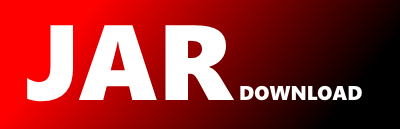
com.pulumi.azurenative.network.VirtualNetworkPeeringArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.VirtualNetworkPeeringLevel;
import com.pulumi.azurenative.network.enums.VirtualNetworkPeeringState;
import com.pulumi.azurenative.network.inputs.AddressSpaceArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkBgpCommunitiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualNetworkPeeringArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualNetworkPeeringArgs Empty = new VirtualNetworkPeeringArgs();
/**
* Whether the forwarded traffic from the VMs in the local virtual network will be allowed/disallowed in remote virtual network.
*
*/
@Import(name="allowForwardedTraffic")
private @Nullable Output allowForwardedTraffic;
/**
* @return Whether the forwarded traffic from the VMs in the local virtual network will be allowed/disallowed in remote virtual network.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy