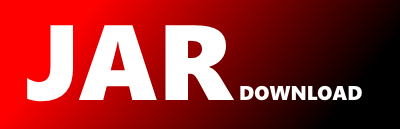
com.pulumi.azurenative.network.PacketCaptureArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.PacketCaptureTargetType;
import com.pulumi.azurenative.network.inputs.PacketCaptureFilterArgs;
import com.pulumi.azurenative.network.inputs.PacketCaptureMachineScopeArgs;
import com.pulumi.azurenative.network.inputs.PacketCaptureStorageLocationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PacketCaptureArgs extends com.pulumi.resources.ResourceArgs {
public static final PacketCaptureArgs Empty = new PacketCaptureArgs();
/**
* Number of bytes captured per packet, the remaining bytes are truncated.
*
*/
@Import(name="bytesToCapturePerPacket")
private @Nullable Output bytesToCapturePerPacket;
/**
* @return Number of bytes captured per packet, the remaining bytes are truncated.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy