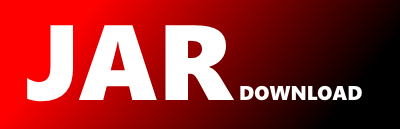
com.pulumi.azurenative.migrate.inputs.AssessmentPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.inputs;
import com.pulumi.azurenative.migrate.enums.AssessmentSizingCriterion;
import com.pulumi.azurenative.migrate.enums.AssessmentStage;
import com.pulumi.azurenative.migrate.enums.AzureDiskType;
import com.pulumi.azurenative.migrate.enums.AzureHybridUseBenefit;
import com.pulumi.azurenative.migrate.enums.AzureLocation;
import com.pulumi.azurenative.migrate.enums.AzureOfferCode;
import com.pulumi.azurenative.migrate.enums.AzurePricingTier;
import com.pulumi.azurenative.migrate.enums.AzureStorageRedundancy;
import com.pulumi.azurenative.migrate.enums.AzureVmFamily;
import com.pulumi.azurenative.migrate.enums.Currency;
import com.pulumi.azurenative.migrate.enums.Percentile;
import com.pulumi.azurenative.migrate.enums.ReservedInstance;
import com.pulumi.azurenative.migrate.enums.TimeRange;
import com.pulumi.azurenative.migrate.inputs.VmUptimeArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
/**
* Properties of an assessment.
*
*/
public final class AssessmentPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final AssessmentPropertiesArgs Empty = new AssessmentPropertiesArgs();
/**
* Storage type selected for this disk.
*
*/
@Import(name="azureDiskType", required=true)
private Output> azureDiskType;
/**
* @return Storage type selected for this disk.
*
*/
public Output> azureDiskType() {
return this.azureDiskType;
}
/**
* AHUB discount on windows virtual machines.
*
*/
@Import(name="azureHybridUseBenefit", required=true)
private Output> azureHybridUseBenefit;
/**
* @return AHUB discount on windows virtual machines.
*
*/
public Output> azureHybridUseBenefit() {
return this.azureHybridUseBenefit;
}
/**
* Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
*
*/
@Import(name="azureLocation", required=true)
private Output> azureLocation;
/**
* @return Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
*
*/
public Output> azureLocation() {
return this.azureLocation;
}
/**
* Offer code according to which cost estimation is done.
*
*/
@Import(name="azureOfferCode", required=true)
private Output> azureOfferCode;
/**
* @return Offer code according to which cost estimation is done.
*
*/
public Output> azureOfferCode() {
return this.azureOfferCode;
}
/**
* Pricing tier for Size evaluation.
*
*/
@Import(name="azurePricingTier", required=true)
private Output> azurePricingTier;
/**
* @return Pricing tier for Size evaluation.
*
*/
public Output> azurePricingTier() {
return this.azurePricingTier;
}
/**
* Storage Redundancy type offered by Azure.
*
*/
@Import(name="azureStorageRedundancy", required=true)
private Output> azureStorageRedundancy;
/**
* @return Storage Redundancy type offered by Azure.
*
*/
public Output> azureStorageRedundancy() {
return this.azureStorageRedundancy;
}
/**
* List of azure VM families.
*
*/
@Import(name="azureVmFamilies", required=true)
private Output>> azureVmFamilies;
/**
* @return List of azure VM families.
*
*/
public Output>> azureVmFamilies() {
return this.azureVmFamilies;
}
/**
* Currency to report prices in.
*
*/
@Import(name="currency", required=true)
private Output> currency;
/**
* @return Currency to report prices in.
*
*/
public Output> currency() {
return this.currency;
}
/**
* Custom discount percentage to be applied on final costs. Can be in the range [0, 100].
*
*/
@Import(name="discountPercentage", required=true)
private Output discountPercentage;
/**
* @return Custom discount percentage to be applied on final costs. Can be in the range [0, 100].
*
*/
public Output discountPercentage() {
return this.discountPercentage;
}
/**
* Percentile of performance data used to recommend Azure size.
*
*/
@Import(name="percentile", required=true)
private Output> percentile;
/**
* @return Percentile of performance data used to recommend Azure size.
*
*/
public Output> percentile() {
return this.percentile;
}
/**
* Azure reserved instance.
*
*/
@Import(name="reservedInstance", required=true)
private Output> reservedInstance;
/**
* @return Azure reserved instance.
*
*/
public Output> reservedInstance() {
return this.reservedInstance;
}
/**
* Scaling factor used over utilization data to add a performance buffer for new machines to be created in Azure. Min Value = 1.0, Max value = 1.9, Default = 1.3.
*
*/
@Import(name="scalingFactor", required=true)
private Output scalingFactor;
/**
* @return Scaling factor used over utilization data to add a performance buffer for new machines to be created in Azure. Min Value = 1.0, Max value = 1.9, Default = 1.3.
*
*/
public Output scalingFactor() {
return this.scalingFactor;
}
/**
* Assessment sizing criterion.
*
*/
@Import(name="sizingCriterion", required=true)
private Output> sizingCriterion;
/**
* @return Assessment sizing criterion.
*
*/
public Output> sizingCriterion() {
return this.sizingCriterion;
}
/**
* User configurable setting that describes the status of the assessment.
*
*/
@Import(name="stage", required=true)
private Output> stage;
/**
* @return User configurable setting that describes the status of the assessment.
*
*/
public Output> stage() {
return this.stage;
}
/**
* Time range of performance data used to recommend a size.
*
*/
@Import(name="timeRange", required=true)
private Output> timeRange;
/**
* @return Time range of performance data used to recommend a size.
*
*/
public Output> timeRange() {
return this.timeRange;
}
/**
* Specify the duration for which the VMs are up in the on-premises environment.
*
*/
@Import(name="vmUptime", required=true)
private Output vmUptime;
/**
* @return Specify the duration for which the VMs are up in the on-premises environment.
*
*/
public Output vmUptime() {
return this.vmUptime;
}
private AssessmentPropertiesArgs() {}
private AssessmentPropertiesArgs(AssessmentPropertiesArgs $) {
this.azureDiskType = $.azureDiskType;
this.azureHybridUseBenefit = $.azureHybridUseBenefit;
this.azureLocation = $.azureLocation;
this.azureOfferCode = $.azureOfferCode;
this.azurePricingTier = $.azurePricingTier;
this.azureStorageRedundancy = $.azureStorageRedundancy;
this.azureVmFamilies = $.azureVmFamilies;
this.currency = $.currency;
this.discountPercentage = $.discountPercentage;
this.percentile = $.percentile;
this.reservedInstance = $.reservedInstance;
this.scalingFactor = $.scalingFactor;
this.sizingCriterion = $.sizingCriterion;
this.stage = $.stage;
this.timeRange = $.timeRange;
this.vmUptime = $.vmUptime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AssessmentPropertiesArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private AssessmentPropertiesArgs $;
public Builder() {
$ = new AssessmentPropertiesArgs();
}
public Builder(AssessmentPropertiesArgs defaults) {
$ = new AssessmentPropertiesArgs(Objects.requireNonNull(defaults));
}
/**
* @param azureDiskType Storage type selected for this disk.
*
* @return builder
*
*/
public Builder azureDiskType(Output> azureDiskType) {
$.azureDiskType = azureDiskType;
return this;
}
/**
* @param azureDiskType Storage type selected for this disk.
*
* @return builder
*
*/
public Builder azureDiskType(Either azureDiskType) {
return azureDiskType(Output.of(azureDiskType));
}
/**
* @param azureDiskType Storage type selected for this disk.
*
* @return builder
*
*/
public Builder azureDiskType(String azureDiskType) {
return azureDiskType(Either.ofLeft(azureDiskType));
}
/**
* @param azureDiskType Storage type selected for this disk.
*
* @return builder
*
*/
public Builder azureDiskType(AzureDiskType azureDiskType) {
return azureDiskType(Either.ofRight(azureDiskType));
}
/**
* @param azureHybridUseBenefit AHUB discount on windows virtual machines.
*
* @return builder
*
*/
public Builder azureHybridUseBenefit(Output> azureHybridUseBenefit) {
$.azureHybridUseBenefit = azureHybridUseBenefit;
return this;
}
/**
* @param azureHybridUseBenefit AHUB discount on windows virtual machines.
*
* @return builder
*
*/
public Builder azureHybridUseBenefit(Either azureHybridUseBenefit) {
return azureHybridUseBenefit(Output.of(azureHybridUseBenefit));
}
/**
* @param azureHybridUseBenefit AHUB discount on windows virtual machines.
*
* @return builder
*
*/
public Builder azureHybridUseBenefit(String azureHybridUseBenefit) {
return azureHybridUseBenefit(Either.ofLeft(azureHybridUseBenefit));
}
/**
* @param azureHybridUseBenefit AHUB discount on windows virtual machines.
*
* @return builder
*
*/
public Builder azureHybridUseBenefit(AzureHybridUseBenefit azureHybridUseBenefit) {
return azureHybridUseBenefit(Either.ofRight(azureHybridUseBenefit));
}
/**
* @param azureLocation Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
*
* @return builder
*
*/
public Builder azureLocation(Output> azureLocation) {
$.azureLocation = azureLocation;
return this;
}
/**
* @param azureLocation Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
*
* @return builder
*
*/
public Builder azureLocation(Either azureLocation) {
return azureLocation(Output.of(azureLocation));
}
/**
* @param azureLocation Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
*
* @return builder
*
*/
public Builder azureLocation(String azureLocation) {
return azureLocation(Either.ofLeft(azureLocation));
}
/**
* @param azureLocation Target Azure location for which the machines should be assessed. These enums are the same as used by Compute API.
*
* @return builder
*
*/
public Builder azureLocation(AzureLocation azureLocation) {
return azureLocation(Either.ofRight(azureLocation));
}
/**
* @param azureOfferCode Offer code according to which cost estimation is done.
*
* @return builder
*
*/
public Builder azureOfferCode(Output> azureOfferCode) {
$.azureOfferCode = azureOfferCode;
return this;
}
/**
* @param azureOfferCode Offer code according to which cost estimation is done.
*
* @return builder
*
*/
public Builder azureOfferCode(Either azureOfferCode) {
return azureOfferCode(Output.of(azureOfferCode));
}
/**
* @param azureOfferCode Offer code according to which cost estimation is done.
*
* @return builder
*
*/
public Builder azureOfferCode(String azureOfferCode) {
return azureOfferCode(Either.ofLeft(azureOfferCode));
}
/**
* @param azureOfferCode Offer code according to which cost estimation is done.
*
* @return builder
*
*/
public Builder azureOfferCode(AzureOfferCode azureOfferCode) {
return azureOfferCode(Either.ofRight(azureOfferCode));
}
/**
* @param azurePricingTier Pricing tier for Size evaluation.
*
* @return builder
*
*/
public Builder azurePricingTier(Output> azurePricingTier) {
$.azurePricingTier = azurePricingTier;
return this;
}
/**
* @param azurePricingTier Pricing tier for Size evaluation.
*
* @return builder
*
*/
public Builder azurePricingTier(Either azurePricingTier) {
return azurePricingTier(Output.of(azurePricingTier));
}
/**
* @param azurePricingTier Pricing tier for Size evaluation.
*
* @return builder
*
*/
public Builder azurePricingTier(String azurePricingTier) {
return azurePricingTier(Either.ofLeft(azurePricingTier));
}
/**
* @param azurePricingTier Pricing tier for Size evaluation.
*
* @return builder
*
*/
public Builder azurePricingTier(AzurePricingTier azurePricingTier) {
return azurePricingTier(Either.ofRight(azurePricingTier));
}
/**
* @param azureStorageRedundancy Storage Redundancy type offered by Azure.
*
* @return builder
*
*/
public Builder azureStorageRedundancy(Output> azureStorageRedundancy) {
$.azureStorageRedundancy = azureStorageRedundancy;
return this;
}
/**
* @param azureStorageRedundancy Storage Redundancy type offered by Azure.
*
* @return builder
*
*/
public Builder azureStorageRedundancy(Either azureStorageRedundancy) {
return azureStorageRedundancy(Output.of(azureStorageRedundancy));
}
/**
* @param azureStorageRedundancy Storage Redundancy type offered by Azure.
*
* @return builder
*
*/
public Builder azureStorageRedundancy(String azureStorageRedundancy) {
return azureStorageRedundancy(Either.ofLeft(azureStorageRedundancy));
}
/**
* @param azureStorageRedundancy Storage Redundancy type offered by Azure.
*
* @return builder
*
*/
public Builder azureStorageRedundancy(AzureStorageRedundancy azureStorageRedundancy) {
return azureStorageRedundancy(Either.ofRight(azureStorageRedundancy));
}
/**
* @param azureVmFamilies List of azure VM families.
*
* @return builder
*
*/
public Builder azureVmFamilies(Output>> azureVmFamilies) {
$.azureVmFamilies = azureVmFamilies;
return this;
}
/**
* @param azureVmFamilies List of azure VM families.
*
* @return builder
*
*/
public Builder azureVmFamilies(List> azureVmFamilies) {
return azureVmFamilies(Output.of(azureVmFamilies));
}
/**
* @param azureVmFamilies List of azure VM families.
*
* @return builder
*
*/
public Builder azureVmFamilies(Either... azureVmFamilies) {
return azureVmFamilies(List.of(azureVmFamilies));
}
/**
* @param currency Currency to report prices in.
*
* @return builder
*
*/
public Builder currency(Output> currency) {
$.currency = currency;
return this;
}
/**
* @param currency Currency to report prices in.
*
* @return builder
*
*/
public Builder currency(Either currency) {
return currency(Output.of(currency));
}
/**
* @param currency Currency to report prices in.
*
* @return builder
*
*/
public Builder currency(String currency) {
return currency(Either.ofLeft(currency));
}
/**
* @param currency Currency to report prices in.
*
* @return builder
*
*/
public Builder currency(Currency currency) {
return currency(Either.ofRight(currency));
}
/**
* @param discountPercentage Custom discount percentage to be applied on final costs. Can be in the range [0, 100].
*
* @return builder
*
*/
public Builder discountPercentage(Output discountPercentage) {
$.discountPercentage = discountPercentage;
return this;
}
/**
* @param discountPercentage Custom discount percentage to be applied on final costs. Can be in the range [0, 100].
*
* @return builder
*
*/
public Builder discountPercentage(Double discountPercentage) {
return discountPercentage(Output.of(discountPercentage));
}
/**
* @param percentile Percentile of performance data used to recommend Azure size.
*
* @return builder
*
*/
public Builder percentile(Output> percentile) {
$.percentile = percentile;
return this;
}
/**
* @param percentile Percentile of performance data used to recommend Azure size.
*
* @return builder
*
*/
public Builder percentile(Either percentile) {
return percentile(Output.of(percentile));
}
/**
* @param percentile Percentile of performance data used to recommend Azure size.
*
* @return builder
*
*/
public Builder percentile(String percentile) {
return percentile(Either.ofLeft(percentile));
}
/**
* @param percentile Percentile of performance data used to recommend Azure size.
*
* @return builder
*
*/
public Builder percentile(Percentile percentile) {
return percentile(Either.ofRight(percentile));
}
/**
* @param reservedInstance Azure reserved instance.
*
* @return builder
*
*/
public Builder reservedInstance(Output> reservedInstance) {
$.reservedInstance = reservedInstance;
return this;
}
/**
* @param reservedInstance Azure reserved instance.
*
* @return builder
*
*/
public Builder reservedInstance(Either reservedInstance) {
return reservedInstance(Output.of(reservedInstance));
}
/**
* @param reservedInstance Azure reserved instance.
*
* @return builder
*
*/
public Builder reservedInstance(String reservedInstance) {
return reservedInstance(Either.ofLeft(reservedInstance));
}
/**
* @param reservedInstance Azure reserved instance.
*
* @return builder
*
*/
public Builder reservedInstance(ReservedInstance reservedInstance) {
return reservedInstance(Either.ofRight(reservedInstance));
}
/**
* @param scalingFactor Scaling factor used over utilization data to add a performance buffer for new machines to be created in Azure. Min Value = 1.0, Max value = 1.9, Default = 1.3.
*
* @return builder
*
*/
public Builder scalingFactor(Output scalingFactor) {
$.scalingFactor = scalingFactor;
return this;
}
/**
* @param scalingFactor Scaling factor used over utilization data to add a performance buffer for new machines to be created in Azure. Min Value = 1.0, Max value = 1.9, Default = 1.3.
*
* @return builder
*
*/
public Builder scalingFactor(Double scalingFactor) {
return scalingFactor(Output.of(scalingFactor));
}
/**
* @param sizingCriterion Assessment sizing criterion.
*
* @return builder
*
*/
public Builder sizingCriterion(Output> sizingCriterion) {
$.sizingCriterion = sizingCriterion;
return this;
}
/**
* @param sizingCriterion Assessment sizing criterion.
*
* @return builder
*
*/
public Builder sizingCriterion(Either sizingCriterion) {
return sizingCriterion(Output.of(sizingCriterion));
}
/**
* @param sizingCriterion Assessment sizing criterion.
*
* @return builder
*
*/
public Builder sizingCriterion(String sizingCriterion) {
return sizingCriterion(Either.ofLeft(sizingCriterion));
}
/**
* @param sizingCriterion Assessment sizing criterion.
*
* @return builder
*
*/
public Builder sizingCriterion(AssessmentSizingCriterion sizingCriterion) {
return sizingCriterion(Either.ofRight(sizingCriterion));
}
/**
* @param stage User configurable setting that describes the status of the assessment.
*
* @return builder
*
*/
public Builder stage(Output> stage) {
$.stage = stage;
return this;
}
/**
* @param stage User configurable setting that describes the status of the assessment.
*
* @return builder
*
*/
public Builder stage(Either stage) {
return stage(Output.of(stage));
}
/**
* @param stage User configurable setting that describes the status of the assessment.
*
* @return builder
*
*/
public Builder stage(String stage) {
return stage(Either.ofLeft(stage));
}
/**
* @param stage User configurable setting that describes the status of the assessment.
*
* @return builder
*
*/
public Builder stage(AssessmentStage stage) {
return stage(Either.ofRight(stage));
}
/**
* @param timeRange Time range of performance data used to recommend a size.
*
* @return builder
*
*/
public Builder timeRange(Output> timeRange) {
$.timeRange = timeRange;
return this;
}
/**
* @param timeRange Time range of performance data used to recommend a size.
*
* @return builder
*
*/
public Builder timeRange(Either timeRange) {
return timeRange(Output.of(timeRange));
}
/**
* @param timeRange Time range of performance data used to recommend a size.
*
* @return builder
*
*/
public Builder timeRange(String timeRange) {
return timeRange(Either.ofLeft(timeRange));
}
/**
* @param timeRange Time range of performance data used to recommend a size.
*
* @return builder
*
*/
public Builder timeRange(TimeRange timeRange) {
return timeRange(Either.ofRight(timeRange));
}
/**
* @param vmUptime Specify the duration for which the VMs are up in the on-premises environment.
*
* @return builder
*
*/
public Builder vmUptime(Output vmUptime) {
$.vmUptime = vmUptime;
return this;
}
/**
* @param vmUptime Specify the duration for which the VMs are up in the on-premises environment.
*
* @return builder
*
*/
public Builder vmUptime(VmUptimeArgs vmUptime) {
return vmUptime(Output.of(vmUptime));
}
public AssessmentPropertiesArgs build() {
if ($.azureDiskType == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azureDiskType");
}
if ($.azureHybridUseBenefit == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azureHybridUseBenefit");
}
if ($.azureLocation == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azureLocation");
}
if ($.azureOfferCode == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azureOfferCode");
}
if ($.azurePricingTier == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azurePricingTier");
}
if ($.azureStorageRedundancy == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azureStorageRedundancy");
}
if ($.azureVmFamilies == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "azureVmFamilies");
}
if ($.currency == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "currency");
}
if ($.discountPercentage == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "discountPercentage");
}
if ($.percentile == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "percentile");
}
if ($.reservedInstance == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "reservedInstance");
}
if ($.scalingFactor == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "scalingFactor");
}
if ($.sizingCriterion == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "sizingCriterion");
}
if ($.stage == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "stage");
}
if ($.timeRange == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "timeRange");
}
if ($.vmUptime == null) {
throw new MissingRequiredPropertyException("AssessmentPropertiesArgs", "vmUptime");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy