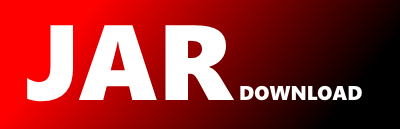
com.pulumi.azurenative.migrate.outputs.IISWebApplicationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.outputs;
import com.pulumi.azurenative.migrate.outputs.BindingResponse;
import com.pulumi.azurenative.migrate.outputs.DirectoryPathResponse;
import com.pulumi.azurenative.migrate.outputs.IISApplicationDetailsResponse;
import com.pulumi.azurenative.migrate.outputs.IISVirtualApplicationDetailsResponse;
import com.pulumi.azurenative.migrate.outputs.IISWebServerResponse;
import com.pulumi.azurenative.migrate.outputs.ResourceRequirementsResponse;
import com.pulumi.azurenative.migrate.outputs.WebApplicationConfigurationResponse;
import com.pulumi.azurenative.migrate.outputs.WebApplicationDirectoryResponse;
import com.pulumi.azurenative.migrate.outputs.WebApplicationFrameworkResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IISWebApplicationResponse {
/**
* @return Gets or sets the web application id.
*
*/
private @Nullable String applicationId;
/**
* @return Gets or sets the web application name.
*
*/
private @Nullable String applicationName;
/**
* @return Gets or sets application scratch path.
*
*/
private @Nullable String applicationScratchPath;
/**
* @return Gets or sets the list of applications for the IIS web site.
*
*/
private @Nullable List applications;
/**
* @return Gets or sets the bindings for the application.
*
*/
private @Nullable List bindings;
/**
* @return Gets or sets application configuration.
*
*/
private @Nullable List configurations;
/**
* @return Gets or sets application directories.
*
*/
private @Nullable List directories;
/**
* @return Gets or sets the discovered frameworks of application.
*
*/
private @Nullable List discoveredFrameworks;
/**
* @return Gets or sets the display name.
*
*/
private @Nullable String displayName;
/**
* @return IISWeb server.
*
*/
private @Nullable IISWebServerResponse iisWebServer;
/**
* @return Resource Requirements.
*
*/
private @Nullable ResourceRequirementsResponse limits;
/**
* @return Second level entity for virtual directories.
*
*/
private @Nullable DirectoryPathResponse path;
/**
* @return Framework specific data for a web application.
*
*/
private @Nullable WebApplicationFrameworkResponse primaryFramework;
/**
* @return Resource Requirements.
*
*/
private @Nullable ResourceRequirementsResponse requests;
/**
* @return Gets or sets the list of application units for the web site.
*
*/
private @Nullable List virtualApplications;
/**
* @return Gets or sets the web server id.
*
*/
private @Nullable String webServerId;
/**
* @return Gets or sets the web server name.
*
*/
private @Nullable String webServerName;
private IISWebApplicationResponse() {}
/**
* @return Gets or sets the web application id.
*
*/
public Optional applicationId() {
return Optional.ofNullable(this.applicationId);
}
/**
* @return Gets or sets the web application name.
*
*/
public Optional applicationName() {
return Optional.ofNullable(this.applicationName);
}
/**
* @return Gets or sets application scratch path.
*
*/
public Optional applicationScratchPath() {
return Optional.ofNullable(this.applicationScratchPath);
}
/**
* @return Gets or sets the list of applications for the IIS web site.
*
*/
public List applications() {
return this.applications == null ? List.of() : this.applications;
}
/**
* @return Gets or sets the bindings for the application.
*
*/
public List bindings() {
return this.bindings == null ? List.of() : this.bindings;
}
/**
* @return Gets or sets application configuration.
*
*/
public List configurations() {
return this.configurations == null ? List.of() : this.configurations;
}
/**
* @return Gets or sets application directories.
*
*/
public List directories() {
return this.directories == null ? List.of() : this.directories;
}
/**
* @return Gets or sets the discovered frameworks of application.
*
*/
public List discoveredFrameworks() {
return this.discoveredFrameworks == null ? List.of() : this.discoveredFrameworks;
}
/**
* @return Gets or sets the display name.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return IISWeb server.
*
*/
public Optional iisWebServer() {
return Optional.ofNullable(this.iisWebServer);
}
/**
* @return Resource Requirements.
*
*/
public Optional limits() {
return Optional.ofNullable(this.limits);
}
/**
* @return Second level entity for virtual directories.
*
*/
public Optional path() {
return Optional.ofNullable(this.path);
}
/**
* @return Framework specific data for a web application.
*
*/
public Optional primaryFramework() {
return Optional.ofNullable(this.primaryFramework);
}
/**
* @return Resource Requirements.
*
*/
public Optional requests() {
return Optional.ofNullable(this.requests);
}
/**
* @return Gets or sets the list of application units for the web site.
*
*/
public List virtualApplications() {
return this.virtualApplications == null ? List.of() : this.virtualApplications;
}
/**
* @return Gets or sets the web server id.
*
*/
public Optional webServerId() {
return Optional.ofNullable(this.webServerId);
}
/**
* @return Gets or sets the web server name.
*
*/
public Optional webServerName() {
return Optional.ofNullable(this.webServerName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IISWebApplicationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String applicationId;
private @Nullable String applicationName;
private @Nullable String applicationScratchPath;
private @Nullable List applications;
private @Nullable List bindings;
private @Nullable List configurations;
private @Nullable List directories;
private @Nullable List discoveredFrameworks;
private @Nullable String displayName;
private @Nullable IISWebServerResponse iisWebServer;
private @Nullable ResourceRequirementsResponse limits;
private @Nullable DirectoryPathResponse path;
private @Nullable WebApplicationFrameworkResponse primaryFramework;
private @Nullable ResourceRequirementsResponse requests;
private @Nullable List virtualApplications;
private @Nullable String webServerId;
private @Nullable String webServerName;
public Builder() {}
public Builder(IISWebApplicationResponse defaults) {
Objects.requireNonNull(defaults);
this.applicationId = defaults.applicationId;
this.applicationName = defaults.applicationName;
this.applicationScratchPath = defaults.applicationScratchPath;
this.applications = defaults.applications;
this.bindings = defaults.bindings;
this.configurations = defaults.configurations;
this.directories = defaults.directories;
this.discoveredFrameworks = defaults.discoveredFrameworks;
this.displayName = defaults.displayName;
this.iisWebServer = defaults.iisWebServer;
this.limits = defaults.limits;
this.path = defaults.path;
this.primaryFramework = defaults.primaryFramework;
this.requests = defaults.requests;
this.virtualApplications = defaults.virtualApplications;
this.webServerId = defaults.webServerId;
this.webServerName = defaults.webServerName;
}
@CustomType.Setter
public Builder applicationId(@Nullable String applicationId) {
this.applicationId = applicationId;
return this;
}
@CustomType.Setter
public Builder applicationName(@Nullable String applicationName) {
this.applicationName = applicationName;
return this;
}
@CustomType.Setter
public Builder applicationScratchPath(@Nullable String applicationScratchPath) {
this.applicationScratchPath = applicationScratchPath;
return this;
}
@CustomType.Setter
public Builder applications(@Nullable List applications) {
this.applications = applications;
return this;
}
public Builder applications(IISApplicationDetailsResponse... applications) {
return applications(List.of(applications));
}
@CustomType.Setter
public Builder bindings(@Nullable List bindings) {
this.bindings = bindings;
return this;
}
public Builder bindings(BindingResponse... bindings) {
return bindings(List.of(bindings));
}
@CustomType.Setter
public Builder configurations(@Nullable List configurations) {
this.configurations = configurations;
return this;
}
public Builder configurations(WebApplicationConfigurationResponse... configurations) {
return configurations(List.of(configurations));
}
@CustomType.Setter
public Builder directories(@Nullable List directories) {
this.directories = directories;
return this;
}
public Builder directories(WebApplicationDirectoryResponse... directories) {
return directories(List.of(directories));
}
@CustomType.Setter
public Builder discoveredFrameworks(@Nullable List discoveredFrameworks) {
this.discoveredFrameworks = discoveredFrameworks;
return this;
}
public Builder discoveredFrameworks(WebApplicationFrameworkResponse... discoveredFrameworks) {
return discoveredFrameworks(List.of(discoveredFrameworks));
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder iisWebServer(@Nullable IISWebServerResponse iisWebServer) {
this.iisWebServer = iisWebServer;
return this;
}
@CustomType.Setter
public Builder limits(@Nullable ResourceRequirementsResponse limits) {
this.limits = limits;
return this;
}
@CustomType.Setter
public Builder path(@Nullable DirectoryPathResponse path) {
this.path = path;
return this;
}
@CustomType.Setter
public Builder primaryFramework(@Nullable WebApplicationFrameworkResponse primaryFramework) {
this.primaryFramework = primaryFramework;
return this;
}
@CustomType.Setter
public Builder requests(@Nullable ResourceRequirementsResponse requests) {
this.requests = requests;
return this;
}
@CustomType.Setter
public Builder virtualApplications(@Nullable List virtualApplications) {
this.virtualApplications = virtualApplications;
return this;
}
public Builder virtualApplications(IISVirtualApplicationDetailsResponse... virtualApplications) {
return virtualApplications(List.of(virtualApplications));
}
@CustomType.Setter
public Builder webServerId(@Nullable String webServerId) {
this.webServerId = webServerId;
return this;
}
@CustomType.Setter
public Builder webServerName(@Nullable String webServerName) {
this.webServerName = webServerName;
return this;
}
public IISWebApplicationResponse build() {
final var _resultValue = new IISWebApplicationResponse();
_resultValue.applicationId = applicationId;
_resultValue.applicationName = applicationName;
_resultValue.applicationScratchPath = applicationScratchPath;
_resultValue.applications = applications;
_resultValue.bindings = bindings;
_resultValue.configurations = configurations;
_resultValue.directories = directories;
_resultValue.discoveredFrameworks = discoveredFrameworks;
_resultValue.displayName = displayName;
_resultValue.iisWebServer = iisWebServer;
_resultValue.limits = limits;
_resultValue.path = path;
_resultValue.primaryFramework = primaryFramework;
_resultValue.requests = requests;
_resultValue.virtualApplications = virtualApplications;
_resultValue.webServerId = webServerId;
_resultValue.webServerName = webServerName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy