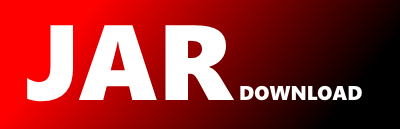
com.pulumi.azurenative.network.outputs.GetP2sVpnGatewayP2sVpnConnectionHealthResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.P2SConnectionConfigurationResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.azurenative.network.outputs.VpnClientConnectionHealthResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetP2sVpnGatewayP2sVpnConnectionHealthResult {
/**
* @return List of all customer specified DNS servers IP addresses.
*
*/
private @Nullable List customDnsServers;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Enable Routing Preference property for the Public IP Interface of the P2SVpnGateway.
*
*/
private @Nullable Boolean isRoutingPreferenceInternet;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return List of all p2s connection configurations of the gateway.
*
*/
private @Nullable List p2SConnectionConfigurations;
/**
* @return The provisioning state of the P2S VPN gateway resource.
*
*/
private String provisioningState;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return The VirtualHub to which the gateway belongs.
*
*/
private @Nullable SubResourceResponse virtualHub;
/**
* @return All P2S VPN clients' connection health status.
*
*/
private VpnClientConnectionHealthResponse vpnClientConnectionHealth;
/**
* @return The scale unit for this p2s vpn gateway.
*
*/
private @Nullable Integer vpnGatewayScaleUnit;
/**
* @return The VpnServerConfiguration to which the p2sVpnGateway is attached to.
*
*/
private @Nullable SubResourceResponse vpnServerConfiguration;
private GetP2sVpnGatewayP2sVpnConnectionHealthResult() {}
/**
* @return List of all customer specified DNS servers IP addresses.
*
*/
public List customDnsServers() {
return this.customDnsServers == null ? List.of() : this.customDnsServers;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Enable Routing Preference property for the Public IP Interface of the P2SVpnGateway.
*
*/
public Optional isRoutingPreferenceInternet() {
return Optional.ofNullable(this.isRoutingPreferenceInternet);
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return List of all p2s connection configurations of the gateway.
*
*/
public List p2SConnectionConfigurations() {
return this.p2SConnectionConfigurations == null ? List.of() : this.p2SConnectionConfigurations;
}
/**
* @return The provisioning state of the P2S VPN gateway resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The VirtualHub to which the gateway belongs.
*
*/
public Optional virtualHub() {
return Optional.ofNullable(this.virtualHub);
}
/**
* @return All P2S VPN clients' connection health status.
*
*/
public VpnClientConnectionHealthResponse vpnClientConnectionHealth() {
return this.vpnClientConnectionHealth;
}
/**
* @return The scale unit for this p2s vpn gateway.
*
*/
public Optional vpnGatewayScaleUnit() {
return Optional.ofNullable(this.vpnGatewayScaleUnit);
}
/**
* @return The VpnServerConfiguration to which the p2sVpnGateway is attached to.
*
*/
public Optional vpnServerConfiguration() {
return Optional.ofNullable(this.vpnServerConfiguration);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetP2sVpnGatewayP2sVpnConnectionHealthResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List customDnsServers;
private String etag;
private @Nullable String id;
private @Nullable Boolean isRoutingPreferenceInternet;
private String location;
private String name;
private @Nullable List p2SConnectionConfigurations;
private String provisioningState;
private @Nullable Map tags;
private String type;
private @Nullable SubResourceResponse virtualHub;
private VpnClientConnectionHealthResponse vpnClientConnectionHealth;
private @Nullable Integer vpnGatewayScaleUnit;
private @Nullable SubResourceResponse vpnServerConfiguration;
public Builder() {}
public Builder(GetP2sVpnGatewayP2sVpnConnectionHealthResult defaults) {
Objects.requireNonNull(defaults);
this.customDnsServers = defaults.customDnsServers;
this.etag = defaults.etag;
this.id = defaults.id;
this.isRoutingPreferenceInternet = defaults.isRoutingPreferenceInternet;
this.location = defaults.location;
this.name = defaults.name;
this.p2SConnectionConfigurations = defaults.p2SConnectionConfigurations;
this.provisioningState = defaults.provisioningState;
this.tags = defaults.tags;
this.type = defaults.type;
this.virtualHub = defaults.virtualHub;
this.vpnClientConnectionHealth = defaults.vpnClientConnectionHealth;
this.vpnGatewayScaleUnit = defaults.vpnGatewayScaleUnit;
this.vpnServerConfiguration = defaults.vpnServerConfiguration;
}
@CustomType.Setter
public Builder customDnsServers(@Nullable List customDnsServers) {
this.customDnsServers = customDnsServers;
return this;
}
public Builder customDnsServers(String... customDnsServers) {
return customDnsServers(List.of(customDnsServers));
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetP2sVpnGatewayP2sVpnConnectionHealthResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder isRoutingPreferenceInternet(@Nullable Boolean isRoutingPreferenceInternet) {
this.isRoutingPreferenceInternet = isRoutingPreferenceInternet;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetP2sVpnGatewayP2sVpnConnectionHealthResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetP2sVpnGatewayP2sVpnConnectionHealthResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder p2SConnectionConfigurations(@Nullable List p2SConnectionConfigurations) {
this.p2SConnectionConfigurations = p2SConnectionConfigurations;
return this;
}
public Builder p2SConnectionConfigurations(P2SConnectionConfigurationResponse... p2SConnectionConfigurations) {
return p2SConnectionConfigurations(List.of(p2SConnectionConfigurations));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetP2sVpnGatewayP2sVpnConnectionHealthResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetP2sVpnGatewayP2sVpnConnectionHealthResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualHub(@Nullable SubResourceResponse virtualHub) {
this.virtualHub = virtualHub;
return this;
}
@CustomType.Setter
public Builder vpnClientConnectionHealth(VpnClientConnectionHealthResponse vpnClientConnectionHealth) {
if (vpnClientConnectionHealth == null) {
throw new MissingRequiredPropertyException("GetP2sVpnGatewayP2sVpnConnectionHealthResult", "vpnClientConnectionHealth");
}
this.vpnClientConnectionHealth = vpnClientConnectionHealth;
return this;
}
@CustomType.Setter
public Builder vpnGatewayScaleUnit(@Nullable Integer vpnGatewayScaleUnit) {
this.vpnGatewayScaleUnit = vpnGatewayScaleUnit;
return this;
}
@CustomType.Setter
public Builder vpnServerConfiguration(@Nullable SubResourceResponse vpnServerConfiguration) {
this.vpnServerConfiguration = vpnServerConfiguration;
return this;
}
public GetP2sVpnGatewayP2sVpnConnectionHealthResult build() {
final var _resultValue = new GetP2sVpnGatewayP2sVpnConnectionHealthResult();
_resultValue.customDnsServers = customDnsServers;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.isRoutingPreferenceInternet = isRoutingPreferenceInternet;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.p2SConnectionConfigurations = p2SConnectionConfigurations;
_resultValue.provisioningState = provisioningState;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.virtualHub = virtualHub;
_resultValue.vpnClientConnectionHealth = vpnClientConnectionHealth;
_resultValue.vpnGatewayScaleUnit = vpnGatewayScaleUnit;
_resultValue.vpnServerConfiguration = vpnServerConfiguration;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy