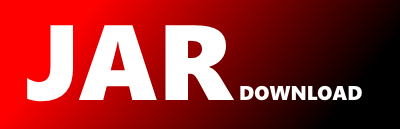
com.pulumi.azurenative.migrate.AssessmentsOperationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate;
import com.pulumi.azurenative.migrate.enums.AssessmentSizingCriterion;
import com.pulumi.azurenative.migrate.enums.AzureCurrency;
import com.pulumi.azurenative.migrate.enums.AzureDiskType;
import com.pulumi.azurenative.migrate.enums.AzureHybridUseBenefit;
import com.pulumi.azurenative.migrate.enums.AzureOfferCode;
import com.pulumi.azurenative.migrate.enums.AzurePricingTier;
import com.pulumi.azurenative.migrate.enums.AzureReservedInstance;
import com.pulumi.azurenative.migrate.enums.AzureStorageRedundancy;
import com.pulumi.azurenative.migrate.enums.AzureVmFamily;
import com.pulumi.azurenative.migrate.enums.Percentile;
import com.pulumi.azurenative.migrate.enums.ProvisioningState;
import com.pulumi.azurenative.migrate.enums.TimeRange;
import com.pulumi.azurenative.migrate.inputs.VmUptimeArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AssessmentsOperationArgs extends com.pulumi.resources.ResourceArgs {
public static final AssessmentsOperationArgs Empty = new AssessmentsOperationArgs();
/**
* Machine Assessment ARM name
*
*/
@Import(name="assessmentName")
private @Nullable Output assessmentName;
/**
* @return Machine Assessment ARM name
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy