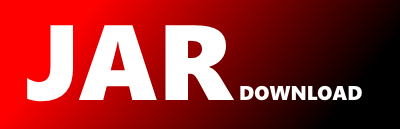
com.pulumi.azurenative.network.outputs.GetDdosCustomPolicyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDdosCustomPolicyResult {
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The provisioning state of the DDoS custom policy resource.
*
*/
private String provisioningState;
/**
* @return The resource GUID property of the DDoS custom policy resource. It uniquely identifies the resource, even if the user changes its name or migrate the resource across subscriptions or resource groups.
*
*/
private String resourceGuid;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
private GetDdosCustomPolicyResult() {}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state of the DDoS custom policy resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The resource GUID property of the DDoS custom policy resource. It uniquely identifies the resource, even if the user changes its name or migrate the resource across subscriptions or resource groups.
*
*/
public String resourceGuid() {
return this.resourceGuid;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDdosCustomPolicyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String etag;
private @Nullable String id;
private @Nullable String location;
private String name;
private String provisioningState;
private String resourceGuid;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetDdosCustomPolicyResult defaults) {
Objects.requireNonNull(defaults);
this.etag = defaults.etag;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.resourceGuid = defaults.resourceGuid;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetDdosCustomPolicyResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDdosCustomPolicyResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDdosCustomPolicyResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceGuid(String resourceGuid) {
if (resourceGuid == null) {
throw new MissingRequiredPropertyException("GetDdosCustomPolicyResult", "resourceGuid");
}
this.resourceGuid = resourceGuid;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDdosCustomPolicyResult", "type");
}
this.type = type;
return this;
}
public GetDdosCustomPolicyResult build() {
final var _resultValue = new GetDdosCustomPolicyResult();
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceGuid = resourceGuid;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy