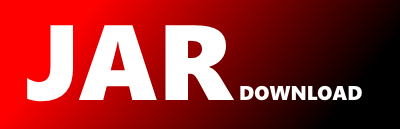
com.pulumi.azurenative.network.ApplicationGateway Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.network.ApplicationGatewayArgs;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayAuthenticationCertificateResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayAutoscaleConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayBackendAddressPoolResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayBackendHttpSettingsResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayBackendSettingsResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayCustomErrorResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayFrontendIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayFrontendPortResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayGlobalConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayHttpListenerResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayListenerResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayLoadDistributionPolicyResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayPrivateEndpointConnectionResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayPrivateLinkConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayProbeResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayRedirectConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayRequestRoutingRuleResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayRewriteRuleSetResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayRoutingRuleResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewaySkuResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewaySslCertificateResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewaySslPolicyResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewaySslProfileResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayTrustedClientCertificateResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayTrustedRootCertificateResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayUrlPathMapResponse;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayWebApplicationFirewallConfigurationResponse;
import com.pulumi.azurenative.network.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Application gateway resource.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-11-01.
*
* Other available API versions: 2015-05-01-preview, 2019-06-01, 2019-08-01, 2023-04-01, 2023-05-01, 2023-06-01, 2023-09-01, 2023-11-01, 2024-01-01, 2024-03-01.
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:network:ApplicationGateway appgw /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/applicationGateways/{applicationGatewayName}
* ```
*
*/
@ResourceType(type="azure-native:network:ApplicationGateway")
public class ApplicationGateway extends com.pulumi.resources.CustomResource {
/**
* Authentication certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="authenticationCertificates", refs={List.class,ApplicationGatewayAuthenticationCertificateResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> authenticationCertificates;
/**
* @return Authentication certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> authenticationCertificates() {
return Codegen.optional(this.authenticationCertificates);
}
/**
* Autoscale Configuration.
*
*/
@Export(name="autoscaleConfiguration", refs={ApplicationGatewayAutoscaleConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationGatewayAutoscaleConfigurationResponse> autoscaleConfiguration;
/**
* @return Autoscale Configuration.
*
*/
public Output> autoscaleConfiguration() {
return Codegen.optional(this.autoscaleConfiguration);
}
/**
* Backend address pool of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="backendAddressPools", refs={List.class,ApplicationGatewayBackendAddressPoolResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> backendAddressPools;
/**
* @return Backend address pool of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> backendAddressPools() {
return Codegen.optional(this.backendAddressPools);
}
/**
* Backend http settings of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="backendHttpSettingsCollection", refs={List.class,ApplicationGatewayBackendHttpSettingsResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> backendHttpSettingsCollection;
/**
* @return Backend http settings of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> backendHttpSettingsCollection() {
return Codegen.optional(this.backendHttpSettingsCollection);
}
/**
* Backend settings of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="backendSettingsCollection", refs={List.class,ApplicationGatewayBackendSettingsResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> backendSettingsCollection;
/**
* @return Backend settings of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> backendSettingsCollection() {
return Codegen.optional(this.backendSettingsCollection);
}
/**
* Custom error configurations of the application gateway resource.
*
*/
@Export(name="customErrorConfigurations", refs={List.class,ApplicationGatewayCustomErrorResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> customErrorConfigurations;
/**
* @return Custom error configurations of the application gateway resource.
*
*/
public Output>> customErrorConfigurations() {
return Codegen.optional(this.customErrorConfigurations);
}
/**
* The default predefined SSL Policy applied on the application gateway resource.
*
*/
@Export(name="defaultPredefinedSslPolicy", refs={String.class}, tree="[0]")
private Output defaultPredefinedSslPolicy;
/**
* @return The default predefined SSL Policy applied on the application gateway resource.
*
*/
public Output defaultPredefinedSslPolicy() {
return this.defaultPredefinedSslPolicy;
}
/**
* Whether FIPS is enabled on the application gateway resource.
*
*/
@Export(name="enableFips", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableFips;
/**
* @return Whether FIPS is enabled on the application gateway resource.
*
*/
public Output> enableFips() {
return Codegen.optional(this.enableFips);
}
/**
* Whether HTTP2 is enabled on the application gateway resource.
*
*/
@Export(name="enableHttp2", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableHttp2;
/**
* @return Whether HTTP2 is enabled on the application gateway resource.
*
*/
public Output> enableHttp2() {
return Codegen.optional(this.enableHttp2);
}
/**
* A unique read-only string that changes whenever the resource is updated.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public Output etag() {
return this.etag;
}
/**
* Reference to the FirewallPolicy resource.
*
*/
@Export(name="firewallPolicy", refs={SubResourceResponse.class}, tree="[0]")
private Output* @Nullable */ SubResourceResponse> firewallPolicy;
/**
* @return Reference to the FirewallPolicy resource.
*
*/
public Output> firewallPolicy() {
return Codegen.optional(this.firewallPolicy);
}
/**
* If true, associates a firewall policy with an application gateway regardless whether the policy differs from the WAF Config.
*
*/
@Export(name="forceFirewallPolicyAssociation", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceFirewallPolicyAssociation;
/**
* @return If true, associates a firewall policy with an application gateway regardless whether the policy differs from the WAF Config.
*
*/
public Output> forceFirewallPolicyAssociation() {
return Codegen.optional(this.forceFirewallPolicyAssociation);
}
/**
* Frontend IP addresses of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="frontendIPConfigurations", refs={List.class,ApplicationGatewayFrontendIPConfigurationResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> frontendIPConfigurations;
/**
* @return Frontend IP addresses of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> frontendIPConfigurations() {
return Codegen.optional(this.frontendIPConfigurations);
}
/**
* Frontend ports of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="frontendPorts", refs={List.class,ApplicationGatewayFrontendPortResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> frontendPorts;
/**
* @return Frontend ports of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> frontendPorts() {
return Codegen.optional(this.frontendPorts);
}
/**
* Subnets of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="gatewayIPConfigurations", refs={List.class,ApplicationGatewayIPConfigurationResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> gatewayIPConfigurations;
/**
* @return Subnets of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> gatewayIPConfigurations() {
return Codegen.optional(this.gatewayIPConfigurations);
}
/**
* Global Configuration.
*
*/
@Export(name="globalConfiguration", refs={ApplicationGatewayGlobalConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationGatewayGlobalConfigurationResponse> globalConfiguration;
/**
* @return Global Configuration.
*
*/
public Output> globalConfiguration() {
return Codegen.optional(this.globalConfiguration);
}
/**
* Http listeners of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="httpListeners", refs={List.class,ApplicationGatewayHttpListenerResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> httpListeners;
/**
* @return Http listeners of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> httpListeners() {
return Codegen.optional(this.httpListeners);
}
/**
* The identity of the application gateway, if configured.
*
*/
@Export(name="identity", refs={ManagedServiceIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ ManagedServiceIdentityResponse> identity;
/**
* @return The identity of the application gateway, if configured.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* Listeners of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="listeners", refs={List.class,ApplicationGatewayListenerResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> listeners;
/**
* @return Listeners of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> listeners() {
return Codegen.optional(this.listeners);
}
/**
* Load distribution policies of the application gateway resource.
*
*/
@Export(name="loadDistributionPolicies", refs={List.class,ApplicationGatewayLoadDistributionPolicyResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> loadDistributionPolicies;
/**
* @return Load distribution policies of the application gateway resource.
*
*/
public Output>> loadDistributionPolicies() {
return Codegen.optional(this.loadDistributionPolicies);
}
/**
* Resource location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return Resource location.
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* Operational state of the application gateway resource.
*
*/
@Export(name="operationalState", refs={String.class}, tree="[0]")
private Output operationalState;
/**
* @return Operational state of the application gateway resource.
*
*/
public Output operationalState() {
return this.operationalState;
}
/**
* Private Endpoint connections on application gateway.
*
*/
@Export(name="privateEndpointConnections", refs={List.class,ApplicationGatewayPrivateEndpointConnectionResponse.class}, tree="[0,1]")
private Output> privateEndpointConnections;
/**
* @return Private Endpoint connections on application gateway.
*
*/
public Output> privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* PrivateLink configurations on application gateway.
*
*/
@Export(name="privateLinkConfigurations", refs={List.class,ApplicationGatewayPrivateLinkConfigurationResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> privateLinkConfigurations;
/**
* @return PrivateLink configurations on application gateway.
*
*/
public Output>> privateLinkConfigurations() {
return Codegen.optional(this.privateLinkConfigurations);
}
/**
* Probes of the application gateway resource.
*
*/
@Export(name="probes", refs={List.class,ApplicationGatewayProbeResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> probes;
/**
* @return Probes of the application gateway resource.
*
*/
public Output>> probes() {
return Codegen.optional(this.probes);
}
/**
* The provisioning state of the application gateway resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the application gateway resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Redirect configurations of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="redirectConfigurations", refs={List.class,ApplicationGatewayRedirectConfigurationResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> redirectConfigurations;
/**
* @return Redirect configurations of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> redirectConfigurations() {
return Codegen.optional(this.redirectConfigurations);
}
/**
* Request routing rules of the application gateway resource.
*
*/
@Export(name="requestRoutingRules", refs={List.class,ApplicationGatewayRequestRoutingRuleResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> requestRoutingRules;
/**
* @return Request routing rules of the application gateway resource.
*
*/
public Output>> requestRoutingRules() {
return Codegen.optional(this.requestRoutingRules);
}
/**
* The resource GUID property of the application gateway resource.
*
*/
@Export(name="resourceGuid", refs={String.class}, tree="[0]")
private Output resourceGuid;
/**
* @return The resource GUID property of the application gateway resource.
*
*/
public Output resourceGuid() {
return this.resourceGuid;
}
/**
* Rewrite rules for the application gateway resource.
*
*/
@Export(name="rewriteRuleSets", refs={List.class,ApplicationGatewayRewriteRuleSetResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> rewriteRuleSets;
/**
* @return Rewrite rules for the application gateway resource.
*
*/
public Output>> rewriteRuleSets() {
return Codegen.optional(this.rewriteRuleSets);
}
/**
* Routing rules of the application gateway resource.
*
*/
@Export(name="routingRules", refs={List.class,ApplicationGatewayRoutingRuleResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> routingRules;
/**
* @return Routing rules of the application gateway resource.
*
*/
public Output>> routingRules() {
return Codegen.optional(this.routingRules);
}
/**
* SKU of the application gateway resource.
*
*/
@Export(name="sku", refs={ApplicationGatewaySkuResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationGatewaySkuResponse> sku;
/**
* @return SKU of the application gateway resource.
*
*/
public Output> sku() {
return Codegen.optional(this.sku);
}
/**
* SSL certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="sslCertificates", refs={List.class,ApplicationGatewaySslCertificateResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> sslCertificates;
/**
* @return SSL certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> sslCertificates() {
return Codegen.optional(this.sslCertificates);
}
/**
* SSL policy of the application gateway resource.
*
*/
@Export(name="sslPolicy", refs={ApplicationGatewaySslPolicyResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationGatewaySslPolicyResponse> sslPolicy;
/**
* @return SSL policy of the application gateway resource.
*
*/
public Output> sslPolicy() {
return Codegen.optional(this.sslPolicy);
}
/**
* SSL profiles of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="sslProfiles", refs={List.class,ApplicationGatewaySslProfileResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> sslProfiles;
/**
* @return SSL profiles of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> sslProfiles() {
return Codegen.optional(this.sslProfiles);
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Trusted client certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="trustedClientCertificates", refs={List.class,ApplicationGatewayTrustedClientCertificateResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> trustedClientCertificates;
/**
* @return Trusted client certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> trustedClientCertificates() {
return Codegen.optional(this.trustedClientCertificates);
}
/**
* Trusted Root certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="trustedRootCertificates", refs={List.class,ApplicationGatewayTrustedRootCertificateResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> trustedRootCertificates;
/**
* @return Trusted Root certificates of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> trustedRootCertificates() {
return Codegen.optional(this.trustedRootCertificates);
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
* URL path map of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
@Export(name="urlPathMaps", refs={List.class,ApplicationGatewayUrlPathMapResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> urlPathMaps;
/**
* @return URL path map of the application gateway resource. For default limits, see [Application Gateway limits](https://docs.microsoft.com/azure/azure-subscription-service-limits#application-gateway-limits).
*
*/
public Output>> urlPathMaps() {
return Codegen.optional(this.urlPathMaps);
}
/**
* Web application firewall configuration.
*
*/
@Export(name="webApplicationFirewallConfiguration", refs={ApplicationGatewayWebApplicationFirewallConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ ApplicationGatewayWebApplicationFirewallConfigurationResponse> webApplicationFirewallConfiguration;
/**
* @return Web application firewall configuration.
*
*/
public Output> webApplicationFirewallConfiguration() {
return Codegen.optional(this.webApplicationFirewallConfiguration);
}
/**
* A list of availability zones denoting where the resource needs to come from.
*
*/
@Export(name="zones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> zones;
/**
* @return A list of availability zones denoting where the resource needs to come from.
*
*/
public Output>> zones() {
return Codegen.optional(this.zones);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ApplicationGateway(java.lang.String name) {
this(name, ApplicationGatewayArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ApplicationGateway(java.lang.String name, ApplicationGatewayArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ApplicationGateway(java.lang.String name, ApplicationGatewayArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:network:ApplicationGateway", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ApplicationGateway(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:network:ApplicationGateway", name, null, makeResourceOptions(options, id), false);
}
private static ApplicationGatewayArgs makeArgs(ApplicationGatewayArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ApplicationGatewayArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:network/v20150501preview:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20150615:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20160330:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20160601:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20160901:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20161201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20170301:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20170601:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20170801:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20170901:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20171001:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20171101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20180101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20180201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20180401:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20180601:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20180701:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20180801:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20181001:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20181101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20181201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20190201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20190401:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20190601:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20190701:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20190801:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20190901:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20191101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20191201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20200301:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20200401:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20200501:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20200601:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20200701:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20200801:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20201101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20210201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20210301:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20210501:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20210801:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20220101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20220501:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20220701:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20220901:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20221101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20230201:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20230401:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20230501:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20230601:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20230901:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20231101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20240101:ApplicationGateway").build()),
Output.of(Alias.builder().type("azure-native:network/v20240301:ApplicationGateway").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ApplicationGateway get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ApplicationGateway(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy