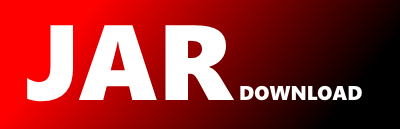
com.pulumi.azurenative.network.outputs.GatewayRouteResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GatewayRouteResponse {
/**
* @return The route's AS path sequence.
*
*/
private String asPath;
/**
* @return The gateway's local address.
*
*/
private String localAddress;
/**
* @return The route's network prefix.
*
*/
private String network;
/**
* @return The route's next hop.
*
*/
private String nextHop;
/**
* @return The source this route was learned from.
*
*/
private String origin;
/**
* @return The peer this route was learned from.
*
*/
private String sourcePeer;
/**
* @return The route's weight.
*
*/
private Integer weight;
private GatewayRouteResponse() {}
/**
* @return The route's AS path sequence.
*
*/
public String asPath() {
return this.asPath;
}
/**
* @return The gateway's local address.
*
*/
public String localAddress() {
return this.localAddress;
}
/**
* @return The route's network prefix.
*
*/
public String network() {
return this.network;
}
/**
* @return The route's next hop.
*
*/
public String nextHop() {
return this.nextHop;
}
/**
* @return The source this route was learned from.
*
*/
public String origin() {
return this.origin;
}
/**
* @return The peer this route was learned from.
*
*/
public String sourcePeer() {
return this.sourcePeer;
}
/**
* @return The route's weight.
*
*/
public Integer weight() {
return this.weight;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GatewayRouteResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String asPath;
private String localAddress;
private String network;
private String nextHop;
private String origin;
private String sourcePeer;
private Integer weight;
public Builder() {}
public Builder(GatewayRouteResponse defaults) {
Objects.requireNonNull(defaults);
this.asPath = defaults.asPath;
this.localAddress = defaults.localAddress;
this.network = defaults.network;
this.nextHop = defaults.nextHop;
this.origin = defaults.origin;
this.sourcePeer = defaults.sourcePeer;
this.weight = defaults.weight;
}
@CustomType.Setter
public Builder asPath(String asPath) {
if (asPath == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "asPath");
}
this.asPath = asPath;
return this;
}
@CustomType.Setter
public Builder localAddress(String localAddress) {
if (localAddress == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "localAddress");
}
this.localAddress = localAddress;
return this;
}
@CustomType.Setter
public Builder network(String network) {
if (network == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "network");
}
this.network = network;
return this;
}
@CustomType.Setter
public Builder nextHop(String nextHop) {
if (nextHop == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "nextHop");
}
this.nextHop = nextHop;
return this;
}
@CustomType.Setter
public Builder origin(String origin) {
if (origin == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "origin");
}
this.origin = origin;
return this;
}
@CustomType.Setter
public Builder sourcePeer(String sourcePeer) {
if (sourcePeer == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "sourcePeer");
}
this.sourcePeer = sourcePeer;
return this;
}
@CustomType.Setter
public Builder weight(Integer weight) {
if (weight == null) {
throw new MissingRequiredPropertyException("GatewayRouteResponse", "weight");
}
this.weight = weight;
return this;
}
public GatewayRouteResponse build() {
final var _resultValue = new GatewayRouteResponse();
_resultValue.asPath = asPath;
_resultValue.localAddress = localAddress;
_resultValue.network = network;
_resultValue.nextHop = nextHop;
_resultValue.origin = origin;
_resultValue.sourcePeer = sourcePeer;
_resultValue.weight = weight;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy