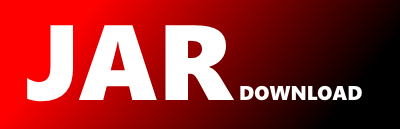
com.pulumi.azurenative.network.inputs.CacheConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.DynamicCompressionEnabled;
import com.pulumi.azurenative.network.enums.FrontDoorQuery;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Caching settings for a caching-type route. To disable caching, do not provide a cacheConfiguration object.
*
*/
public final class CacheConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final CacheConfigurationArgs Empty = new CacheConfigurationArgs();
/**
* The duration for which the content needs to be cached. Allowed format is in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations). HTTP requires the value to be no more than a year
*
*/
@Import(name="cacheDuration")
private @Nullable Output cacheDuration;
/**
* @return The duration for which the content needs to be cached. Allowed format is in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations). HTTP requires the value to be no more than a year
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy