrates differ by more than allowed limit in last 20 seconds.
*
*/
private @Nullable Boolean unexpectedBitrate;
private LiveEventTrackEventDataResponse() {}
/**
* @return Bitrate of the track.
*
*/
public Optional bitrate() {
return Optional.ofNullable(this.bitrate);
}
/**
* @return Number of discontinuities detected in the last 20 seconds.
*
*/
public Optional discontinuityCount() {
return Optional.ofNullable(this.discontinuityCount);
}
/**
* @return Indicates whether ingest is healthy.
*
*/
public Optional healthy() {
return Optional.ofNullable(this.healthy);
}
/**
* @return Calculated bitrate based on data chunks coming from encoder.
*
*/
public Optional incomingBitrate() {
return Optional.ofNullable(this.incomingBitrate);
}
/**
* @return Indicates the speed of delay, in seconds-per-minute, of the incoming audio or video data during the last minute. The value is greater than zero if data is arriving to the live event slower than expected in the last minute; zero if data arrived with no delay; and "n/a" if no audio or video data was received. For example, if you have a contribution encoder sending in live content, and it is slowing down due to processing issues, or network latency, it may be only able to deliver a total of 58 seconds of audio or video in a one-minute period. This would be reported as two seconds-per-minute of drift. If the encoder is able to catch up and send all 60 seconds or more of data every minute, you will see this value reported as 0. If there was a disconnection or discontinuity from the encoder, this value may still display as 0, as it does not account for breaks in the data - only data that is delayed in timestamps.
*
*/
public Optional ingestDriftValue() {
return Optional.ofNullable(this.ingestDriftValue);
}
/**
* @return The last timestamp in UTC that a fragment arrived at the ingest endpoint.
*
*/
public Optional lastFragmentArrivalTime() {
return Optional.ofNullable(this.lastFragmentArrivalTime);
}
/**
* @return Latest timestamp received for a track in last 20 seconds.
*
*/
public Optional lastTimestamp() {
return Optional.ofNullable(this.lastTimestamp);
}
/**
* @return Number of data chunks with timestamps in the past that were received in last 20 seconds.
*
*/
public Optional nonincreasingCount() {
return Optional.ofNullable(this.nonincreasingCount);
}
/**
* @return Number of data chunks that had overlapped timestamps in last 20 seconds.
*
*/
public Optional overlapCount() {
return Optional.ofNullable(this.overlapCount);
}
/**
* @return State of the live event.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return Timescale in which timestamps are expressed.
*
*/
public Optional timescale() {
return Optional.ofNullable(this.timescale);
}
/**
* @return Name of the track.
*
*/
public Optional trackName() {
return Optional.ofNullable(this.trackName);
}
/**
* @return Type of the track.
*
*/
public Optional trackType() {
return Optional.ofNullable(this.trackType);
}
/**
* @return The language code (in BCP-47 format) of the transcription language. For example, "de-de" indicates German (Germany). The value is empty for the video track heartbeats, or when live transcription is turned off.
*
*/
public Optional transcriptionLanguage() {
return Optional.ofNullable(this.transcriptionLanguage);
}
/**
* @return This value is "On" for audio track heartbeats if live transcription is turned on, otherwise you will see an empty string. This state is only applicable to track type of "audio" for Live transcription. All other tracks will have an empty value.
*
*/
public Optional transcriptionState() {
return Optional.ofNullable(this.transcriptionState);
}
/**
* @return If expected and actual bitrates differ by more than allowed limit in last 20 seconds.
*
*/
public Optional unexpectedBitrate() {
return Optional.ofNullable(this.unexpectedBitrate);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(LiveEventTrackEventDataResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Double bitrate;
private @Nullable Double discontinuityCount;
private @Nullable Boolean healthy;
private @Nullable Double incomingBitrate;
private @Nullable String ingestDriftValue;
private @Nullable String lastFragmentArrivalTime;
private @Nullable String lastTimestamp;
private @Nullable Double nonincreasingCount;
private @Nullable Double overlapCount;
private @Nullable String state;
private @Nullable String timescale;
private @Nullable String trackName;
private @Nullable String trackType;
private @Nullable String transcriptionLanguage;
private @Nullable String transcriptionState;
private @Nullable Boolean unexpectedBitrate;
public Builder() {}
public Builder(LiveEventTrackEventDataResponse defaults) {
Objects.requireNonNull(defaults);
this.bitrate = defaults.bitrate;
this.discontinuityCount = defaults.discontinuityCount;
this.healthy = defaults.healthy;
this.incomingBitrate = defaults.incomingBitrate;
this.ingestDriftValue = defaults.ingestDriftValue;
this.lastFragmentArrivalTime = defaults.lastFragmentArrivalTime;
this.lastTimestamp = defaults.lastTimestamp;
this.nonincreasingCount = defaults.nonincreasingCount;
this.overlapCount = defaults.overlapCount;
this.state = defaults.state;
this.timescale = defaults.timescale;
this.trackName = defaults.trackName;
this.trackType = defaults.trackType;
this.transcriptionLanguage = defaults.transcriptionLanguage;
this.transcriptionState = defaults.transcriptionState;
this.unexpectedBitrate = defaults.unexpectedBitrate;
}
@CustomType.Setter
public Builder bitrate(@Nullable Double bitrate) {
this.bitrate = bitrate;
return this;
}
@CustomType.Setter
public Builder discontinuityCount(@Nullable Double discontinuityCount) {
this.discontinuityCount = discontinuityCount;
return this;
}
@CustomType.Setter
public Builder healthy(@Nullable Boolean healthy) {
this.healthy = healthy;
return this;
}
@CustomType.Setter
public Builder incomingBitrate(@Nullable Double incomingBitrate) {
this.incomingBitrate = incomingBitrate;
return this;
}
@CustomType.Setter
public Builder ingestDriftValue(@Nullable String ingestDriftValue) {
this.ingestDriftValue = ingestDriftValue;
return this;
}
@CustomType.Setter
public Builder lastFragmentArrivalTime(@Nullable String lastFragmentArrivalTime) {
this.lastFragmentArrivalTime = lastFragmentArrivalTime;
return this;
}
@CustomType.Setter
public Builder lastTimestamp(@Nullable String lastTimestamp) {
this.lastTimestamp = lastTimestamp;
return this;
}
@CustomType.Setter
public Builder nonincreasingCount(@Nullable Double nonincreasingCount) {
this.nonincreasingCount = nonincreasingCount;
return this;
}
@CustomType.Setter
public Builder overlapCount(@Nullable Double overlapCount) {
this.overlapCount = overlapCount;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder timescale(@Nullable String timescale) {
this.timescale = timescale;
return this;
}
@CustomType.Setter
public Builder trackName(@Nullable String trackName) {
this.trackName = trackName;
return this;
}
@CustomType.Setter
public Builder trackType(@Nullable String trackType) {
this.trackType = trackType;
return this;
}
@CustomType.Setter
public Builder transcriptionLanguage(@Nullable String transcriptionLanguage) {
this.transcriptionLanguage = transcriptionLanguage;
return this;
}
@CustomType.Setter
public Builder transcriptionState(@Nullable String transcriptionState) {
this.transcriptionState = transcriptionState;
return this;
}
@CustomType.Setter
public Builder unexpectedBitrate(@Nullable Boolean unexpectedBitrate) {
this.unexpectedBitrate = unexpectedBitrate;
return this;
}
public LiveEventTrackEventDataResponse build() {
final var _resultValue = new LiveEventTrackEventDataResponse();
_resultValue.bitrate = bitrate;
_resultValue.discontinuityCount = discontinuityCount;
_resultValue.healthy = healthy;
_resultValue.incomingBitrate = incomingBitrate;
_resultValue.ingestDriftValue = ingestDriftValue;
_resultValue.lastFragmentArrivalTime = lastFragmentArrivalTime;
_resultValue.lastTimestamp = lastTimestamp;
_resultValue.nonincreasingCount = nonincreasingCount;
_resultValue.overlapCount = overlapCount;
_resultValue.state = state;
_resultValue.timescale = timescale;
_resultValue.trackName = trackName;
_resultValue.trackType = trackType;
_resultValue.transcriptionLanguage = transcriptionLanguage;
_resultValue.transcriptionState = transcriptionState;
_resultValue.unexpectedBitrate = unexpectedBitrate;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy