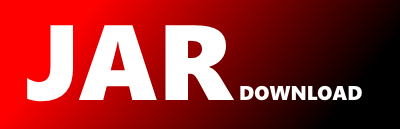
com.pulumi.azurenative.network.PrivateRecordSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.network.PrivateRecordSetArgs;
import com.pulumi.azurenative.network.outputs.ARecordResponse;
import com.pulumi.azurenative.network.outputs.AaaaRecordResponse;
import com.pulumi.azurenative.network.outputs.CnameRecordResponse;
import com.pulumi.azurenative.network.outputs.MxRecordResponse;
import com.pulumi.azurenative.network.outputs.PtrRecordResponse;
import com.pulumi.azurenative.network.outputs.SoaRecordResponse;
import com.pulumi.azurenative.network.outputs.SrvRecordResponse;
import com.pulumi.azurenative.network.outputs.TxtRecordResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a DNS record set (a collection of DNS records with the same name and type) in a Private DNS zone.
* Azure REST API version: 2020-06-01. Prior API version in Azure Native 1.x: 2020-06-01.
*
* Other available API versions: 2024-06-01.
*
* ## Example Usage
* ### PUT Private DNS Zone A Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.ARecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .aRecords(ARecordArgs.builder()
* .ipv4Address("1.2.3.4")
* .build())
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("privatezone1.com")
* .recordType("A")
* .relativeRecordSetName("recordA")
* .resourceGroupName("resourceGroup1")
* .ttl(3600)
* .build());
*
* }
* }
*
* }
*
* ### PUT Private DNS Zone AAAA Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.AaaaRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .aaaaRecords(AaaaRecordArgs.builder()
* .ipv6Address("::1")
* .build())
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("privatezone1.com")
* .recordType("AAAA")
* .relativeRecordSetName("recordAAAA")
* .resourceGroupName("resourceGroup1")
* .ttl(3600)
* .build());
*
* }
* }
*
* }
*
* ### PUT Private DNS Zone CNAME Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.CnameRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .cnameRecord(CnameRecordArgs.builder()
* .cname("contoso.com")
* .build())
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("privatezone1.com")
* .recordType("CNAME")
* .relativeRecordSetName("recordCNAME")
* .resourceGroupName("resourceGroup1")
* .ttl(3600)
* .build());
*
* }
* }
*
* }
*
* ### PUT Private DNS Zone MX Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.MxRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .metadata(Map.of("key1", "value1"))
* .mxRecords(MxRecordArgs.builder()
* .exchange("mail.privatezone1.com")
* .preference(0)
* .build())
* .privateZoneName("privatezone1.com")
* .recordType("MX")
* .relativeRecordSetName("recordMX")
* .resourceGroupName("resourceGroup1")
* .ttl(3600)
* .build());
*
* }
* }
*
* }
*
* ### PUT Private DNS Zone PTR Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.PtrRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("0.0.127.in-addr.arpa")
* .ptrRecords(PtrRecordArgs.builder()
* .ptrdname("localhost")
* .build())
* .recordType("PTR")
* .relativeRecordSetName("1")
* .resourceGroupName("resourceGroup1")
* .ttl(3600)
* .build());
*
* }
* }
*
* }
*
* ### PUT Private DNS Zone SOA Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.SoaRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("privatezone1.com")
* .recordType("SOA")
* .relativeRecordSetName("}{@literal @}{@code ")
* .resourceGroupName("resourceGroup1")
* .soaRecord(SoaRecordArgs.builder()
* .email("azureprivatedns-hostmaster.microsoft.com")
* .expireTime(2419200)
* .host("azureprivatedns.net")
* .minimumTtl(300)
* .refreshTime(3600)
* .retryTime(300)
* .serialNumber(1)
* .build())
* .ttl(3600)
* .build());
*
* }}{@code
* }}{@code
*
* }
*
* ### PUT Private DNS Zone SRV Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.SrvRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("privatezone1.com")
* .recordType("SRV")
* .relativeRecordSetName("recordSRV")
* .resourceGroupName("resourceGroup1")
* .srvRecords(SrvRecordArgs.builder()
* .port(80)
* .priority(0)
* .target("contoso.com")
* .weight(10)
* .build())
* .ttl(3600)
* .build());
*
* }
* }
*
* }
*
* ### PUT Private DNS Zone TXT Record Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.PrivateRecordSet;
* import com.pulumi.azurenative.network.PrivateRecordSetArgs;
* import com.pulumi.azurenative.network.inputs.TxtRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var privateRecordSet = new PrivateRecordSet("privateRecordSet", PrivateRecordSetArgs.builder()
* .metadata(Map.of("key1", "value1"))
* .privateZoneName("privatezone1.com")
* .recordType("TXT")
* .relativeRecordSetName("recordTXT")
* .resourceGroupName("resourceGroup1")
* .ttl(3600)
* .txtRecords(TxtRecordArgs.builder()
* .value(
* "string1",
* "string2")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:network:PrivateRecordSet recordtxt /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/privateDnsZones/{privateZoneName}/{recordType}/{relativeRecordSetName}
* ```
*
*/
@ResourceType(type="azure-native:network:PrivateRecordSet")
public class PrivateRecordSet extends com.pulumi.resources.CustomResource {
/**
* The list of A records in the record set.
*
*/
@Export(name="aRecords", refs={List.class,ARecordResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> aRecords;
/**
* @return The list of A records in the record set.
*
*/
public Output>> aRecords() {
return Codegen.optional(this.aRecords);
}
/**
* The list of AAAA records in the record set.
*
*/
@Export(name="aaaaRecords", refs={List.class,AaaaRecordResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> aaaaRecords;
/**
* @return The list of AAAA records in the record set.
*
*/
public Output>> aaaaRecords() {
return Codegen.optional(this.aaaaRecords);
}
/**
* The CNAME record in the record set.
*
*/
@Export(name="cnameRecord", refs={CnameRecordResponse.class}, tree="[0]")
private Output* @Nullable */ CnameRecordResponse> cnameRecord;
/**
* @return The CNAME record in the record set.
*
*/
public Output> cnameRecord() {
return Codegen.optional(this.cnameRecord);
}
/**
* The ETag of the record set.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> etag;
/**
* @return The ETag of the record set.
*
*/
public Output> etag() {
return Codegen.optional(this.etag);
}
/**
* Fully qualified domain name of the record set.
*
*/
@Export(name="fqdn", refs={String.class}, tree="[0]")
private Output fqdn;
/**
* @return Fully qualified domain name of the record set.
*
*/
public Output fqdn() {
return this.fqdn;
}
/**
* Is the record set auto-registered in the Private DNS zone through a virtual network link?
*
*/
@Export(name="isAutoRegistered", refs={Boolean.class}, tree="[0]")
private Output isAutoRegistered;
/**
* @return Is the record set auto-registered in the Private DNS zone through a virtual network link?
*
*/
public Output isAutoRegistered() {
return this.isAutoRegistered;
}
/**
* The metadata attached to the record set.
*
*/
@Export(name="metadata", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> metadata;
/**
* @return The metadata attached to the record set.
*
*/
public Output>> metadata() {
return Codegen.optional(this.metadata);
}
/**
* The list of MX records in the record set.
*
*/
@Export(name="mxRecords", refs={List.class,MxRecordResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> mxRecords;
/**
* @return The list of MX records in the record set.
*
*/
public Output>> mxRecords() {
return Codegen.optional(this.mxRecords);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The list of PTR records in the record set.
*
*/
@Export(name="ptrRecords", refs={List.class,PtrRecordResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> ptrRecords;
/**
* @return The list of PTR records in the record set.
*
*/
public Output>> ptrRecords() {
return Codegen.optional(this.ptrRecords);
}
/**
* The SOA record in the record set.
*
*/
@Export(name="soaRecord", refs={SoaRecordResponse.class}, tree="[0]")
private Output* @Nullable */ SoaRecordResponse> soaRecord;
/**
* @return The SOA record in the record set.
*
*/
public Output> soaRecord() {
return Codegen.optional(this.soaRecord);
}
/**
* The list of SRV records in the record set.
*
*/
@Export(name="srvRecords", refs={List.class,SrvRecordResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> srvRecords;
/**
* @return The list of SRV records in the record set.
*
*/
public Output>> srvRecords() {
return Codegen.optional(this.srvRecords);
}
/**
* The TTL (time-to-live) of the records in the record set.
*
*/
@Export(name="ttl", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> ttl;
/**
* @return The TTL (time-to-live) of the records in the record set.
*
*/
public Output> ttl() {
return Codegen.optional(this.ttl);
}
/**
* The list of TXT records in the record set.
*
*/
@Export(name="txtRecords", refs={List.class,TxtRecordResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> txtRecords;
/**
* @return The list of TXT records in the record set.
*
*/
public Output>> txtRecords() {
return Codegen.optional(this.txtRecords);
}
/**
* The type of the resource. Example - 'Microsoft.Network/privateDnsZones'.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. Example - 'Microsoft.Network/privateDnsZones'.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public PrivateRecordSet(java.lang.String name) {
this(name, PrivateRecordSetArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public PrivateRecordSet(java.lang.String name, PrivateRecordSetArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public PrivateRecordSet(java.lang.String name, PrivateRecordSetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:network:PrivateRecordSet", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private PrivateRecordSet(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:network:PrivateRecordSet", name, null, makeResourceOptions(options, id), false);
}
private static PrivateRecordSetArgs makeArgs(PrivateRecordSetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? PrivateRecordSetArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:network/v20180901:PrivateRecordSet").build()),
Output.of(Alias.builder().type("azure-native:network/v20200101:PrivateRecordSet").build()),
Output.of(Alias.builder().type("azure-native:network/v20200601:PrivateRecordSet").build()),
Output.of(Alias.builder().type("azure-native:network/v20240601:PrivateRecordSet").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static PrivateRecordSet get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new PrivateRecordSet(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy