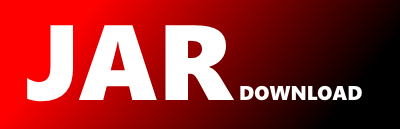
com.pulumi.azurenative.network.VpnConnectionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.VirtualNetworkGatewayConnectionProtocol;
import com.pulumi.azurenative.network.inputs.IpsecPolicyArgs;
import com.pulumi.azurenative.network.inputs.RoutingConfigurationArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.azurenative.network.inputs.TrafficSelectorPolicyArgs;
import com.pulumi.azurenative.network.inputs.VpnSiteLinkConnectionArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VpnConnectionArgs extends com.pulumi.resources.ResourceArgs {
public static final VpnConnectionArgs Empty = new VpnConnectionArgs();
/**
* Expected bandwidth in MBPS.
*
*/
@Import(name="connectionBandwidth")
private @Nullable Output connectionBandwidth;
/**
* @return Expected bandwidth in MBPS.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy