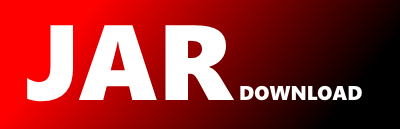
com.pulumi.azurenative.mobilenetwork.inputs.PccRuleQosPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilenetwork.inputs;
import com.pulumi.azurenative.mobilenetwork.enums.PreemptionCapability;
import com.pulumi.azurenative.mobilenetwork.enums.PreemptionVulnerability;
import com.pulumi.azurenative.mobilenetwork.inputs.AmbrArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Data flow policy rule QoS policy
*
*/
public final class PccRuleQosPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final PccRuleQosPolicyArgs Empty = new PccRuleQosPolicyArgs();
/**
* QoS Flow allocation and retention priority (ARP) level. Flows with higher priority preempt flows with lower priority, if the settings of `preemptionCapability` and `preemptionVulnerability` allow it. 1 is the highest level of priority. If this field is not specified then `5qi` is used to derive the ARP value. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters.
*
*/
@Import(name="allocationAndRetentionPriorityLevel")
private @Nullable Output allocationAndRetentionPriorityLevel;
/**
* @return QoS Flow allocation and retention priority (ARP) level. Flows with higher priority preempt flows with lower priority, if the settings of `preemptionCapability` and `preemptionVulnerability` allow it. 1 is the highest level of priority. If this field is not specified then `5qi` is used to derive the ARP value. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy