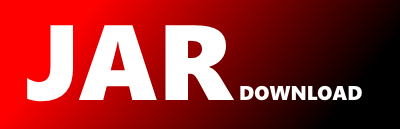
com.pulumi.azurenative.migrate.outputs.IISWebServerResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.outputs;
import com.pulumi.azurenative.migrate.outputs.OperatingSystemDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IISWebServerResponse {
/**
* @return Gets or sets the display name.
*
*/
private @Nullable String displayName;
/**
* @return Gets or sets list of ip addresses.
*
*/
private @Nullable List ipAddresses;
/**
* @return Gets or sets the list of machines.
*
*/
private @Nullable List machines;
private @Nullable OperatingSystemDetailsResponse operatingSystemDetails;
/**
* @return Gets or sets the server root configuration location.
*
*/
private @Nullable String rootConfigurationLocation;
/**
* @return Gets or sets the run as account id.
*
*/
private @Nullable String runAsAccountId;
/**
* @return Gets or sets the server FQDN.
*
*/
private @Nullable String serverFqdn;
/**
* @return Gets or sets the web server id.
*
*/
private @Nullable String serverId;
/**
* @return Gets or sets the web server name.
*
*/
private @Nullable String serverName;
/**
* @return Gets or sets the server version.
*
*/
private @Nullable String version;
/**
* @return Gets or sets the list of web applications.
*
*/
private @Nullable List webApplications;
private IISWebServerResponse() {}
/**
* @return Gets or sets the display name.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return Gets or sets list of ip addresses.
*
*/
public List ipAddresses() {
return this.ipAddresses == null ? List.of() : this.ipAddresses;
}
/**
* @return Gets or sets the list of machines.
*
*/
public List machines() {
return this.machines == null ? List.of() : this.machines;
}
public Optional operatingSystemDetails() {
return Optional.ofNullable(this.operatingSystemDetails);
}
/**
* @return Gets or sets the server root configuration location.
*
*/
public Optional rootConfigurationLocation() {
return Optional.ofNullable(this.rootConfigurationLocation);
}
/**
* @return Gets or sets the run as account id.
*
*/
public Optional runAsAccountId() {
return Optional.ofNullable(this.runAsAccountId);
}
/**
* @return Gets or sets the server FQDN.
*
*/
public Optional serverFqdn() {
return Optional.ofNullable(this.serverFqdn);
}
/**
* @return Gets or sets the web server id.
*
*/
public Optional serverId() {
return Optional.ofNullable(this.serverId);
}
/**
* @return Gets or sets the web server name.
*
*/
public Optional serverName() {
return Optional.ofNullable(this.serverName);
}
/**
* @return Gets or sets the server version.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
/**
* @return Gets or sets the list of web applications.
*
*/
public List webApplications() {
return this.webApplications == null ? List.of() : this.webApplications;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IISWebServerResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String displayName;
private @Nullable List ipAddresses;
private @Nullable List machines;
private @Nullable OperatingSystemDetailsResponse operatingSystemDetails;
private @Nullable String rootConfigurationLocation;
private @Nullable String runAsAccountId;
private @Nullable String serverFqdn;
private @Nullable String serverId;
private @Nullable String serverName;
private @Nullable String version;
private @Nullable List webApplications;
public Builder() {}
public Builder(IISWebServerResponse defaults) {
Objects.requireNonNull(defaults);
this.displayName = defaults.displayName;
this.ipAddresses = defaults.ipAddresses;
this.machines = defaults.machines;
this.operatingSystemDetails = defaults.operatingSystemDetails;
this.rootConfigurationLocation = defaults.rootConfigurationLocation;
this.runAsAccountId = defaults.runAsAccountId;
this.serverFqdn = defaults.serverFqdn;
this.serverId = defaults.serverId;
this.serverName = defaults.serverName;
this.version = defaults.version;
this.webApplications = defaults.webApplications;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder ipAddresses(@Nullable List ipAddresses) {
this.ipAddresses = ipAddresses;
return this;
}
public Builder ipAddresses(String... ipAddresses) {
return ipAddresses(List.of(ipAddresses));
}
@CustomType.Setter
public Builder machines(@Nullable List machines) {
this.machines = machines;
return this;
}
public Builder machines(String... machines) {
return machines(List.of(machines));
}
@CustomType.Setter
public Builder operatingSystemDetails(@Nullable OperatingSystemDetailsResponse operatingSystemDetails) {
this.operatingSystemDetails = operatingSystemDetails;
return this;
}
@CustomType.Setter
public Builder rootConfigurationLocation(@Nullable String rootConfigurationLocation) {
this.rootConfigurationLocation = rootConfigurationLocation;
return this;
}
@CustomType.Setter
public Builder runAsAccountId(@Nullable String runAsAccountId) {
this.runAsAccountId = runAsAccountId;
return this;
}
@CustomType.Setter
public Builder serverFqdn(@Nullable String serverFqdn) {
this.serverFqdn = serverFqdn;
return this;
}
@CustomType.Setter
public Builder serverId(@Nullable String serverId) {
this.serverId = serverId;
return this;
}
@CustomType.Setter
public Builder serverName(@Nullable String serverName) {
this.serverName = serverName;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
@CustomType.Setter
public Builder webApplications(@Nullable List webApplications) {
this.webApplications = webApplications;
return this;
}
public Builder webApplications(String... webApplications) {
return webApplications(List.of(webApplications));
}
public IISWebServerResponse build() {
final var _resultValue = new IISWebServerResponse();
_resultValue.displayName = displayName;
_resultValue.ipAddresses = ipAddresses;
_resultValue.machines = machines;
_resultValue.operatingSystemDetails = operatingSystemDetails;
_resultValue.rootConfigurationLocation = rootConfigurationLocation;
_resultValue.runAsAccountId = runAsAccountId;
_resultValue.serverFqdn = serverFqdn;
_resultValue.serverId = serverId;
_resultValue.serverName = serverName;
_resultValue.version = version;
_resultValue.webApplications = webApplications;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy