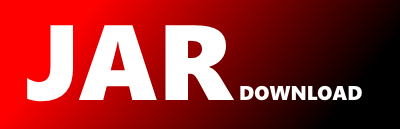
com.pulumi.azurenative.network.CustomIPPrefixArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.CommissionedState;
import com.pulumi.azurenative.network.enums.CustomIpPrefixType;
import com.pulumi.azurenative.network.enums.Geo;
import com.pulumi.azurenative.network.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CustomIPPrefixArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomIPPrefixArgs Empty = new CustomIPPrefixArgs();
/**
* The ASN for CIDR advertising. Should be an integer as string.
*
*/
@Import(name="asn")
private @Nullable Output asn;
/**
* @return The ASN for CIDR advertising. Should be an integer as string.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy