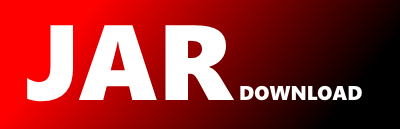
com.pulumi.azurenative.mobilenetwork.Sim Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilenetwork;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.mobilenetwork.SimArgs;
import com.pulumi.azurenative.mobilenetwork.outputs.SimPolicyResourceIdResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.SimStaticIpPropertiesResponse;
import com.pulumi.azurenative.mobilenetwork.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* SIM resource.
* Azure REST API version: 2023-06-01. Prior API version in Azure Native 1.x: 2022-04-01-preview.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
* ## Example Usage
* ### Create SIM
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.mobilenetwork.Sim;
* import com.pulumi.azurenative.mobilenetwork.SimArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.SimPolicyResourceIdArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.SimStaticIpPropertiesArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.AttachedDataNetworkResourceIdArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.SliceResourceIdArgs;
* import com.pulumi.azurenative.mobilenetwork.inputs.SimStaticIpPropertiesStaticIpArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var sim = new Sim("sim", SimArgs.builder()
* .authenticationKey("00000000000000000000000000000000")
* .deviceType("Video camera")
* .integratedCircuitCardIdentifier("8900000000000000000")
* .internationalMobileSubscriberIdentity("00000")
* .operatorKeyCode("00000000000000000000000000000000")
* .resourceGroupName("rg1")
* .simGroupName("testSimGroup")
* .simName("testSim")
* .simPolicy(SimPolicyResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.MobileNetwork/mobileNetworks/testMobileNetwork/simPolicies/MySimPolicy")
* .build())
* .staticIpConfiguration(SimStaticIpPropertiesArgs.builder()
* .attachedDataNetwork(AttachedDataNetworkResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.MobileNetwork/packetCoreControlPlanes/TestPacketCoreCP/packetCoreDataPlanes/TestPacketCoreDP/attachedDataNetworks/TestAttachedDataNetwork")
* .build())
* .slice(SliceResourceIdArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.MobileNetwork/mobileNetworks/testMobileNetwork/slices/testSlice")
* .build())
* .staticIp(SimStaticIpPropertiesStaticIpArgs.builder()
* .ipv4Address("2.4.0.1")
* .build())
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:mobilenetwork:Sim testSim /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.MobileNetwork/simGroups/{simGroupName}/sims/{simName}
* ```
*
*/
@ResourceType(type="azure-native:mobilenetwork:Sim")
public class Sim extends com.pulumi.resources.CustomResource {
/**
* An optional free-form text field that can be used to record the device type this SIM is associated with, for example 'Video camera'. The Azure portal allows SIMs to be grouped and filtered based on this value.
*
*/
@Export(name="deviceType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> deviceType;
/**
* @return An optional free-form text field that can be used to record the device type this SIM is associated with, for example 'Video camera'. The Azure portal allows SIMs to be grouped and filtered based on this value.
*
*/
public Output> deviceType() {
return Codegen.optional(this.deviceType);
}
/**
* The integrated circuit card ID (ICCID) for the SIM.
*
*/
@Export(name="integratedCircuitCardIdentifier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> integratedCircuitCardIdentifier;
/**
* @return The integrated circuit card ID (ICCID) for the SIM.
*
*/
public Output> integratedCircuitCardIdentifier() {
return Codegen.optional(this.integratedCircuitCardIdentifier);
}
/**
* The international mobile subscriber identity (IMSI) for the SIM.
*
*/
@Export(name="internationalMobileSubscriberIdentity", refs={String.class}, tree="[0]")
private Output internationalMobileSubscriberIdentity;
/**
* @return The international mobile subscriber identity (IMSI) for the SIM.
*
*/
public Output internationalMobileSubscriberIdentity() {
return this.internationalMobileSubscriberIdentity;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The provisioning state of the SIM resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the SIM resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The SIM policy used by this SIM. The SIM policy must be in the same location as the SIM.
*
*/
@Export(name="simPolicy", refs={SimPolicyResourceIdResponse.class}, tree="[0]")
private Output* @Nullable */ SimPolicyResourceIdResponse> simPolicy;
/**
* @return The SIM policy used by this SIM. The SIM policy must be in the same location as the SIM.
*
*/
public Output> simPolicy() {
return Codegen.optional(this.simPolicy);
}
/**
* The state of the SIM resource.
*
*/
@Export(name="simState", refs={String.class}, tree="[0]")
private Output simState;
/**
* @return The state of the SIM resource.
*
*/
public Output simState() {
return this.simState;
}
/**
* A dictionary of sites to the provisioning state of this SIM on that site.
*
*/
@Export(name="siteProvisioningState", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy