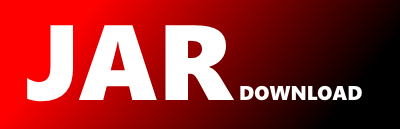
com.pulumi.azurenative.network.outputs.ExpressRouteLinkResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.ExpressRouteLinkMacSecConfigResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ExpressRouteLinkResponse {
/**
* @return Administrative state of the physical port.
*
*/
private @Nullable String adminState;
/**
* @return Cololocation for ExpressRoute Hybrid Direct.
*
*/
private String coloLocation;
/**
* @return Physical fiber port type.
*
*/
private String connectorType;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Name of Azure router interface.
*
*/
private String interfaceName;
/**
* @return MacSec configuration.
*
*/
private @Nullable ExpressRouteLinkMacSecConfigResponse macSecConfig;
/**
* @return Name of child port resource that is unique among child port resources of the parent.
*
*/
private @Nullable String name;
/**
* @return Mapping between physical port to patch panel port.
*
*/
private String patchPanelId;
/**
* @return The provisioning state of the express route link resource.
*
*/
private String provisioningState;
/**
* @return Mapping of physical patch panel to rack.
*
*/
private String rackId;
/**
* @return Name of Azure router associated with physical port.
*
*/
private String routerName;
private ExpressRouteLinkResponse() {}
/**
* @return Administrative state of the physical port.
*
*/
public Optional adminState() {
return Optional.ofNullable(this.adminState);
}
/**
* @return Cololocation for ExpressRoute Hybrid Direct.
*
*/
public String coloLocation() {
return this.coloLocation;
}
/**
* @return Physical fiber port type.
*
*/
public String connectorType() {
return this.connectorType;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Name of Azure router interface.
*
*/
public String interfaceName() {
return this.interfaceName;
}
/**
* @return MacSec configuration.
*
*/
public Optional macSecConfig() {
return Optional.ofNullable(this.macSecConfig);
}
/**
* @return Name of child port resource that is unique among child port resources of the parent.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Mapping between physical port to patch panel port.
*
*/
public String patchPanelId() {
return this.patchPanelId;
}
/**
* @return The provisioning state of the express route link resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Mapping of physical patch panel to rack.
*
*/
public String rackId() {
return this.rackId;
}
/**
* @return Name of Azure router associated with physical port.
*
*/
public String routerName() {
return this.routerName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ExpressRouteLinkResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String adminState;
private String coloLocation;
private String connectorType;
private String etag;
private @Nullable String id;
private String interfaceName;
private @Nullable ExpressRouteLinkMacSecConfigResponse macSecConfig;
private @Nullable String name;
private String patchPanelId;
private String provisioningState;
private String rackId;
private String routerName;
public Builder() {}
public Builder(ExpressRouteLinkResponse defaults) {
Objects.requireNonNull(defaults);
this.adminState = defaults.adminState;
this.coloLocation = defaults.coloLocation;
this.connectorType = defaults.connectorType;
this.etag = defaults.etag;
this.id = defaults.id;
this.interfaceName = defaults.interfaceName;
this.macSecConfig = defaults.macSecConfig;
this.name = defaults.name;
this.patchPanelId = defaults.patchPanelId;
this.provisioningState = defaults.provisioningState;
this.rackId = defaults.rackId;
this.routerName = defaults.routerName;
}
@CustomType.Setter
public Builder adminState(@Nullable String adminState) {
this.adminState = adminState;
return this;
}
@CustomType.Setter
public Builder coloLocation(String coloLocation) {
if (coloLocation == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "coloLocation");
}
this.coloLocation = coloLocation;
return this;
}
@CustomType.Setter
public Builder connectorType(String connectorType) {
if (connectorType == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "connectorType");
}
this.connectorType = connectorType;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder interfaceName(String interfaceName) {
if (interfaceName == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "interfaceName");
}
this.interfaceName = interfaceName;
return this;
}
@CustomType.Setter
public Builder macSecConfig(@Nullable ExpressRouteLinkMacSecConfigResponse macSecConfig) {
this.macSecConfig = macSecConfig;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder patchPanelId(String patchPanelId) {
if (patchPanelId == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "patchPanelId");
}
this.patchPanelId = patchPanelId;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder rackId(String rackId) {
if (rackId == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "rackId");
}
this.rackId = rackId;
return this;
}
@CustomType.Setter
public Builder routerName(String routerName) {
if (routerName == null) {
throw new MissingRequiredPropertyException("ExpressRouteLinkResponse", "routerName");
}
this.routerName = routerName;
return this;
}
public ExpressRouteLinkResponse build() {
final var _resultValue = new ExpressRouteLinkResponse();
_resultValue.adminState = adminState;
_resultValue.coloLocation = coloLocation;
_resultValue.connectorType = connectorType;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.interfaceName = interfaceName;
_resultValue.macSecConfig = macSecConfig;
_resultValue.name = name;
_resultValue.patchPanelId = patchPanelId;
_resultValue.provisioningState = provisioningState;
_resultValue.rackId = rackId;
_resultValue.routerName = routerName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy