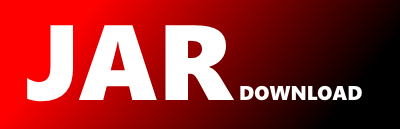
com.pulumi.azurenative.netapp.NetappFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.netapp.inputs.GetAccountArgs;
import com.pulumi.azurenative.netapp.inputs.GetAccountPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetBackupArgs;
import com.pulumi.azurenative.netapp.inputs.GetBackupPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetBackupPolicyArgs;
import com.pulumi.azurenative.netapp.inputs.GetBackupPolicyPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetBackupVaultArgs;
import com.pulumi.azurenative.netapp.inputs.GetBackupVaultPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetPoolArgs;
import com.pulumi.azurenative.netapp.inputs.GetPoolPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetSnapshotArgs;
import com.pulumi.azurenative.netapp.inputs.GetSnapshotPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetSnapshotPolicyArgs;
import com.pulumi.azurenative.netapp.inputs.GetSnapshotPolicyPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetSubvolumeArgs;
import com.pulumi.azurenative.netapp.inputs.GetSubvolumeMetadataArgs;
import com.pulumi.azurenative.netapp.inputs.GetSubvolumeMetadataPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetSubvolumePlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeGroupArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeGroupIdForLdapUserArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeGroupIdForLdapUserPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeGroupPlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumePlainArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeQuotaRuleArgs;
import com.pulumi.azurenative.netapp.inputs.GetVolumeQuotaRulePlainArgs;
import com.pulumi.azurenative.netapp.inputs.ListVolumeQuotaReportArgs;
import com.pulumi.azurenative.netapp.inputs.ListVolumeQuotaReportPlainArgs;
import com.pulumi.azurenative.netapp.inputs.ListVolumeReplicationsArgs;
import com.pulumi.azurenative.netapp.inputs.ListVolumeReplicationsPlainArgs;
import com.pulumi.azurenative.netapp.outputs.GetAccountResult;
import com.pulumi.azurenative.netapp.outputs.GetBackupPolicyResult;
import com.pulumi.azurenative.netapp.outputs.GetBackupResult;
import com.pulumi.azurenative.netapp.outputs.GetBackupVaultResult;
import com.pulumi.azurenative.netapp.outputs.GetPoolResult;
import com.pulumi.azurenative.netapp.outputs.GetSnapshotPolicyResult;
import com.pulumi.azurenative.netapp.outputs.GetSnapshotResult;
import com.pulumi.azurenative.netapp.outputs.GetSubvolumeMetadataResult;
import com.pulumi.azurenative.netapp.outputs.GetSubvolumeResult;
import com.pulumi.azurenative.netapp.outputs.GetVolumeGroupIdForLdapUserResult;
import com.pulumi.azurenative.netapp.outputs.GetVolumeGroupResult;
import com.pulumi.azurenative.netapp.outputs.GetVolumeQuotaRuleResult;
import com.pulumi.azurenative.netapp.outputs.GetVolumeResult;
import com.pulumi.azurenative.netapp.outputs.ListVolumeQuotaReportResult;
import com.pulumi.azurenative.netapp.outputs.ListVolumeReplicationsResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class NetappFunctions {
/**
* Get the NetApp account
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2019-07-01, 2022-05-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getAccount(GetAccountArgs args) {
return getAccount(args, InvokeOptions.Empty);
}
/**
* Get the NetApp account
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2019-07-01, 2022-05-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getAccountPlain(GetAccountPlainArgs args) {
return getAccountPlain(args, InvokeOptions.Empty);
}
/**
* Get the NetApp account
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2019-07-01, 2022-05-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getAccount(GetAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getAccount", TypeShape.of(GetAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Get the NetApp account
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2019-07-01, 2022-05-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getAccountPlain(GetAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getAccount", TypeShape.of(GetAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified backup of the volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getBackup(GetBackupArgs args) {
return getBackup(args, InvokeOptions.Empty);
}
/**
* Gets the specified backup of the volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getBackupPlain(GetBackupPlainArgs args) {
return getBackupPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified backup of the volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getBackup(GetBackupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getBackup", TypeShape.of(GetBackupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified backup of the volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getBackupPlain(GetBackupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getBackup", TypeShape.of(GetBackupResult.class), args, Utilities.withVersion(options));
}
/**
* Get a particular backup Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-04-01, 2021-04-01-preview, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getBackupPolicy(GetBackupPolicyArgs args) {
return getBackupPolicy(args, InvokeOptions.Empty);
}
/**
* Get a particular backup Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-04-01, 2021-04-01-preview, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getBackupPolicyPlain(GetBackupPolicyPlainArgs args) {
return getBackupPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Get a particular backup Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-04-01, 2021-04-01-preview, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getBackupPolicy(GetBackupPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getBackupPolicy", TypeShape.of(GetBackupPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Get a particular backup Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-04-01, 2021-04-01-preview, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getBackupPolicyPlain(GetBackupPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getBackupPolicy", TypeShape.of(GetBackupPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Get the Backup Vault
* Azure REST API version: 2022-11-01-preview.
*
* Other available API versions: 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getBackupVault(GetBackupVaultArgs args) {
return getBackupVault(args, InvokeOptions.Empty);
}
/**
* Get the Backup Vault
* Azure REST API version: 2022-11-01-preview.
*
* Other available API versions: 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getBackupVaultPlain(GetBackupVaultPlainArgs args) {
return getBackupVaultPlain(args, InvokeOptions.Empty);
}
/**
* Get the Backup Vault
* Azure REST API version: 2022-11-01-preview.
*
* Other available API versions: 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getBackupVault(GetBackupVaultArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getBackupVault", TypeShape.of(GetBackupVaultResult.class), args, Utilities.withVersion(options));
}
/**
* Get the Backup Vault
* Azure REST API version: 2022-11-01-preview.
*
* Other available API versions: 2023-05-01-preview, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getBackupVaultPlain(GetBackupVaultPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getBackupVault", TypeShape.of(GetBackupVaultResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified capacity pool
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-07-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getPool(GetPoolArgs args) {
return getPool(args, InvokeOptions.Empty);
}
/**
* Get details of the specified capacity pool
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-07-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getPoolPlain(GetPoolPlainArgs args) {
return getPoolPlain(args, InvokeOptions.Empty);
}
/**
* Get details of the specified capacity pool
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-07-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getPool(GetPoolArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getPool", TypeShape.of(GetPoolResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified capacity pool
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-07-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getPoolPlain(GetPoolPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getPool", TypeShape.of(GetPoolResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified snapshot
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-06-01, 2019-10-01, 2019-11-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSnapshot(GetSnapshotArgs args) {
return getSnapshot(args, InvokeOptions.Empty);
}
/**
* Get details of the specified snapshot
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-06-01, 2019-10-01, 2019-11-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSnapshotPlain(GetSnapshotPlainArgs args) {
return getSnapshotPlain(args, InvokeOptions.Empty);
}
/**
* Get details of the specified snapshot
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-06-01, 2019-10-01, 2019-11-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSnapshot(GetSnapshotArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getSnapshot", TypeShape.of(GetSnapshotResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified snapshot
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-06-01, 2019-10-01, 2019-11-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSnapshotPlain(GetSnapshotPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getSnapshot", TypeShape.of(GetSnapshotResult.class), args, Utilities.withVersion(options));
}
/**
* Get a snapshot Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSnapshotPolicy(GetSnapshotPolicyArgs args) {
return getSnapshotPolicy(args, InvokeOptions.Empty);
}
/**
* Get a snapshot Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSnapshotPolicyPlain(GetSnapshotPolicyPlainArgs args) {
return getSnapshotPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Get a snapshot Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSnapshotPolicy(GetSnapshotPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getSnapshotPolicy", TypeShape.of(GetSnapshotPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Get a snapshot Policy
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSnapshotPolicyPlain(GetSnapshotPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getSnapshotPolicy", TypeShape.of(GetSnapshotPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the path associated with the subvolumeName provided
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSubvolume(GetSubvolumeArgs args) {
return getSubvolume(args, InvokeOptions.Empty);
}
/**
* Returns the path associated with the subvolumeName provided
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSubvolumePlain(GetSubvolumePlainArgs args) {
return getSubvolumePlain(args, InvokeOptions.Empty);
}
/**
* Returns the path associated with the subvolumeName provided
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSubvolume(GetSubvolumeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getSubvolume", TypeShape.of(GetSubvolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the path associated with the subvolumeName provided
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSubvolumePlain(GetSubvolumePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getSubvolume", TypeShape.of(GetSubvolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified subvolume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSubvolumeMetadata(GetSubvolumeMetadataArgs args) {
return getSubvolumeMetadata(args, InvokeOptions.Empty);
}
/**
* Get details of the specified subvolume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSubvolumeMetadataPlain(GetSubvolumeMetadataPlainArgs args) {
return getSubvolumeMetadataPlain(args, InvokeOptions.Empty);
}
/**
* Get details of the specified subvolume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getSubvolumeMetadata(GetSubvolumeMetadataArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getSubvolumeMetadata", TypeShape.of(GetSubvolumeMetadataResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified subvolume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getSubvolumeMetadataPlain(GetSubvolumeMetadataPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getSubvolumeMetadata", TypeShape.of(GetSubvolumeMetadataResult.class), args, Utilities.withVersion(options));
}
/**
* Get the details of the specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-05-01, 2019-07-01, 2019-08-01, 2020-02-01, 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolume(GetVolumeArgs args) {
return getVolume(args, InvokeOptions.Empty);
}
/**
* Get the details of the specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-05-01, 2019-07-01, 2019-08-01, 2020-02-01, 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args) {
return getVolumePlain(args, InvokeOptions.Empty);
}
/**
* Get the details of the specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-05-01, 2019-07-01, 2019-08-01, 2020-02-01, 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolume(GetVolumeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Get the details of the specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2017-08-15, 2019-05-01, 2019-07-01, 2019-08-01, 2020-02-01, 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified volume group
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolumeGroup(GetVolumeGroupArgs args) {
return getVolumeGroup(args, InvokeOptions.Empty);
}
/**
* Get details of the specified volume group
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumeGroupPlain(GetVolumeGroupPlainArgs args) {
return getVolumeGroupPlain(args, InvokeOptions.Empty);
}
/**
* Get details of the specified volume group
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolumeGroup(GetVolumeGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getVolumeGroup", TypeShape.of(GetVolumeGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified volume group
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2021-10-01, 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumeGroupPlain(GetVolumeGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getVolumeGroup", TypeShape.of(GetVolumeGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the list of group Ids for a specific LDAP User
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolumeGroupIdForLdapUser(GetVolumeGroupIdForLdapUserArgs args) {
return getVolumeGroupIdForLdapUser(args, InvokeOptions.Empty);
}
/**
* Returns the list of group Ids for a specific LDAP User
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumeGroupIdForLdapUserPlain(GetVolumeGroupIdForLdapUserPlainArgs args) {
return getVolumeGroupIdForLdapUserPlain(args, InvokeOptions.Empty);
}
/**
* Returns the list of group Ids for a specific LDAP User
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolumeGroupIdForLdapUser(GetVolumeGroupIdForLdapUserArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getVolumeGroupIdForLdapUser", TypeShape.of(GetVolumeGroupIdForLdapUserResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the list of group Ids for a specific LDAP User
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumeGroupIdForLdapUserPlain(GetVolumeGroupIdForLdapUserPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getVolumeGroupIdForLdapUser", TypeShape.of(GetVolumeGroupIdForLdapUserResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified quota rule
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolumeQuotaRule(GetVolumeQuotaRuleArgs args) {
return getVolumeQuotaRule(args, InvokeOptions.Empty);
}
/**
* Get details of the specified quota rule
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumeQuotaRulePlain(GetVolumeQuotaRulePlainArgs args) {
return getVolumeQuotaRulePlain(args, InvokeOptions.Empty);
}
/**
* Get details of the specified quota rule
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output getVolumeQuotaRule(GetVolumeQuotaRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:getVolumeQuotaRule", TypeShape.of(GetVolumeQuotaRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Get details of the specified quota rule
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture getVolumeQuotaRulePlain(GetVolumeQuotaRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:getVolumeQuotaRule", TypeShape.of(GetVolumeQuotaRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Returns report of quotas for the volume
* Azure REST API version: 2024-03-01-preview.
*
*/
public static Output listVolumeQuotaReport(ListVolumeQuotaReportArgs args) {
return listVolumeQuotaReport(args, InvokeOptions.Empty);
}
/**
* Returns report of quotas for the volume
* Azure REST API version: 2024-03-01-preview.
*
*/
public static CompletableFuture listVolumeQuotaReportPlain(ListVolumeQuotaReportPlainArgs args) {
return listVolumeQuotaReportPlain(args, InvokeOptions.Empty);
}
/**
* Returns report of quotas for the volume
* Azure REST API version: 2024-03-01-preview.
*
*/
public static Output listVolumeQuotaReport(ListVolumeQuotaReportArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:listVolumeQuotaReport", TypeShape.of(ListVolumeQuotaReportResult.class), args, Utilities.withVersion(options));
}
/**
* Returns report of quotas for the volume
* Azure REST API version: 2024-03-01-preview.
*
*/
public static CompletableFuture listVolumeQuotaReportPlain(ListVolumeQuotaReportPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:listVolumeQuotaReport", TypeShape.of(ListVolumeQuotaReportResult.class), args, Utilities.withVersion(options));
}
/**
* List all replications for a specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output listVolumeReplications(ListVolumeReplicationsArgs args) {
return listVolumeReplications(args, InvokeOptions.Empty);
}
/**
* List all replications for a specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture listVolumeReplicationsPlain(ListVolumeReplicationsPlainArgs args) {
return listVolumeReplicationsPlain(args, InvokeOptions.Empty);
}
/**
* List all replications for a specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static Output listVolumeReplications(ListVolumeReplicationsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:netapp:listVolumeReplications", TypeShape.of(ListVolumeReplicationsResult.class), args, Utilities.withVersion(options));
}
/**
* List all replications for a specified volume
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-11-01-preview, 2023-05-01, 2023-05-01-preview, 2023-07-01, 2023-07-01-preview, 2023-11-01, 2023-11-01-preview, 2024-01-01, 2024-03-01, 2024-03-01-preview.
*
*/
public static CompletableFuture listVolumeReplicationsPlain(ListVolumeReplicationsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:netapp:listVolumeReplications", TypeShape.of(ListVolumeReplicationsResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy