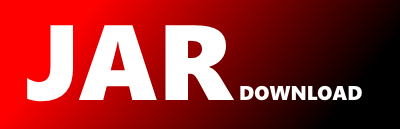
com.pulumi.azurenative.network.outputs.GetVirtualHubResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.azurenative.network.outputs.VirtualHubRouteTableResponse;
import com.pulumi.azurenative.network.outputs.VirtualHubRouteTableV2Response;
import com.pulumi.azurenative.network.outputs.VirtualRouterAutoScaleConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVirtualHubResult {
/**
* @return Address-prefix for this VirtualHub.
*
*/
private @Nullable String addressPrefix;
/**
* @return Flag to control transit for VirtualRouter hub.
*
*/
private @Nullable Boolean allowBranchToBranchTraffic;
/**
* @return The azureFirewall associated with this VirtualHub.
*
*/
private @Nullable SubResourceResponse azureFirewall;
/**
* @return List of references to Bgp Connections.
*
*/
private List bgpConnections;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return The expressRouteGateway associated with this VirtualHub.
*
*/
private @Nullable SubResourceResponse expressRouteGateway;
/**
* @return The hubRoutingPreference of this VirtualHub.
*
*/
private @Nullable String hubRoutingPreference;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return List of references to IpConfigurations.
*
*/
private List ipConfigurations;
/**
* @return Kind of service virtual hub. This is metadata used for the Azure portal experience for Route Server.
*
*/
private String kind;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The P2SVpnGateway associated with this VirtualHub.
*
*/
private @Nullable SubResourceResponse p2SVpnGateway;
/**
* @return The preferred gateway to route on-prem traffic
*
*/
private @Nullable String preferredRoutingGateway;
/**
* @return The provisioning state of the virtual hub resource.
*
*/
private String provisioningState;
/**
* @return List of references to RouteMaps.
*
*/
private List routeMaps;
/**
* @return The routeTable associated with this virtual hub.
*
*/
private @Nullable VirtualHubRouteTableResponse routeTable;
/**
* @return The routing state.
*
*/
private String routingState;
/**
* @return The securityPartnerProvider associated with this VirtualHub.
*
*/
private @Nullable SubResourceResponse securityPartnerProvider;
/**
* @return The Security Provider name.
*
*/
private @Nullable String securityProviderName;
/**
* @return The sku of this VirtualHub.
*
*/
private @Nullable String sku;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return List of all virtual hub route table v2s associated with this VirtualHub.
*
*/
private @Nullable List virtualHubRouteTableV2s;
/**
* @return VirtualRouter ASN.
*
*/
private @Nullable Double virtualRouterAsn;
/**
* @return The VirtualHub Router autoscale configuration.
*
*/
private @Nullable VirtualRouterAutoScaleConfigurationResponse virtualRouterAutoScaleConfiguration;
/**
* @return VirtualRouter IPs.
*
*/
private @Nullable List virtualRouterIps;
/**
* @return The VirtualWAN to which the VirtualHub belongs.
*
*/
private @Nullable SubResourceResponse virtualWan;
/**
* @return The VpnGateway associated with this VirtualHub.
*
*/
private @Nullable SubResourceResponse vpnGateway;
private GetVirtualHubResult() {}
/**
* @return Address-prefix for this VirtualHub.
*
*/
public Optional addressPrefix() {
return Optional.ofNullable(this.addressPrefix);
}
/**
* @return Flag to control transit for VirtualRouter hub.
*
*/
public Optional allowBranchToBranchTraffic() {
return Optional.ofNullable(this.allowBranchToBranchTraffic);
}
/**
* @return The azureFirewall associated with this VirtualHub.
*
*/
public Optional azureFirewall() {
return Optional.ofNullable(this.azureFirewall);
}
/**
* @return List of references to Bgp Connections.
*
*/
public List bgpConnections() {
return this.bgpConnections;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return The expressRouteGateway associated with this VirtualHub.
*
*/
public Optional expressRouteGateway() {
return Optional.ofNullable(this.expressRouteGateway);
}
/**
* @return The hubRoutingPreference of this VirtualHub.
*
*/
public Optional hubRoutingPreference() {
return Optional.ofNullable(this.hubRoutingPreference);
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return List of references to IpConfigurations.
*
*/
public List ipConfigurations() {
return this.ipConfigurations;
}
/**
* @return Kind of service virtual hub. This is metadata used for the Azure portal experience for Route Server.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The P2SVpnGateway associated with this VirtualHub.
*
*/
public Optional p2SVpnGateway() {
return Optional.ofNullable(this.p2SVpnGateway);
}
/**
* @return The preferred gateway to route on-prem traffic
*
*/
public Optional preferredRoutingGateway() {
return Optional.ofNullable(this.preferredRoutingGateway);
}
/**
* @return The provisioning state of the virtual hub resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return List of references to RouteMaps.
*
*/
public List routeMaps() {
return this.routeMaps;
}
/**
* @return The routeTable associated with this virtual hub.
*
*/
public Optional routeTable() {
return Optional.ofNullable(this.routeTable);
}
/**
* @return The routing state.
*
*/
public String routingState() {
return this.routingState;
}
/**
* @return The securityPartnerProvider associated with this VirtualHub.
*
*/
public Optional securityPartnerProvider() {
return Optional.ofNullable(this.securityPartnerProvider);
}
/**
* @return The Security Provider name.
*
*/
public Optional securityProviderName() {
return Optional.ofNullable(this.securityProviderName);
}
/**
* @return The sku of this VirtualHub.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return List of all virtual hub route table v2s associated with this VirtualHub.
*
*/
public List virtualHubRouteTableV2s() {
return this.virtualHubRouteTableV2s == null ? List.of() : this.virtualHubRouteTableV2s;
}
/**
* @return VirtualRouter ASN.
*
*/
public Optional virtualRouterAsn() {
return Optional.ofNullable(this.virtualRouterAsn);
}
/**
* @return The VirtualHub Router autoscale configuration.
*
*/
public Optional virtualRouterAutoScaleConfiguration() {
return Optional.ofNullable(this.virtualRouterAutoScaleConfiguration);
}
/**
* @return VirtualRouter IPs.
*
*/
public List virtualRouterIps() {
return this.virtualRouterIps == null ? List.of() : this.virtualRouterIps;
}
/**
* @return The VirtualWAN to which the VirtualHub belongs.
*
*/
public Optional virtualWan() {
return Optional.ofNullable(this.virtualWan);
}
/**
* @return The VpnGateway associated with this VirtualHub.
*
*/
public Optional vpnGateway() {
return Optional.ofNullable(this.vpnGateway);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVirtualHubResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String addressPrefix;
private @Nullable Boolean allowBranchToBranchTraffic;
private @Nullable SubResourceResponse azureFirewall;
private List bgpConnections;
private String etag;
private @Nullable SubResourceResponse expressRouteGateway;
private @Nullable String hubRoutingPreference;
private @Nullable String id;
private List ipConfigurations;
private String kind;
private String location;
private String name;
private @Nullable SubResourceResponse p2SVpnGateway;
private @Nullable String preferredRoutingGateway;
private String provisioningState;
private List routeMaps;
private @Nullable VirtualHubRouteTableResponse routeTable;
private String routingState;
private @Nullable SubResourceResponse securityPartnerProvider;
private @Nullable String securityProviderName;
private @Nullable String sku;
private @Nullable Map tags;
private String type;
private @Nullable List virtualHubRouteTableV2s;
private @Nullable Double virtualRouterAsn;
private @Nullable VirtualRouterAutoScaleConfigurationResponse virtualRouterAutoScaleConfiguration;
private @Nullable List virtualRouterIps;
private @Nullable SubResourceResponse virtualWan;
private @Nullable SubResourceResponse vpnGateway;
public Builder() {}
public Builder(GetVirtualHubResult defaults) {
Objects.requireNonNull(defaults);
this.addressPrefix = defaults.addressPrefix;
this.allowBranchToBranchTraffic = defaults.allowBranchToBranchTraffic;
this.azureFirewall = defaults.azureFirewall;
this.bgpConnections = defaults.bgpConnections;
this.etag = defaults.etag;
this.expressRouteGateway = defaults.expressRouteGateway;
this.hubRoutingPreference = defaults.hubRoutingPreference;
this.id = defaults.id;
this.ipConfigurations = defaults.ipConfigurations;
this.kind = defaults.kind;
this.location = defaults.location;
this.name = defaults.name;
this.p2SVpnGateway = defaults.p2SVpnGateway;
this.preferredRoutingGateway = defaults.preferredRoutingGateway;
this.provisioningState = defaults.provisioningState;
this.routeMaps = defaults.routeMaps;
this.routeTable = defaults.routeTable;
this.routingState = defaults.routingState;
this.securityPartnerProvider = defaults.securityPartnerProvider;
this.securityProviderName = defaults.securityProviderName;
this.sku = defaults.sku;
this.tags = defaults.tags;
this.type = defaults.type;
this.virtualHubRouteTableV2s = defaults.virtualHubRouteTableV2s;
this.virtualRouterAsn = defaults.virtualRouterAsn;
this.virtualRouterAutoScaleConfiguration = defaults.virtualRouterAutoScaleConfiguration;
this.virtualRouterIps = defaults.virtualRouterIps;
this.virtualWan = defaults.virtualWan;
this.vpnGateway = defaults.vpnGateway;
}
@CustomType.Setter
public Builder addressPrefix(@Nullable String addressPrefix) {
this.addressPrefix = addressPrefix;
return this;
}
@CustomType.Setter
public Builder allowBranchToBranchTraffic(@Nullable Boolean allowBranchToBranchTraffic) {
this.allowBranchToBranchTraffic = allowBranchToBranchTraffic;
return this;
}
@CustomType.Setter
public Builder azureFirewall(@Nullable SubResourceResponse azureFirewall) {
this.azureFirewall = azureFirewall;
return this;
}
@CustomType.Setter
public Builder bgpConnections(List bgpConnections) {
if (bgpConnections == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "bgpConnections");
}
this.bgpConnections = bgpConnections;
return this;
}
public Builder bgpConnections(SubResourceResponse... bgpConnections) {
return bgpConnections(List.of(bgpConnections));
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder expressRouteGateway(@Nullable SubResourceResponse expressRouteGateway) {
this.expressRouteGateway = expressRouteGateway;
return this;
}
@CustomType.Setter
public Builder hubRoutingPreference(@Nullable String hubRoutingPreference) {
this.hubRoutingPreference = hubRoutingPreference;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipConfigurations(List ipConfigurations) {
if (ipConfigurations == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "ipConfigurations");
}
this.ipConfigurations = ipConfigurations;
return this;
}
public Builder ipConfigurations(SubResourceResponse... ipConfigurations) {
return ipConfigurations(List.of(ipConfigurations));
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder p2SVpnGateway(@Nullable SubResourceResponse p2SVpnGateway) {
this.p2SVpnGateway = p2SVpnGateway;
return this;
}
@CustomType.Setter
public Builder preferredRoutingGateway(@Nullable String preferredRoutingGateway) {
this.preferredRoutingGateway = preferredRoutingGateway;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder routeMaps(List routeMaps) {
if (routeMaps == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "routeMaps");
}
this.routeMaps = routeMaps;
return this;
}
public Builder routeMaps(SubResourceResponse... routeMaps) {
return routeMaps(List.of(routeMaps));
}
@CustomType.Setter
public Builder routeTable(@Nullable VirtualHubRouteTableResponse routeTable) {
this.routeTable = routeTable;
return this;
}
@CustomType.Setter
public Builder routingState(String routingState) {
if (routingState == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "routingState");
}
this.routingState = routingState;
return this;
}
@CustomType.Setter
public Builder securityPartnerProvider(@Nullable SubResourceResponse securityPartnerProvider) {
this.securityPartnerProvider = securityPartnerProvider;
return this;
}
@CustomType.Setter
public Builder securityProviderName(@Nullable String securityProviderName) {
this.securityProviderName = securityProviderName;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable String sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetVirtualHubResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualHubRouteTableV2s(@Nullable List virtualHubRouteTableV2s) {
this.virtualHubRouteTableV2s = virtualHubRouteTableV2s;
return this;
}
public Builder virtualHubRouteTableV2s(VirtualHubRouteTableV2Response... virtualHubRouteTableV2s) {
return virtualHubRouteTableV2s(List.of(virtualHubRouteTableV2s));
}
@CustomType.Setter
public Builder virtualRouterAsn(@Nullable Double virtualRouterAsn) {
this.virtualRouterAsn = virtualRouterAsn;
return this;
}
@CustomType.Setter
public Builder virtualRouterAutoScaleConfiguration(@Nullable VirtualRouterAutoScaleConfigurationResponse virtualRouterAutoScaleConfiguration) {
this.virtualRouterAutoScaleConfiguration = virtualRouterAutoScaleConfiguration;
return this;
}
@CustomType.Setter
public Builder virtualRouterIps(@Nullable List virtualRouterIps) {
this.virtualRouterIps = virtualRouterIps;
return this;
}
public Builder virtualRouterIps(String... virtualRouterIps) {
return virtualRouterIps(List.of(virtualRouterIps));
}
@CustomType.Setter
public Builder virtualWan(@Nullable SubResourceResponse virtualWan) {
this.virtualWan = virtualWan;
return this;
}
@CustomType.Setter
public Builder vpnGateway(@Nullable SubResourceResponse vpnGateway) {
this.vpnGateway = vpnGateway;
return this;
}
public GetVirtualHubResult build() {
final var _resultValue = new GetVirtualHubResult();
_resultValue.addressPrefix = addressPrefix;
_resultValue.allowBranchToBranchTraffic = allowBranchToBranchTraffic;
_resultValue.azureFirewall = azureFirewall;
_resultValue.bgpConnections = bgpConnections;
_resultValue.etag = etag;
_resultValue.expressRouteGateway = expressRouteGateway;
_resultValue.hubRoutingPreference = hubRoutingPreference;
_resultValue.id = id;
_resultValue.ipConfigurations = ipConfigurations;
_resultValue.kind = kind;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.p2SVpnGateway = p2SVpnGateway;
_resultValue.preferredRoutingGateway = preferredRoutingGateway;
_resultValue.provisioningState = provisioningState;
_resultValue.routeMaps = routeMaps;
_resultValue.routeTable = routeTable;
_resultValue.routingState = routingState;
_resultValue.securityPartnerProvider = securityPartnerProvider;
_resultValue.securityProviderName = securityProviderName;
_resultValue.sku = sku;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.virtualHubRouteTableV2s = virtualHubRouteTableV2s;
_resultValue.virtualRouterAsn = virtualRouterAsn;
_resultValue.virtualRouterAutoScaleConfiguration = virtualRouterAutoScaleConfiguration;
_resultValue.virtualRouterIps = virtualRouterIps;
_resultValue.virtualWan = virtualWan;
_resultValue.vpnGateway = vpnGateway;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy