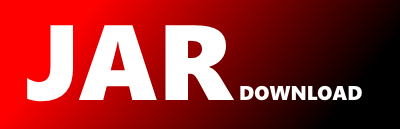
com.pulumi.azurenative.netapp.outputs.GetPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp.outputs;
import com.pulumi.azurenative.netapp.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPoolResult {
/**
* @return If enabled (true) the pool can contain cool Access enabled volumes.
*
*/
private @Nullable Boolean coolAccess;
/**
* @return Encryption type of the capacity pool, set encryption type for data at rest for this pool and all volumes in it. This value can only be set when creating new pool.
*
*/
private @Nullable String encryptionType;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return UUID v4 used to identify the Pool
*
*/
private String poolId;
/**
* @return Azure lifecycle management
*
*/
private String provisioningState;
/**
* @return The qos type of the pool
*
*/
private @Nullable String qosType;
/**
* @return The service level of the file system
*
*/
private String serviceLevel;
/**
* @return Provisioned size of the pool (in bytes). Allowed values are in 1TiB chunks (value must be multiply of 4398046511104).
*
*/
private Double size;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Total throughput of pool in MiB/s
*
*/
private Double totalThroughputMibps;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Utilized throughput of pool in MiB/s
*
*/
private Double utilizedThroughputMibps;
private GetPoolResult() {}
/**
* @return If enabled (true) the pool can contain cool Access enabled volumes.
*
*/
public Optional coolAccess() {
return Optional.ofNullable(this.coolAccess);
}
/**
* @return Encryption type of the capacity pool, set encryption type for data at rest for this pool and all volumes in it. This value can only be set when creating new pool.
*
*/
public Optional encryptionType() {
return Optional.ofNullable(this.encryptionType);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return UUID v4 used to identify the Pool
*
*/
public String poolId() {
return this.poolId;
}
/**
* @return Azure lifecycle management
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The qos type of the pool
*
*/
public Optional qosType() {
return Optional.ofNullable(this.qosType);
}
/**
* @return The service level of the file system
*
*/
public String serviceLevel() {
return this.serviceLevel;
}
/**
* @return Provisioned size of the pool (in bytes). Allowed values are in 1TiB chunks (value must be multiply of 4398046511104).
*
*/
public Double size() {
return this.size;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Total throughput of pool in MiB/s
*
*/
public Double totalThroughputMibps() {
return this.totalThroughputMibps;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Utilized throughput of pool in MiB/s
*
*/
public Double utilizedThroughputMibps() {
return this.utilizedThroughputMibps;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean coolAccess;
private @Nullable String encryptionType;
private String etag;
private String id;
private String location;
private String name;
private String poolId;
private String provisioningState;
private @Nullable String qosType;
private String serviceLevel;
private Double size;
private SystemDataResponse systemData;
private @Nullable Map tags;
private Double totalThroughputMibps;
private String type;
private Double utilizedThroughputMibps;
public Builder() {}
public Builder(GetPoolResult defaults) {
Objects.requireNonNull(defaults);
this.coolAccess = defaults.coolAccess;
this.encryptionType = defaults.encryptionType;
this.etag = defaults.etag;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.poolId = defaults.poolId;
this.provisioningState = defaults.provisioningState;
this.qosType = defaults.qosType;
this.serviceLevel = defaults.serviceLevel;
this.size = defaults.size;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.totalThroughputMibps = defaults.totalThroughputMibps;
this.type = defaults.type;
this.utilizedThroughputMibps = defaults.utilizedThroughputMibps;
}
@CustomType.Setter
public Builder coolAccess(@Nullable Boolean coolAccess) {
this.coolAccess = coolAccess;
return this;
}
@CustomType.Setter
public Builder encryptionType(@Nullable String encryptionType) {
this.encryptionType = encryptionType;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder poolId(String poolId) {
if (poolId == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "poolId");
}
this.poolId = poolId;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder qosType(@Nullable String qosType) {
this.qosType = qosType;
return this;
}
@CustomType.Setter
public Builder serviceLevel(String serviceLevel) {
if (serviceLevel == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "serviceLevel");
}
this.serviceLevel = serviceLevel;
return this;
}
@CustomType.Setter
public Builder size(Double size) {
if (size == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "size");
}
this.size = size;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder totalThroughputMibps(Double totalThroughputMibps) {
if (totalThroughputMibps == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "totalThroughputMibps");
}
this.totalThroughputMibps = totalThroughputMibps;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder utilizedThroughputMibps(Double utilizedThroughputMibps) {
if (utilizedThroughputMibps == null) {
throw new MissingRequiredPropertyException("GetPoolResult", "utilizedThroughputMibps");
}
this.utilizedThroughputMibps = utilizedThroughputMibps;
return this;
}
public GetPoolResult build() {
final var _resultValue = new GetPoolResult();
_resultValue.coolAccess = coolAccess;
_resultValue.encryptionType = encryptionType;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.poolId = poolId;
_resultValue.provisioningState = provisioningState;
_resultValue.qosType = qosType;
_resultValue.serviceLevel = serviceLevel;
_resultValue.size = size;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.totalThroughputMibps = totalThroughputMibps;
_resultValue.type = type;
_resultValue.utilizedThroughputMibps = utilizedThroughputMibps;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy