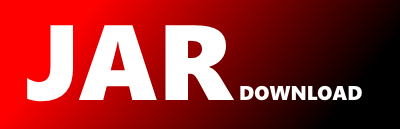
com.pulumi.azurenative.network.outputs.BgpPeerStatusResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class BgpPeerStatusResponse {
/**
* @return The autonomous system number of the remote BGP peer.
*
*/
private Double asn;
/**
* @return For how long the peering has been up.
*
*/
private String connectedDuration;
/**
* @return The virtual network gateway's local address.
*
*/
private String localAddress;
/**
* @return The number of BGP messages received.
*
*/
private Double messagesReceived;
/**
* @return The number of BGP messages sent.
*
*/
private Double messagesSent;
/**
* @return The remote BGP peer.
*
*/
private String neighbor;
/**
* @return The number of routes learned from this peer.
*
*/
private Double routesReceived;
/**
* @return The BGP peer state.
*
*/
private String state;
private BgpPeerStatusResponse() {}
/**
* @return The autonomous system number of the remote BGP peer.
*
*/
public Double asn() {
return this.asn;
}
/**
* @return For how long the peering has been up.
*
*/
public String connectedDuration() {
return this.connectedDuration;
}
/**
* @return The virtual network gateway's local address.
*
*/
public String localAddress() {
return this.localAddress;
}
/**
* @return The number of BGP messages received.
*
*/
public Double messagesReceived() {
return this.messagesReceived;
}
/**
* @return The number of BGP messages sent.
*
*/
public Double messagesSent() {
return this.messagesSent;
}
/**
* @return The remote BGP peer.
*
*/
public String neighbor() {
return this.neighbor;
}
/**
* @return The number of routes learned from this peer.
*
*/
public Double routesReceived() {
return this.routesReceived;
}
/**
* @return The BGP peer state.
*
*/
public String state() {
return this.state;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BgpPeerStatusResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double asn;
private String connectedDuration;
private String localAddress;
private Double messagesReceived;
private Double messagesSent;
private String neighbor;
private Double routesReceived;
private String state;
public Builder() {}
public Builder(BgpPeerStatusResponse defaults) {
Objects.requireNonNull(defaults);
this.asn = defaults.asn;
this.connectedDuration = defaults.connectedDuration;
this.localAddress = defaults.localAddress;
this.messagesReceived = defaults.messagesReceived;
this.messagesSent = defaults.messagesSent;
this.neighbor = defaults.neighbor;
this.routesReceived = defaults.routesReceived;
this.state = defaults.state;
}
@CustomType.Setter
public Builder asn(Double asn) {
if (asn == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "asn");
}
this.asn = asn;
return this;
}
@CustomType.Setter
public Builder connectedDuration(String connectedDuration) {
if (connectedDuration == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "connectedDuration");
}
this.connectedDuration = connectedDuration;
return this;
}
@CustomType.Setter
public Builder localAddress(String localAddress) {
if (localAddress == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "localAddress");
}
this.localAddress = localAddress;
return this;
}
@CustomType.Setter
public Builder messagesReceived(Double messagesReceived) {
if (messagesReceived == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "messagesReceived");
}
this.messagesReceived = messagesReceived;
return this;
}
@CustomType.Setter
public Builder messagesSent(Double messagesSent) {
if (messagesSent == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "messagesSent");
}
this.messagesSent = messagesSent;
return this;
}
@CustomType.Setter
public Builder neighbor(String neighbor) {
if (neighbor == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "neighbor");
}
this.neighbor = neighbor;
return this;
}
@CustomType.Setter
public Builder routesReceived(Double routesReceived) {
if (routesReceived == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "routesReceived");
}
this.routesReceived = routesReceived;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("BgpPeerStatusResponse", "state");
}
this.state = state;
return this;
}
public BgpPeerStatusResponse build() {
final var _resultValue = new BgpPeerStatusResponse();
_resultValue.asn = asn;
_resultValue.connectedDuration = connectedDuration;
_resultValue.localAddress = localAddress;
_resultValue.messagesReceived = messagesReceived;
_resultValue.messagesSent = messagesSent;
_resultValue.neighbor = neighbor;
_resultValue.routesReceived = routesReceived;
_resultValue.state = state;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy