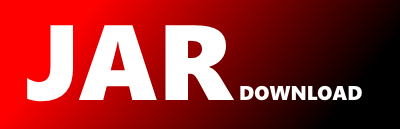
com.pulumi.azurenative.network.outputs.ProbeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ProbeResponse {
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return The interval, in seconds, for how frequently to probe the endpoint for health status. Typically, the interval is slightly less than half the allocated timeout period (in seconds) which allows two full probes before taking the instance out of rotation. The default value is 15, the minimum value is 5.
*
*/
private @Nullable Integer intervalInSeconds;
/**
* @return The load balancer rules that use this probe.
*
*/
private List loadBalancingRules;
/**
* @return The name of the resource that is unique within the set of probes used by the load balancer. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return The number of probes where if no response, will result in stopping further traffic from being delivered to the endpoint. This values allows endpoints to be taken out of rotation faster or slower than the typical times used in Azure.
*
*/
private @Nullable Integer numberOfProbes;
/**
* @return The port for communicating the probe. Possible values range from 1 to 65535, inclusive.
*
*/
private Integer port;
/**
* @return The number of consecutive successful or failed probes in order to allow or deny traffic from being delivered to this endpoint. After failing the number of consecutive probes equal to this value, the endpoint will be taken out of rotation and require the same number of successful consecutive probes to be placed back in rotation.
*
*/
private @Nullable Integer probeThreshold;
/**
* @return The protocol of the end point. If 'Tcp' is specified, a received ACK is required for the probe to be successful. If 'Http' or 'Https' is specified, a 200 OK response from the specifies URI is required for the probe to be successful.
*
*/
private String protocol;
/**
* @return The provisioning state of the probe resource.
*
*/
private String provisioningState;
/**
* @return The URI used for requesting health status from the VM. Path is required if a protocol is set to http. Otherwise, it is not allowed. There is no default value.
*
*/
private @Nullable String requestPath;
/**
* @return Type of the resource.
*
*/
private String type;
private ProbeResponse() {}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The interval, in seconds, for how frequently to probe the endpoint for health status. Typically, the interval is slightly less than half the allocated timeout period (in seconds) which allows two full probes before taking the instance out of rotation. The default value is 15, the minimum value is 5.
*
*/
public Optional intervalInSeconds() {
return Optional.ofNullable(this.intervalInSeconds);
}
/**
* @return The load balancer rules that use this probe.
*
*/
public List loadBalancingRules() {
return this.loadBalancingRules;
}
/**
* @return The name of the resource that is unique within the set of probes used by the load balancer. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The number of probes where if no response, will result in stopping further traffic from being delivered to the endpoint. This values allows endpoints to be taken out of rotation faster or slower than the typical times used in Azure.
*
*/
public Optional numberOfProbes() {
return Optional.ofNullable(this.numberOfProbes);
}
/**
* @return The port for communicating the probe. Possible values range from 1 to 65535, inclusive.
*
*/
public Integer port() {
return this.port;
}
/**
* @return The number of consecutive successful or failed probes in order to allow or deny traffic from being delivered to this endpoint. After failing the number of consecutive probes equal to this value, the endpoint will be taken out of rotation and require the same number of successful consecutive probes to be placed back in rotation.
*
*/
public Optional probeThreshold() {
return Optional.ofNullable(this.probeThreshold);
}
/**
* @return The protocol of the end point. If 'Tcp' is specified, a received ACK is required for the probe to be successful. If 'Http' or 'Https' is specified, a 200 OK response from the specifies URI is required for the probe to be successful.
*
*/
public String protocol() {
return this.protocol;
}
/**
* @return The provisioning state of the probe resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The URI used for requesting health status from the VM. Path is required if a protocol is set to http. Otherwise, it is not allowed. There is no default value.
*
*/
public Optional requestPath() {
return Optional.ofNullable(this.requestPath);
}
/**
* @return Type of the resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProbeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String etag;
private @Nullable String id;
private @Nullable Integer intervalInSeconds;
private List loadBalancingRules;
private @Nullable String name;
private @Nullable Integer numberOfProbes;
private Integer port;
private @Nullable Integer probeThreshold;
private String protocol;
private String provisioningState;
private @Nullable String requestPath;
private String type;
public Builder() {}
public Builder(ProbeResponse defaults) {
Objects.requireNonNull(defaults);
this.etag = defaults.etag;
this.id = defaults.id;
this.intervalInSeconds = defaults.intervalInSeconds;
this.loadBalancingRules = defaults.loadBalancingRules;
this.name = defaults.name;
this.numberOfProbes = defaults.numberOfProbes;
this.port = defaults.port;
this.probeThreshold = defaults.probeThreshold;
this.protocol = defaults.protocol;
this.provisioningState = defaults.provisioningState;
this.requestPath = defaults.requestPath;
this.type = defaults.type;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("ProbeResponse", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder intervalInSeconds(@Nullable Integer intervalInSeconds) {
this.intervalInSeconds = intervalInSeconds;
return this;
}
@CustomType.Setter
public Builder loadBalancingRules(List loadBalancingRules) {
if (loadBalancingRules == null) {
throw new MissingRequiredPropertyException("ProbeResponse", "loadBalancingRules");
}
this.loadBalancingRules = loadBalancingRules;
return this;
}
public Builder loadBalancingRules(SubResourceResponse... loadBalancingRules) {
return loadBalancingRules(List.of(loadBalancingRules));
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder numberOfProbes(@Nullable Integer numberOfProbes) {
this.numberOfProbes = numberOfProbes;
return this;
}
@CustomType.Setter
public Builder port(Integer port) {
if (port == null) {
throw new MissingRequiredPropertyException("ProbeResponse", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder probeThreshold(@Nullable Integer probeThreshold) {
this.probeThreshold = probeThreshold;
return this;
}
@CustomType.Setter
public Builder protocol(String protocol) {
if (protocol == null) {
throw new MissingRequiredPropertyException("ProbeResponse", "protocol");
}
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("ProbeResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder requestPath(@Nullable String requestPath) {
this.requestPath = requestPath;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ProbeResponse", "type");
}
this.type = type;
return this;
}
public ProbeResponse build() {
final var _resultValue = new ProbeResponse();
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.intervalInSeconds = intervalInSeconds;
_resultValue.loadBalancingRules = loadBalancingRules;
_resultValue.name = name;
_resultValue.numberOfProbes = numberOfProbes;
_resultValue.port = port;
_resultValue.probeThreshold = probeThreshold;
_resultValue.protocol = protocol;
_resultValue.provisioningState = provisioningState;
_resultValue.requestPath = requestPath;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy