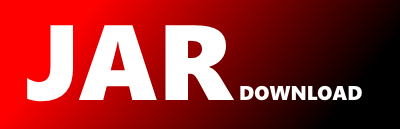
com.pulumi.azurenative.network.outputs.GetSubnetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.ApplicationGatewayIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.DelegationResponse;
import com.pulumi.azurenative.network.outputs.IPConfigurationProfileResponse;
import com.pulumi.azurenative.network.outputs.IPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.NetworkSecurityGroupResponse;
import com.pulumi.azurenative.network.outputs.PrivateEndpointResponse;
import com.pulumi.azurenative.network.outputs.ResourceNavigationLinkResponse;
import com.pulumi.azurenative.network.outputs.RouteTableResponse;
import com.pulumi.azurenative.network.outputs.ServiceAssociationLinkResponse;
import com.pulumi.azurenative.network.outputs.ServiceEndpointPolicyResponse;
import com.pulumi.azurenative.network.outputs.ServiceEndpointPropertiesFormatResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetSubnetResult {
/**
* @return The address prefix for the subnet.
*
*/
private @Nullable String addressPrefix;
/**
* @return List of address prefixes for the subnet.
*
*/
private @Nullable List addressPrefixes;
/**
* @return Application gateway IP configurations of virtual network resource.
*
*/
private @Nullable List applicationGatewayIPConfigurations;
/**
* @return An array of references to the delegations on the subnet.
*
*/
private @Nullable List delegations;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Array of IpAllocation which reference this subnet.
*
*/
private @Nullable List ipAllocations;
/**
* @return Array of IP configuration profiles which reference this subnet.
*
*/
private List ipConfigurationProfiles;
/**
* @return An array of references to the network interface IP configurations using subnet.
*
*/
private List ipConfigurations;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return Nat gateway associated with this subnet.
*
*/
private @Nullable SubResourceResponse natGateway;
/**
* @return The reference to the NetworkSecurityGroup resource.
*
*/
private @Nullable NetworkSecurityGroupResponse networkSecurityGroup;
/**
* @return Enable or Disable apply network policies on private end point in the subnet.
*
*/
private @Nullable String privateEndpointNetworkPolicies;
/**
* @return An array of references to private endpoints.
*
*/
private List privateEndpoints;
/**
* @return Enable or Disable apply network policies on private link service in the subnet.
*
*/
private @Nullable String privateLinkServiceNetworkPolicies;
/**
* @return The provisioning state of the subnet resource.
*
*/
private String provisioningState;
/**
* @return A read-only string identifying the intention of use for this subnet based on delegations and other user-defined properties.
*
*/
private String purpose;
/**
* @return An array of references to the external resources using subnet.
*
*/
private List resourceNavigationLinks;
/**
* @return The reference to the RouteTable resource.
*
*/
private @Nullable RouteTableResponse routeTable;
/**
* @return An array of references to services injecting into this subnet.
*
*/
private List serviceAssociationLinks;
/**
* @return An array of service endpoint policies.
*
*/
private @Nullable List serviceEndpointPolicies;
/**
* @return An array of service endpoints.
*
*/
private @Nullable List serviceEndpoints;
/**
* @return Resource type.
*
*/
private @Nullable String type;
private GetSubnetResult() {}
/**
* @return The address prefix for the subnet.
*
*/
public Optional addressPrefix() {
return Optional.ofNullable(this.addressPrefix);
}
/**
* @return List of address prefixes for the subnet.
*
*/
public List addressPrefixes() {
return this.addressPrefixes == null ? List.of() : this.addressPrefixes;
}
/**
* @return Application gateway IP configurations of virtual network resource.
*
*/
public List applicationGatewayIPConfigurations() {
return this.applicationGatewayIPConfigurations == null ? List.of() : this.applicationGatewayIPConfigurations;
}
/**
* @return An array of references to the delegations on the subnet.
*
*/
public List delegations() {
return this.delegations == null ? List.of() : this.delegations;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Array of IpAllocation which reference this subnet.
*
*/
public List ipAllocations() {
return this.ipAllocations == null ? List.of() : this.ipAllocations;
}
/**
* @return Array of IP configuration profiles which reference this subnet.
*
*/
public List ipConfigurationProfiles() {
return this.ipConfigurationProfiles;
}
/**
* @return An array of references to the network interface IP configurations using subnet.
*
*/
public List ipConfigurations() {
return this.ipConfigurations;
}
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Nat gateway associated with this subnet.
*
*/
public Optional natGateway() {
return Optional.ofNullable(this.natGateway);
}
/**
* @return The reference to the NetworkSecurityGroup resource.
*
*/
public Optional networkSecurityGroup() {
return Optional.ofNullable(this.networkSecurityGroup);
}
/**
* @return Enable or Disable apply network policies on private end point in the subnet.
*
*/
public Optional privateEndpointNetworkPolicies() {
return Optional.ofNullable(this.privateEndpointNetworkPolicies);
}
/**
* @return An array of references to private endpoints.
*
*/
public List privateEndpoints() {
return this.privateEndpoints;
}
/**
* @return Enable or Disable apply network policies on private link service in the subnet.
*
*/
public Optional privateLinkServiceNetworkPolicies() {
return Optional.ofNullable(this.privateLinkServiceNetworkPolicies);
}
/**
* @return The provisioning state of the subnet resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return A read-only string identifying the intention of use for this subnet based on delegations and other user-defined properties.
*
*/
public String purpose() {
return this.purpose;
}
/**
* @return An array of references to the external resources using subnet.
*
*/
public List resourceNavigationLinks() {
return this.resourceNavigationLinks;
}
/**
* @return The reference to the RouteTable resource.
*
*/
public Optional routeTable() {
return Optional.ofNullable(this.routeTable);
}
/**
* @return An array of references to services injecting into this subnet.
*
*/
public List serviceAssociationLinks() {
return this.serviceAssociationLinks;
}
/**
* @return An array of service endpoint policies.
*
*/
public List serviceEndpointPolicies() {
return this.serviceEndpointPolicies == null ? List.of() : this.serviceEndpointPolicies;
}
/**
* @return An array of service endpoints.
*
*/
public List serviceEndpoints() {
return this.serviceEndpoints == null ? List.of() : this.serviceEndpoints;
}
/**
* @return Resource type.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetSubnetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String addressPrefix;
private @Nullable List addressPrefixes;
private @Nullable List applicationGatewayIPConfigurations;
private @Nullable List delegations;
private String etag;
private @Nullable String id;
private @Nullable List ipAllocations;
private List ipConfigurationProfiles;
private List ipConfigurations;
private @Nullable String name;
private @Nullable SubResourceResponse natGateway;
private @Nullable NetworkSecurityGroupResponse networkSecurityGroup;
private @Nullable String privateEndpointNetworkPolicies;
private List privateEndpoints;
private @Nullable String privateLinkServiceNetworkPolicies;
private String provisioningState;
private String purpose;
private List resourceNavigationLinks;
private @Nullable RouteTableResponse routeTable;
private List serviceAssociationLinks;
private @Nullable List serviceEndpointPolicies;
private @Nullable List serviceEndpoints;
private @Nullable String type;
public Builder() {}
public Builder(GetSubnetResult defaults) {
Objects.requireNonNull(defaults);
this.addressPrefix = defaults.addressPrefix;
this.addressPrefixes = defaults.addressPrefixes;
this.applicationGatewayIPConfigurations = defaults.applicationGatewayIPConfigurations;
this.delegations = defaults.delegations;
this.etag = defaults.etag;
this.id = defaults.id;
this.ipAllocations = defaults.ipAllocations;
this.ipConfigurationProfiles = defaults.ipConfigurationProfiles;
this.ipConfigurations = defaults.ipConfigurations;
this.name = defaults.name;
this.natGateway = defaults.natGateway;
this.networkSecurityGroup = defaults.networkSecurityGroup;
this.privateEndpointNetworkPolicies = defaults.privateEndpointNetworkPolicies;
this.privateEndpoints = defaults.privateEndpoints;
this.privateLinkServiceNetworkPolicies = defaults.privateLinkServiceNetworkPolicies;
this.provisioningState = defaults.provisioningState;
this.purpose = defaults.purpose;
this.resourceNavigationLinks = defaults.resourceNavigationLinks;
this.routeTable = defaults.routeTable;
this.serviceAssociationLinks = defaults.serviceAssociationLinks;
this.serviceEndpointPolicies = defaults.serviceEndpointPolicies;
this.serviceEndpoints = defaults.serviceEndpoints;
this.type = defaults.type;
}
@CustomType.Setter
public Builder addressPrefix(@Nullable String addressPrefix) {
this.addressPrefix = addressPrefix;
return this;
}
@CustomType.Setter
public Builder addressPrefixes(@Nullable List addressPrefixes) {
this.addressPrefixes = addressPrefixes;
return this;
}
public Builder addressPrefixes(String... addressPrefixes) {
return addressPrefixes(List.of(addressPrefixes));
}
@CustomType.Setter
public Builder applicationGatewayIPConfigurations(@Nullable List applicationGatewayIPConfigurations) {
this.applicationGatewayIPConfigurations = applicationGatewayIPConfigurations;
return this;
}
public Builder applicationGatewayIPConfigurations(ApplicationGatewayIPConfigurationResponse... applicationGatewayIPConfigurations) {
return applicationGatewayIPConfigurations(List.of(applicationGatewayIPConfigurations));
}
@CustomType.Setter
public Builder delegations(@Nullable List delegations) {
this.delegations = delegations;
return this;
}
public Builder delegations(DelegationResponse... delegations) {
return delegations(List.of(delegations));
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipAllocations(@Nullable List ipAllocations) {
this.ipAllocations = ipAllocations;
return this;
}
public Builder ipAllocations(SubResourceResponse... ipAllocations) {
return ipAllocations(List.of(ipAllocations));
}
@CustomType.Setter
public Builder ipConfigurationProfiles(List ipConfigurationProfiles) {
if (ipConfigurationProfiles == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "ipConfigurationProfiles");
}
this.ipConfigurationProfiles = ipConfigurationProfiles;
return this;
}
public Builder ipConfigurationProfiles(IPConfigurationProfileResponse... ipConfigurationProfiles) {
return ipConfigurationProfiles(List.of(ipConfigurationProfiles));
}
@CustomType.Setter
public Builder ipConfigurations(List ipConfigurations) {
if (ipConfigurations == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "ipConfigurations");
}
this.ipConfigurations = ipConfigurations;
return this;
}
public Builder ipConfigurations(IPConfigurationResponse... ipConfigurations) {
return ipConfigurations(List.of(ipConfigurations));
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder natGateway(@Nullable SubResourceResponse natGateway) {
this.natGateway = natGateway;
return this;
}
@CustomType.Setter
public Builder networkSecurityGroup(@Nullable NetworkSecurityGroupResponse networkSecurityGroup) {
this.networkSecurityGroup = networkSecurityGroup;
return this;
}
@CustomType.Setter
public Builder privateEndpointNetworkPolicies(@Nullable String privateEndpointNetworkPolicies) {
this.privateEndpointNetworkPolicies = privateEndpointNetworkPolicies;
return this;
}
@CustomType.Setter
public Builder privateEndpoints(List privateEndpoints) {
if (privateEndpoints == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "privateEndpoints");
}
this.privateEndpoints = privateEndpoints;
return this;
}
public Builder privateEndpoints(PrivateEndpointResponse... privateEndpoints) {
return privateEndpoints(List.of(privateEndpoints));
}
@CustomType.Setter
public Builder privateLinkServiceNetworkPolicies(@Nullable String privateLinkServiceNetworkPolicies) {
this.privateLinkServiceNetworkPolicies = privateLinkServiceNetworkPolicies;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder purpose(String purpose) {
if (purpose == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "purpose");
}
this.purpose = purpose;
return this;
}
@CustomType.Setter
public Builder resourceNavigationLinks(List resourceNavigationLinks) {
if (resourceNavigationLinks == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "resourceNavigationLinks");
}
this.resourceNavigationLinks = resourceNavigationLinks;
return this;
}
public Builder resourceNavigationLinks(ResourceNavigationLinkResponse... resourceNavigationLinks) {
return resourceNavigationLinks(List.of(resourceNavigationLinks));
}
@CustomType.Setter
public Builder routeTable(@Nullable RouteTableResponse routeTable) {
this.routeTable = routeTable;
return this;
}
@CustomType.Setter
public Builder serviceAssociationLinks(List serviceAssociationLinks) {
if (serviceAssociationLinks == null) {
throw new MissingRequiredPropertyException("GetSubnetResult", "serviceAssociationLinks");
}
this.serviceAssociationLinks = serviceAssociationLinks;
return this;
}
public Builder serviceAssociationLinks(ServiceAssociationLinkResponse... serviceAssociationLinks) {
return serviceAssociationLinks(List.of(serviceAssociationLinks));
}
@CustomType.Setter
public Builder serviceEndpointPolicies(@Nullable List serviceEndpointPolicies) {
this.serviceEndpointPolicies = serviceEndpointPolicies;
return this;
}
public Builder serviceEndpointPolicies(ServiceEndpointPolicyResponse... serviceEndpointPolicies) {
return serviceEndpointPolicies(List.of(serviceEndpointPolicies));
}
@CustomType.Setter
public Builder serviceEndpoints(@Nullable List serviceEndpoints) {
this.serviceEndpoints = serviceEndpoints;
return this;
}
public Builder serviceEndpoints(ServiceEndpointPropertiesFormatResponse... serviceEndpoints) {
return serviceEndpoints(List.of(serviceEndpoints));
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
public GetSubnetResult build() {
final var _resultValue = new GetSubnetResult();
_resultValue.addressPrefix = addressPrefix;
_resultValue.addressPrefixes = addressPrefixes;
_resultValue.applicationGatewayIPConfigurations = applicationGatewayIPConfigurations;
_resultValue.delegations = delegations;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.ipAllocations = ipAllocations;
_resultValue.ipConfigurationProfiles = ipConfigurationProfiles;
_resultValue.ipConfigurations = ipConfigurations;
_resultValue.name = name;
_resultValue.natGateway = natGateway;
_resultValue.networkSecurityGroup = networkSecurityGroup;
_resultValue.privateEndpointNetworkPolicies = privateEndpointNetworkPolicies;
_resultValue.privateEndpoints = privateEndpoints;
_resultValue.privateLinkServiceNetworkPolicies = privateLinkServiceNetworkPolicies;
_resultValue.provisioningState = provisioningState;
_resultValue.purpose = purpose;
_resultValue.resourceNavigationLinks = resourceNavigationLinks;
_resultValue.routeTable = routeTable;
_resultValue.serviceAssociationLinks = serviceAssociationLinks;
_resultValue.serviceEndpointPolicies = serviceEndpointPolicies;
_resultValue.serviceEndpoints = serviceEndpoints;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy