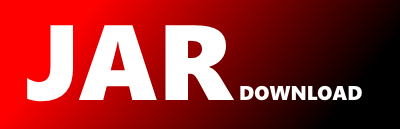
com.pulumi.azurenative.network.outputs.SecurityRuleResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.ApplicationSecurityGroupResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SecurityRuleResponse {
/**
* @return The network traffic is allowed or denied.
*
*/
private String access;
/**
* @return A description for this rule. Restricted to 140 chars.
*
*/
private @Nullable String description;
/**
* @return The destination address prefix. CIDR or destination IP range. Asterisk '*' can also be used to match all source IPs. Default tags such as 'VirtualNetwork', 'AzureLoadBalancer' and 'Internet' can also be used.
*
*/
private @Nullable String destinationAddressPrefix;
/**
* @return The destination address prefixes. CIDR or destination IP ranges.
*
*/
private @Nullable List destinationAddressPrefixes;
/**
* @return The application security group specified as destination.
*
*/
private @Nullable List destinationApplicationSecurityGroups;
/**
* @return The destination port or range. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
private @Nullable String destinationPortRange;
/**
* @return The destination port ranges.
*
*/
private @Nullable List destinationPortRanges;
/**
* @return The direction of the rule. The direction specifies if rule will be evaluated on incoming or outgoing traffic.
*
*/
private String direction;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return The priority of the rule. The value can be between 100 and 4096. The priority number must be unique for each rule in the collection. The lower the priority number, the higher the priority of the rule.
*
*/
private Integer priority;
/**
* @return Network protocol this rule applies to.
*
*/
private String protocol;
/**
* @return The provisioning state of the security rule resource.
*
*/
private String provisioningState;
/**
* @return The CIDR or source IP range. Asterisk '*' can also be used to match all source IPs. Default tags such as 'VirtualNetwork', 'AzureLoadBalancer' and 'Internet' can also be used. If this is an ingress rule, specifies where network traffic originates from.
*
*/
private @Nullable String sourceAddressPrefix;
/**
* @return The CIDR or source IP ranges.
*
*/
private @Nullable List sourceAddressPrefixes;
/**
* @return The application security group specified as source.
*
*/
private @Nullable List sourceApplicationSecurityGroups;
/**
* @return The source port or range. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
private @Nullable String sourcePortRange;
/**
* @return The source port ranges.
*
*/
private @Nullable List sourcePortRanges;
/**
* @return The type of the resource.
*
*/
private @Nullable String type;
private SecurityRuleResponse() {}
/**
* @return The network traffic is allowed or denied.
*
*/
public String access() {
return this.access;
}
/**
* @return A description for this rule. Restricted to 140 chars.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The destination address prefix. CIDR or destination IP range. Asterisk '*' can also be used to match all source IPs. Default tags such as 'VirtualNetwork', 'AzureLoadBalancer' and 'Internet' can also be used.
*
*/
public Optional destinationAddressPrefix() {
return Optional.ofNullable(this.destinationAddressPrefix);
}
/**
* @return The destination address prefixes. CIDR or destination IP ranges.
*
*/
public List destinationAddressPrefixes() {
return this.destinationAddressPrefixes == null ? List.of() : this.destinationAddressPrefixes;
}
/**
* @return The application security group specified as destination.
*
*/
public List destinationApplicationSecurityGroups() {
return this.destinationApplicationSecurityGroups == null ? List.of() : this.destinationApplicationSecurityGroups;
}
/**
* @return The destination port or range. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
public Optional destinationPortRange() {
return Optional.ofNullable(this.destinationPortRange);
}
/**
* @return The destination port ranges.
*
*/
public List destinationPortRanges() {
return this.destinationPortRanges == null ? List.of() : this.destinationPortRanges;
}
/**
* @return The direction of the rule. The direction specifies if rule will be evaluated on incoming or outgoing traffic.
*
*/
public String direction() {
return this.direction;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The priority of the rule. The value can be between 100 and 4096. The priority number must be unique for each rule in the collection. The lower the priority number, the higher the priority of the rule.
*
*/
public Integer priority() {
return this.priority;
}
/**
* @return Network protocol this rule applies to.
*
*/
public String protocol() {
return this.protocol;
}
/**
* @return The provisioning state of the security rule resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The CIDR or source IP range. Asterisk '*' can also be used to match all source IPs. Default tags such as 'VirtualNetwork', 'AzureLoadBalancer' and 'Internet' can also be used. If this is an ingress rule, specifies where network traffic originates from.
*
*/
public Optional sourceAddressPrefix() {
return Optional.ofNullable(this.sourceAddressPrefix);
}
/**
* @return The CIDR or source IP ranges.
*
*/
public List sourceAddressPrefixes() {
return this.sourceAddressPrefixes == null ? List.of() : this.sourceAddressPrefixes;
}
/**
* @return The application security group specified as source.
*
*/
public List sourceApplicationSecurityGroups() {
return this.sourceApplicationSecurityGroups == null ? List.of() : this.sourceApplicationSecurityGroups;
}
/**
* @return The source port or range. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
public Optional sourcePortRange() {
return Optional.ofNullable(this.sourcePortRange);
}
/**
* @return The source port ranges.
*
*/
public List sourcePortRanges() {
return this.sourcePortRanges == null ? List.of() : this.sourcePortRanges;
}
/**
* @return The type of the resource.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SecurityRuleResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String access;
private @Nullable String description;
private @Nullable String destinationAddressPrefix;
private @Nullable List destinationAddressPrefixes;
private @Nullable List destinationApplicationSecurityGroups;
private @Nullable String destinationPortRange;
private @Nullable List destinationPortRanges;
private String direction;
private String etag;
private @Nullable String id;
private @Nullable String name;
private Integer priority;
private String protocol;
private String provisioningState;
private @Nullable String sourceAddressPrefix;
private @Nullable List sourceAddressPrefixes;
private @Nullable List sourceApplicationSecurityGroups;
private @Nullable String sourcePortRange;
private @Nullable List sourcePortRanges;
private @Nullable String type;
public Builder() {}
public Builder(SecurityRuleResponse defaults) {
Objects.requireNonNull(defaults);
this.access = defaults.access;
this.description = defaults.description;
this.destinationAddressPrefix = defaults.destinationAddressPrefix;
this.destinationAddressPrefixes = defaults.destinationAddressPrefixes;
this.destinationApplicationSecurityGroups = defaults.destinationApplicationSecurityGroups;
this.destinationPortRange = defaults.destinationPortRange;
this.destinationPortRanges = defaults.destinationPortRanges;
this.direction = defaults.direction;
this.etag = defaults.etag;
this.id = defaults.id;
this.name = defaults.name;
this.priority = defaults.priority;
this.protocol = defaults.protocol;
this.provisioningState = defaults.provisioningState;
this.sourceAddressPrefix = defaults.sourceAddressPrefix;
this.sourceAddressPrefixes = defaults.sourceAddressPrefixes;
this.sourceApplicationSecurityGroups = defaults.sourceApplicationSecurityGroups;
this.sourcePortRange = defaults.sourcePortRange;
this.sourcePortRanges = defaults.sourcePortRanges;
this.type = defaults.type;
}
@CustomType.Setter
public Builder access(String access) {
if (access == null) {
throw new MissingRequiredPropertyException("SecurityRuleResponse", "access");
}
this.access = access;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder destinationAddressPrefix(@Nullable String destinationAddressPrefix) {
this.destinationAddressPrefix = destinationAddressPrefix;
return this;
}
@CustomType.Setter
public Builder destinationAddressPrefixes(@Nullable List destinationAddressPrefixes) {
this.destinationAddressPrefixes = destinationAddressPrefixes;
return this;
}
public Builder destinationAddressPrefixes(String... destinationAddressPrefixes) {
return destinationAddressPrefixes(List.of(destinationAddressPrefixes));
}
@CustomType.Setter
public Builder destinationApplicationSecurityGroups(@Nullable List destinationApplicationSecurityGroups) {
this.destinationApplicationSecurityGroups = destinationApplicationSecurityGroups;
return this;
}
public Builder destinationApplicationSecurityGroups(ApplicationSecurityGroupResponse... destinationApplicationSecurityGroups) {
return destinationApplicationSecurityGroups(List.of(destinationApplicationSecurityGroups));
}
@CustomType.Setter
public Builder destinationPortRange(@Nullable String destinationPortRange) {
this.destinationPortRange = destinationPortRange;
return this;
}
@CustomType.Setter
public Builder destinationPortRanges(@Nullable List destinationPortRanges) {
this.destinationPortRanges = destinationPortRanges;
return this;
}
public Builder destinationPortRanges(String... destinationPortRanges) {
return destinationPortRanges(List.of(destinationPortRanges));
}
@CustomType.Setter
public Builder direction(String direction) {
if (direction == null) {
throw new MissingRequiredPropertyException("SecurityRuleResponse", "direction");
}
this.direction = direction;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("SecurityRuleResponse", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(Integer priority) {
if (priority == null) {
throw new MissingRequiredPropertyException("SecurityRuleResponse", "priority");
}
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder protocol(String protocol) {
if (protocol == null) {
throw new MissingRequiredPropertyException("SecurityRuleResponse", "protocol");
}
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("SecurityRuleResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sourceAddressPrefix(@Nullable String sourceAddressPrefix) {
this.sourceAddressPrefix = sourceAddressPrefix;
return this;
}
@CustomType.Setter
public Builder sourceAddressPrefixes(@Nullable List sourceAddressPrefixes) {
this.sourceAddressPrefixes = sourceAddressPrefixes;
return this;
}
public Builder sourceAddressPrefixes(String... sourceAddressPrefixes) {
return sourceAddressPrefixes(List.of(sourceAddressPrefixes));
}
@CustomType.Setter
public Builder sourceApplicationSecurityGroups(@Nullable List sourceApplicationSecurityGroups) {
this.sourceApplicationSecurityGroups = sourceApplicationSecurityGroups;
return this;
}
public Builder sourceApplicationSecurityGroups(ApplicationSecurityGroupResponse... sourceApplicationSecurityGroups) {
return sourceApplicationSecurityGroups(List.of(sourceApplicationSecurityGroups));
}
@CustomType.Setter
public Builder sourcePortRange(@Nullable String sourcePortRange) {
this.sourcePortRange = sourcePortRange;
return this;
}
@CustomType.Setter
public Builder sourcePortRanges(@Nullable List sourcePortRanges) {
this.sourcePortRanges = sourcePortRanges;
return this;
}
public Builder sourcePortRanges(String... sourcePortRanges) {
return sourcePortRanges(List.of(sourcePortRanges));
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
public SecurityRuleResponse build() {
final var _resultValue = new SecurityRuleResponse();
_resultValue.access = access;
_resultValue.description = description;
_resultValue.destinationAddressPrefix = destinationAddressPrefix;
_resultValue.destinationAddressPrefixes = destinationAddressPrefixes;
_resultValue.destinationApplicationSecurityGroups = destinationApplicationSecurityGroups;
_resultValue.destinationPortRange = destinationPortRange;
_resultValue.destinationPortRanges = destinationPortRanges;
_resultValue.direction = direction;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.protocol = protocol;
_resultValue.provisioningState = provisioningState;
_resultValue.sourceAddressPrefix = sourceAddressPrefix;
_resultValue.sourceAddressPrefixes = sourceAddressPrefixes;
_resultValue.sourceApplicationSecurityGroups = sourceApplicationSecurityGroups;
_resultValue.sourcePortRange = sourcePortRange;
_resultValue.sourcePortRanges = sourcePortRanges;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy