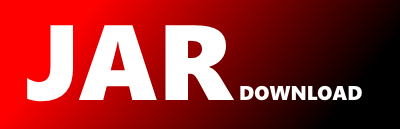
com.pulumi.azurenative.netapp.PoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp;
import com.pulumi.azurenative.netapp.enums.EncryptionType;
import com.pulumi.azurenative.netapp.enums.QosType;
import com.pulumi.azurenative.netapp.enums.ServiceLevel;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PoolArgs extends com.pulumi.resources.ResourceArgs {
public static final PoolArgs Empty = new PoolArgs();
/**
* The name of the NetApp account
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return The name of the NetApp account
*
*/
public Output accountName() {
return this.accountName;
}
/**
* If enabled (true) the pool can contain cool Access enabled volumes.
*
*/
@Import(name="coolAccess")
private @Nullable Output coolAccess;
/**
* @return If enabled (true) the pool can contain cool Access enabled volumes.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy