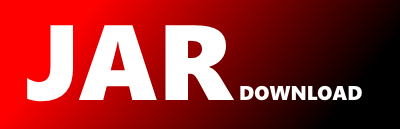
com.pulumi.azurenative.network.outputs.GetExpressRouteConnectionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.ExpressRouteCircuitPeeringIdResponse;
import com.pulumi.azurenative.network.outputs.RoutingConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetExpressRouteConnectionResult {
/**
* @return Authorization key to establish the connection.
*
*/
private @Nullable String authorizationKey;
/**
* @return Enable internet security.
*
*/
private @Nullable Boolean enableInternetSecurity;
/**
* @return Bypass the ExpressRoute gateway when accessing private-links. ExpressRoute FastPath (expressRouteGatewayBypass) must be enabled.
*
*/
private @Nullable Boolean enablePrivateLinkFastPath;
/**
* @return The ExpressRoute circuit peering.
*
*/
private ExpressRouteCircuitPeeringIdResponse expressRouteCircuitPeering;
/**
* @return Enable FastPath to vWan Firewall hub.
*
*/
private @Nullable Boolean expressRouteGatewayBypass;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return The provisioning state of the express route connection resource.
*
*/
private String provisioningState;
/**
* @return The Routing Configuration indicating the associated and propagated route tables on this connection.
*
*/
private @Nullable RoutingConfigurationResponse routingConfiguration;
/**
* @return The routing weight associated to the connection.
*
*/
private @Nullable Integer routingWeight;
private GetExpressRouteConnectionResult() {}
/**
* @return Authorization key to establish the connection.
*
*/
public Optional authorizationKey() {
return Optional.ofNullable(this.authorizationKey);
}
/**
* @return Enable internet security.
*
*/
public Optional enableInternetSecurity() {
return Optional.ofNullable(this.enableInternetSecurity);
}
/**
* @return Bypass the ExpressRoute gateway when accessing private-links. ExpressRoute FastPath (expressRouteGatewayBypass) must be enabled.
*
*/
public Optional enablePrivateLinkFastPath() {
return Optional.ofNullable(this.enablePrivateLinkFastPath);
}
/**
* @return The ExpressRoute circuit peering.
*
*/
public ExpressRouteCircuitPeeringIdResponse expressRouteCircuitPeering() {
return this.expressRouteCircuitPeering;
}
/**
* @return Enable FastPath to vWan Firewall hub.
*
*/
public Optional expressRouteGatewayBypass() {
return Optional.ofNullable(this.expressRouteGatewayBypass);
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state of the express route connection resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The Routing Configuration indicating the associated and propagated route tables on this connection.
*
*/
public Optional routingConfiguration() {
return Optional.ofNullable(this.routingConfiguration);
}
/**
* @return The routing weight associated to the connection.
*
*/
public Optional routingWeight() {
return Optional.ofNullable(this.routingWeight);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetExpressRouteConnectionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String authorizationKey;
private @Nullable Boolean enableInternetSecurity;
private @Nullable Boolean enablePrivateLinkFastPath;
private ExpressRouteCircuitPeeringIdResponse expressRouteCircuitPeering;
private @Nullable Boolean expressRouteGatewayBypass;
private @Nullable String id;
private String name;
private String provisioningState;
private @Nullable RoutingConfigurationResponse routingConfiguration;
private @Nullable Integer routingWeight;
public Builder() {}
public Builder(GetExpressRouteConnectionResult defaults) {
Objects.requireNonNull(defaults);
this.authorizationKey = defaults.authorizationKey;
this.enableInternetSecurity = defaults.enableInternetSecurity;
this.enablePrivateLinkFastPath = defaults.enablePrivateLinkFastPath;
this.expressRouteCircuitPeering = defaults.expressRouteCircuitPeering;
this.expressRouteGatewayBypass = defaults.expressRouteGatewayBypass;
this.id = defaults.id;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.routingConfiguration = defaults.routingConfiguration;
this.routingWeight = defaults.routingWeight;
}
@CustomType.Setter
public Builder authorizationKey(@Nullable String authorizationKey) {
this.authorizationKey = authorizationKey;
return this;
}
@CustomType.Setter
public Builder enableInternetSecurity(@Nullable Boolean enableInternetSecurity) {
this.enableInternetSecurity = enableInternetSecurity;
return this;
}
@CustomType.Setter
public Builder enablePrivateLinkFastPath(@Nullable Boolean enablePrivateLinkFastPath) {
this.enablePrivateLinkFastPath = enablePrivateLinkFastPath;
return this;
}
@CustomType.Setter
public Builder expressRouteCircuitPeering(ExpressRouteCircuitPeeringIdResponse expressRouteCircuitPeering) {
if (expressRouteCircuitPeering == null) {
throw new MissingRequiredPropertyException("GetExpressRouteConnectionResult", "expressRouteCircuitPeering");
}
this.expressRouteCircuitPeering = expressRouteCircuitPeering;
return this;
}
@CustomType.Setter
public Builder expressRouteGatewayBypass(@Nullable Boolean expressRouteGatewayBypass) {
this.expressRouteGatewayBypass = expressRouteGatewayBypass;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetExpressRouteConnectionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetExpressRouteConnectionResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder routingConfiguration(@Nullable RoutingConfigurationResponse routingConfiguration) {
this.routingConfiguration = routingConfiguration;
return this;
}
@CustomType.Setter
public Builder routingWeight(@Nullable Integer routingWeight) {
this.routingWeight = routingWeight;
return this;
}
public GetExpressRouteConnectionResult build() {
final var _resultValue = new GetExpressRouteConnectionResult();
_resultValue.authorizationKey = authorizationKey;
_resultValue.enableInternetSecurity = enableInternetSecurity;
_resultValue.enablePrivateLinkFastPath = enablePrivateLinkFastPath;
_resultValue.expressRouteCircuitPeering = expressRouteCircuitPeering;
_resultValue.expressRouteGatewayBypass = expressRouteGatewayBypass;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.routingConfiguration = routingConfiguration;
_resultValue.routingWeight = routingWeight;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy