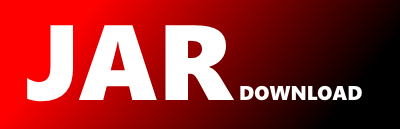
com.pulumi.azurenative.network.VirtualHubArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.HubRoutingPreference;
import com.pulumi.azurenative.network.enums.PreferredRoutingGateway;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.azurenative.network.inputs.VirtualHubRouteTableArgs;
import com.pulumi.azurenative.network.inputs.VirtualHubRouteTableV2Args;
import com.pulumi.azurenative.network.inputs.VirtualRouterAutoScaleConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualHubArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualHubArgs Empty = new VirtualHubArgs();
/**
* Address-prefix for this VirtualHub.
*
*/
@Import(name="addressPrefix")
private @Nullable Output addressPrefix;
/**
* @return Address-prefix for this VirtualHub.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy