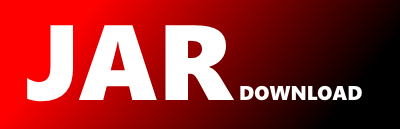
com.pulumi.azurenative.migrate.inputs.SolutionPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.inputs;
import com.pulumi.azurenative.migrate.enums.CleanupState;
import com.pulumi.azurenative.migrate.enums.Goal;
import com.pulumi.azurenative.migrate.enums.Purpose;
import com.pulumi.azurenative.migrate.enums.Status;
import com.pulumi.azurenative.migrate.enums.Tool;
import com.pulumi.azurenative.migrate.inputs.SolutionDetailsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Class for solution properties.
*
*/
public final class SolutionPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final SolutionPropertiesArgs Empty = new SolutionPropertiesArgs();
/**
* Gets or sets the cleanup state of the solution.
*
*/
@Import(name="cleanupState")
private @Nullable Output> cleanupState;
/**
* @return Gets or sets the cleanup state of the solution.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy