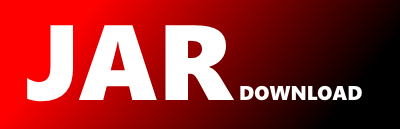
com.pulumi.azurenative.netapp.outputs.ActiveDirectoryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp.outputs;
import com.pulumi.azurenative.netapp.outputs.LdapSearchScopeOptResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ActiveDirectoryResponse {
/**
* @return Id of the Active Directory
*
*/
private @Nullable String activeDirectoryId;
/**
* @return Name of the active directory machine. This optional parameter is used only while creating kerberos volume
*
*/
private @Nullable String adName;
/**
* @return Users to be added to the Built-in Administrators active directory group. A list of unique usernames without domain specifier
*
*/
private @Nullable List administrators;
/**
* @return If enabled, AES encryption will be enabled for SMB communication.
*
*/
private @Nullable Boolean aesEncryption;
/**
* @return If enabled, NFS client local users can also (in addition to LDAP users) access the NFS volumes.
*
*/
private @Nullable Boolean allowLocalNfsUsersWithLdap;
/**
* @return Users to be added to the Built-in Backup Operator active directory group. A list of unique usernames without domain specifier
*
*/
private @Nullable List backupOperators;
/**
* @return Comma separated list of DNS server IP addresses (IPv4 only) for the Active Directory domain
*
*/
private @Nullable String dns;
/**
* @return Name of the Active Directory domain
*
*/
private @Nullable String domain;
/**
* @return If enabled, Traffic between the SMB server to Domain Controller (DC) will be encrypted.
*
*/
private @Nullable Boolean encryptDCConnections;
/**
* @return kdc server IP addresses for the active directory machine. This optional parameter is used only while creating kerberos volume.
*
*/
private @Nullable String kdcIP;
/**
* @return Specifies whether or not the LDAP traffic needs to be secured via TLS.
*
*/
private @Nullable Boolean ldapOverTLS;
/**
* @return LDAP Search scope options
*
*/
private @Nullable LdapSearchScopeOptResponse ldapSearchScope;
/**
* @return Specifies whether or not the LDAP traffic needs to be signed.
*
*/
private @Nullable Boolean ldapSigning;
/**
* @return The Organizational Unit (OU) within the Windows Active Directory
*
*/
private @Nullable String organizationalUnit;
/**
* @return Plain text password of Active Directory domain administrator, value is masked in the response
*
*/
private @Nullable String password;
/**
* @return Comma separated list of IPv4 addresses of preferred servers for LDAP client. At most two comma separated IPv4 addresses can be passed.
*
*/
private @Nullable String preferredServersForLdapClient;
/**
* @return Domain Users in the Active directory to be given SeSecurityPrivilege privilege (Needed for SMB Continuously available shares for SQL). A list of unique usernames without domain specifier
*
*/
private @Nullable List securityOperators;
/**
* @return When LDAP over SSL/TLS is enabled, the LDAP client is required to have base64 encoded Active Directory Certificate Service's self-signed root CA certificate, this optional parameter is used only for dual protocol with LDAP user-mapping volumes.
*
*/
private @Nullable String serverRootCACertificate;
/**
* @return The Active Directory site the service will limit Domain Controller discovery to
*
*/
private @Nullable String site;
/**
* @return NetBIOS name of the SMB server. This name will be registered as a computer account in the AD and used to mount volumes
*
*/
private @Nullable String smbServerName;
/**
* @return Status of the Active Directory
*
*/
private String status;
/**
* @return Any details in regards to the Status of the Active Directory
*
*/
private String statusDetails;
/**
* @return A domain user account with permission to create machine accounts
*
*/
private @Nullable String username;
private ActiveDirectoryResponse() {}
/**
* @return Id of the Active Directory
*
*/
public Optional activeDirectoryId() {
return Optional.ofNullable(this.activeDirectoryId);
}
/**
* @return Name of the active directory machine. This optional parameter is used only while creating kerberos volume
*
*/
public Optional adName() {
return Optional.ofNullable(this.adName);
}
/**
* @return Users to be added to the Built-in Administrators active directory group. A list of unique usernames without domain specifier
*
*/
public List administrators() {
return this.administrators == null ? List.of() : this.administrators;
}
/**
* @return If enabled, AES encryption will be enabled for SMB communication.
*
*/
public Optional aesEncryption() {
return Optional.ofNullable(this.aesEncryption);
}
/**
* @return If enabled, NFS client local users can also (in addition to LDAP users) access the NFS volumes.
*
*/
public Optional allowLocalNfsUsersWithLdap() {
return Optional.ofNullable(this.allowLocalNfsUsersWithLdap);
}
/**
* @return Users to be added to the Built-in Backup Operator active directory group. A list of unique usernames without domain specifier
*
*/
public List backupOperators() {
return this.backupOperators == null ? List.of() : this.backupOperators;
}
/**
* @return Comma separated list of DNS server IP addresses (IPv4 only) for the Active Directory domain
*
*/
public Optional dns() {
return Optional.ofNullable(this.dns);
}
/**
* @return Name of the Active Directory domain
*
*/
public Optional domain() {
return Optional.ofNullable(this.domain);
}
/**
* @return If enabled, Traffic between the SMB server to Domain Controller (DC) will be encrypted.
*
*/
public Optional encryptDCConnections() {
return Optional.ofNullable(this.encryptDCConnections);
}
/**
* @return kdc server IP addresses for the active directory machine. This optional parameter is used only while creating kerberos volume.
*
*/
public Optional kdcIP() {
return Optional.ofNullable(this.kdcIP);
}
/**
* @return Specifies whether or not the LDAP traffic needs to be secured via TLS.
*
*/
public Optional ldapOverTLS() {
return Optional.ofNullable(this.ldapOverTLS);
}
/**
* @return LDAP Search scope options
*
*/
public Optional ldapSearchScope() {
return Optional.ofNullable(this.ldapSearchScope);
}
/**
* @return Specifies whether or not the LDAP traffic needs to be signed.
*
*/
public Optional ldapSigning() {
return Optional.ofNullable(this.ldapSigning);
}
/**
* @return The Organizational Unit (OU) within the Windows Active Directory
*
*/
public Optional organizationalUnit() {
return Optional.ofNullable(this.organizationalUnit);
}
/**
* @return Plain text password of Active Directory domain administrator, value is masked in the response
*
*/
public Optional password() {
return Optional.ofNullable(this.password);
}
/**
* @return Comma separated list of IPv4 addresses of preferred servers for LDAP client. At most two comma separated IPv4 addresses can be passed.
*
*/
public Optional preferredServersForLdapClient() {
return Optional.ofNullable(this.preferredServersForLdapClient);
}
/**
* @return Domain Users in the Active directory to be given SeSecurityPrivilege privilege (Needed for SMB Continuously available shares for SQL). A list of unique usernames without domain specifier
*
*/
public List securityOperators() {
return this.securityOperators == null ? List.of() : this.securityOperators;
}
/**
* @return When LDAP over SSL/TLS is enabled, the LDAP client is required to have base64 encoded Active Directory Certificate Service's self-signed root CA certificate, this optional parameter is used only for dual protocol with LDAP user-mapping volumes.
*
*/
public Optional serverRootCACertificate() {
return Optional.ofNullable(this.serverRootCACertificate);
}
/**
* @return The Active Directory site the service will limit Domain Controller discovery to
*
*/
public Optional site() {
return Optional.ofNullable(this.site);
}
/**
* @return NetBIOS name of the SMB server. This name will be registered as a computer account in the AD and used to mount volumes
*
*/
public Optional smbServerName() {
return Optional.ofNullable(this.smbServerName);
}
/**
* @return Status of the Active Directory
*
*/
public String status() {
return this.status;
}
/**
* @return Any details in regards to the Status of the Active Directory
*
*/
public String statusDetails() {
return this.statusDetails;
}
/**
* @return A domain user account with permission to create machine accounts
*
*/
public Optional username() {
return Optional.ofNullable(this.username);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ActiveDirectoryResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String activeDirectoryId;
private @Nullable String adName;
private @Nullable List administrators;
private @Nullable Boolean aesEncryption;
private @Nullable Boolean allowLocalNfsUsersWithLdap;
private @Nullable List backupOperators;
private @Nullable String dns;
private @Nullable String domain;
private @Nullable Boolean encryptDCConnections;
private @Nullable String kdcIP;
private @Nullable Boolean ldapOverTLS;
private @Nullable LdapSearchScopeOptResponse ldapSearchScope;
private @Nullable Boolean ldapSigning;
private @Nullable String organizationalUnit;
private @Nullable String password;
private @Nullable String preferredServersForLdapClient;
private @Nullable List securityOperators;
private @Nullable String serverRootCACertificate;
private @Nullable String site;
private @Nullable String smbServerName;
private String status;
private String statusDetails;
private @Nullable String username;
public Builder() {}
public Builder(ActiveDirectoryResponse defaults) {
Objects.requireNonNull(defaults);
this.activeDirectoryId = defaults.activeDirectoryId;
this.adName = defaults.adName;
this.administrators = defaults.administrators;
this.aesEncryption = defaults.aesEncryption;
this.allowLocalNfsUsersWithLdap = defaults.allowLocalNfsUsersWithLdap;
this.backupOperators = defaults.backupOperators;
this.dns = defaults.dns;
this.domain = defaults.domain;
this.encryptDCConnections = defaults.encryptDCConnections;
this.kdcIP = defaults.kdcIP;
this.ldapOverTLS = defaults.ldapOverTLS;
this.ldapSearchScope = defaults.ldapSearchScope;
this.ldapSigning = defaults.ldapSigning;
this.organizationalUnit = defaults.organizationalUnit;
this.password = defaults.password;
this.preferredServersForLdapClient = defaults.preferredServersForLdapClient;
this.securityOperators = defaults.securityOperators;
this.serverRootCACertificate = defaults.serverRootCACertificate;
this.site = defaults.site;
this.smbServerName = defaults.smbServerName;
this.status = defaults.status;
this.statusDetails = defaults.statusDetails;
this.username = defaults.username;
}
@CustomType.Setter
public Builder activeDirectoryId(@Nullable String activeDirectoryId) {
this.activeDirectoryId = activeDirectoryId;
return this;
}
@CustomType.Setter
public Builder adName(@Nullable String adName) {
this.adName = adName;
return this;
}
@CustomType.Setter
public Builder administrators(@Nullable List administrators) {
this.administrators = administrators;
return this;
}
public Builder administrators(String... administrators) {
return administrators(List.of(administrators));
}
@CustomType.Setter
public Builder aesEncryption(@Nullable Boolean aesEncryption) {
this.aesEncryption = aesEncryption;
return this;
}
@CustomType.Setter
public Builder allowLocalNfsUsersWithLdap(@Nullable Boolean allowLocalNfsUsersWithLdap) {
this.allowLocalNfsUsersWithLdap = allowLocalNfsUsersWithLdap;
return this;
}
@CustomType.Setter
public Builder backupOperators(@Nullable List backupOperators) {
this.backupOperators = backupOperators;
return this;
}
public Builder backupOperators(String... backupOperators) {
return backupOperators(List.of(backupOperators));
}
@CustomType.Setter
public Builder dns(@Nullable String dns) {
this.dns = dns;
return this;
}
@CustomType.Setter
public Builder domain(@Nullable String domain) {
this.domain = domain;
return this;
}
@CustomType.Setter
public Builder encryptDCConnections(@Nullable Boolean encryptDCConnections) {
this.encryptDCConnections = encryptDCConnections;
return this;
}
@CustomType.Setter
public Builder kdcIP(@Nullable String kdcIP) {
this.kdcIP = kdcIP;
return this;
}
@CustomType.Setter
public Builder ldapOverTLS(@Nullable Boolean ldapOverTLS) {
this.ldapOverTLS = ldapOverTLS;
return this;
}
@CustomType.Setter
public Builder ldapSearchScope(@Nullable LdapSearchScopeOptResponse ldapSearchScope) {
this.ldapSearchScope = ldapSearchScope;
return this;
}
@CustomType.Setter
public Builder ldapSigning(@Nullable Boolean ldapSigning) {
this.ldapSigning = ldapSigning;
return this;
}
@CustomType.Setter
public Builder organizationalUnit(@Nullable String organizationalUnit) {
this.organizationalUnit = organizationalUnit;
return this;
}
@CustomType.Setter
public Builder password(@Nullable String password) {
this.password = password;
return this;
}
@CustomType.Setter
public Builder preferredServersForLdapClient(@Nullable String preferredServersForLdapClient) {
this.preferredServersForLdapClient = preferredServersForLdapClient;
return this;
}
@CustomType.Setter
public Builder securityOperators(@Nullable List securityOperators) {
this.securityOperators = securityOperators;
return this;
}
public Builder securityOperators(String... securityOperators) {
return securityOperators(List.of(securityOperators));
}
@CustomType.Setter
public Builder serverRootCACertificate(@Nullable String serverRootCACertificate) {
this.serverRootCACertificate = serverRootCACertificate;
return this;
}
@CustomType.Setter
public Builder site(@Nullable String site) {
this.site = site;
return this;
}
@CustomType.Setter
public Builder smbServerName(@Nullable String smbServerName) {
this.smbServerName = smbServerName;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("ActiveDirectoryResponse", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder statusDetails(String statusDetails) {
if (statusDetails == null) {
throw new MissingRequiredPropertyException("ActiveDirectoryResponse", "statusDetails");
}
this.statusDetails = statusDetails;
return this;
}
@CustomType.Setter
public Builder username(@Nullable String username) {
this.username = username;
return this;
}
public ActiveDirectoryResponse build() {
final var _resultValue = new ActiveDirectoryResponse();
_resultValue.activeDirectoryId = activeDirectoryId;
_resultValue.adName = adName;
_resultValue.administrators = administrators;
_resultValue.aesEncryption = aesEncryption;
_resultValue.allowLocalNfsUsersWithLdap = allowLocalNfsUsersWithLdap;
_resultValue.backupOperators = backupOperators;
_resultValue.dns = dns;
_resultValue.domain = domain;
_resultValue.encryptDCConnections = encryptDCConnections;
_resultValue.kdcIP = kdcIP;
_resultValue.ldapOverTLS = ldapOverTLS;
_resultValue.ldapSearchScope = ldapSearchScope;
_resultValue.ldapSigning = ldapSigning;
_resultValue.organizationalUnit = organizationalUnit;
_resultValue.password = password;
_resultValue.preferredServersForLdapClient = preferredServersForLdapClient;
_resultValue.securityOperators = securityOperators;
_resultValue.serverRootCACertificate = serverRootCACertificate;
_resultValue.site = site;
_resultValue.smbServerName = smbServerName;
_resultValue.status = status;
_resultValue.statusDetails = statusDetails;
_resultValue.username = username;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy