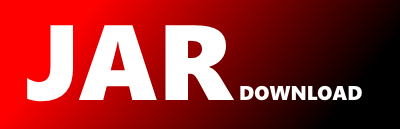
com.pulumi.azurenative.network.inputs.RoutingRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.FrontDoorProtocol;
import com.pulumi.azurenative.network.enums.RoutingRuleEnabledState;
import com.pulumi.azurenative.network.inputs.ForwardingConfigurationArgs;
import com.pulumi.azurenative.network.inputs.RedirectConfigurationArgs;
import com.pulumi.azurenative.network.inputs.RoutingRuleUpdateParametersWebApplicationFirewallPolicyLinkArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A routing rule represents a specification for traffic to treat and where to send it, along with health probe information.
*
*/
public final class RoutingRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final RoutingRuleArgs Empty = new RoutingRuleArgs();
/**
* Protocol schemes to match for this rule
*
*/
@Import(name="acceptedProtocols")
private @Nullable Output>> acceptedProtocols;
/**
* @return Protocol schemes to match for this rule
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy