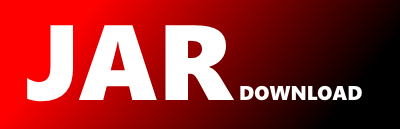
com.pulumi.azurenative.networkcloud.Cluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.networkcloud.ClusterArgs;
import com.pulumi.azurenative.networkcloud.outputs.ClusterAvailableUpgradeVersionResponse;
import com.pulumi.azurenative.networkcloud.outputs.ClusterCapacityResponse;
import com.pulumi.azurenative.networkcloud.outputs.ClusterSecretArchiveResponse;
import com.pulumi.azurenative.networkcloud.outputs.ClusterUpdateStrategyResponse;
import com.pulumi.azurenative.networkcloud.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.networkcloud.outputs.ManagedResourceGroupConfigurationResponse;
import com.pulumi.azurenative.networkcloud.outputs.RackDefinitionResponse;
import com.pulumi.azurenative.networkcloud.outputs.RuntimeProtectionConfigurationResponse;
import com.pulumi.azurenative.networkcloud.outputs.ServicePrincipalInformationResponse;
import com.pulumi.azurenative.networkcloud.outputs.SystemDataResponse;
import com.pulumi.azurenative.networkcloud.outputs.ValidationThresholdResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Azure REST API version: 2023-10-01-preview. Prior API version in Azure Native 1.x: 2022-12-12-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
* ## Example Usage
* ### Create or update cluster
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.networkcloud.Cluster;
* import com.pulumi.azurenative.networkcloud.ClusterArgs;
* import com.pulumi.azurenative.networkcloud.inputs.RackDefinitionArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ServicePrincipalInformationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ValidationThresholdArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ManagedResourceGroupConfigurationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.RuntimeProtectionConfigurationArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ClusterSecretArchiveArgs;
* import com.pulumi.azurenative.networkcloud.inputs.ClusterUpdateStrategyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var cluster = new Cluster("cluster", ClusterArgs.builder()
* .aggregatorOrSingleRackDefinition(RackDefinitionArgs.builder()
* .bareMetalMachineConfigurationData(
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:FF")
* .bootMacAddress("00:BB:CC:DD:EE:FF")
* .machineDetails("extraDetails")
* .machineName("bmmName1")
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .build(),
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:00")
* .bootMacAddress("00:BB:CC:DD:EE:00")
* .machineDetails("extraDetails")
* .machineName("bmmName2")
* .rackSlot(2)
* .serialNumber("BM1219YYY")
* .build())
* .networkRackId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName")
* .rackLocation("Foo Datacenter, Floor 3, Aisle 9, Rack 2")
* .rackSerialNumber("AA1234")
* .rackSkuId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName")
* .storageApplianceConfigurationData(StorageApplianceConfigurationDataArgs.builder()
* .adminCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .storageApplianceName("vmName")
* .build())
* .build())
* .analyticsWorkspaceId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/microsoft.operationalInsights/workspaces/logAnalyticsWorkspaceName")
* .clusterLocation("Foo Street, 3rd Floor, row 9")
* .clusterName("clusterName")
* .clusterServicePrincipal(ServicePrincipalInformationArgs.builder()
* .applicationId("12345678-1234-1234-1234-123456789012")
* .password("{password}")
* .principalId("00000008-0004-0004-0004-000000000012")
* .tenantId("80000000-4000-4000-4000-120000000000")
* .build())
* .clusterType("SingleRack")
* .clusterVersion("1.0.0")
* .computeDeploymentThreshold(ValidationThresholdArgs.builder()
* .grouping("PerCluster")
* .type("PercentSuccess")
* .value(90)
* .build())
* .computeRackDefinitions(RackDefinitionArgs.builder()
* .bareMetalMachineConfigurationData(
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:FF")
* .bootMacAddress("00:BB:CC:DD:EE:FF")
* .machineDetails("extraDetails")
* .machineName("bmmName1")
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .build(),
* BareMetalMachineConfigurationDataArgs.builder()
* .bmcCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .bmcMacAddress("AA:BB:CC:DD:EE:00")
* .bootMacAddress("00:BB:CC:DD:EE:00")
* .machineDetails("extraDetails")
* .machineName("bmmName2")
* .rackSlot(2)
* .serialNumber("BM1219YYY")
* .build())
* .networkRackId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkRacks/networkRackName")
* .rackLocation("Foo Datacenter, Floor 3, Aisle 9, Rack 2")
* .rackSerialNumber("AA1234")
* .rackSkuId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/providers/Microsoft.NetworkCloud/rackSkus/rackSkuName")
* .storageApplianceConfigurationData(StorageApplianceConfigurationDataArgs.builder()
* .adminCredentials(AdministrativeCredentialsArgs.builder()
* .password("{password}")
* .username("username")
* .build())
* .rackSlot(1)
* .serialNumber("BM1219XXX")
* .storageApplianceName("vmName")
* .build())
* .build())
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ExtendedLocation/customLocations/clusterManagerExtendedLocationName")
* .type("CustomLocation")
* .build())
* .location("location")
* .managedResourceGroupConfiguration(ManagedResourceGroupConfigurationArgs.builder()
* .location("East US")
* .name("my-managed-rg")
* .build())
* .networkFabricId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.ManagedNetworkFabric/networkFabrics/fabricName")
* .resourceGroupName("resourceGroupName")
* .runtimeProtectionConfiguration(RuntimeProtectionConfigurationArgs.builder()
* .enforcementLevel("OnDemand")
* .build())
* .secretArchive(ClusterSecretArchiveArgs.builder()
* .keyVaultId("/subscriptions/123e4567-e89b-12d3-a456-426655440000/resourceGroups/resourceGroupName/providers/Microsoft.KeyVault/vaults/keyVaultName")
* .useKeyVault("True")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "myvalue1"),
* Map.entry("key2", "myvalue2")
* ))
* .updateStrategy(ClusterUpdateStrategyArgs.builder()
* .maxUnavailable(4)
* .strategyType("Rack")
* .thresholdType("CountSuccess")
* .thresholdValue(4)
* .waitTimeMinutes(10)
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:networkcloud:Cluster clusterName /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetworkCloud/clusters/{clusterName}
* ```
*
*/
@ResourceType(type="azure-native:networkcloud:Cluster")
public class Cluster extends com.pulumi.resources.CustomResource {
/**
* The rack definition that is intended to reflect only a single rack in a single rack cluster, or an aggregator rack in a multi-rack cluster.
*
*/
@Export(name="aggregatorOrSingleRackDefinition", refs={RackDefinitionResponse.class}, tree="[0]")
private Output aggregatorOrSingleRackDefinition;
/**
* @return The rack definition that is intended to reflect only a single rack in a single rack cluster, or an aggregator rack in a multi-rack cluster.
*
*/
public Output aggregatorOrSingleRackDefinition() {
return this.aggregatorOrSingleRackDefinition;
}
/**
* The resource ID of the Log Analytics Workspace that will be used for storing relevant logs.
*
*/
@Export(name="analyticsWorkspaceId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> analyticsWorkspaceId;
/**
* @return The resource ID of the Log Analytics Workspace that will be used for storing relevant logs.
*
*/
public Output> analyticsWorkspaceId() {
return Codegen.optional(this.analyticsWorkspaceId);
}
/**
* The list of cluster runtime version upgrades available for this cluster.
*
*/
@Export(name="availableUpgradeVersions", refs={List.class,ClusterAvailableUpgradeVersionResponse.class}, tree="[0,1]")
private Output> availableUpgradeVersions;
/**
* @return The list of cluster runtime version upgrades available for this cluster.
*
*/
public Output> availableUpgradeVersions() {
return this.availableUpgradeVersions;
}
/**
* The capacity supported by this cluster.
*
*/
@Export(name="clusterCapacity", refs={ClusterCapacityResponse.class}, tree="[0]")
private Output clusterCapacity;
/**
* @return The capacity supported by this cluster.
*
*/
public Output clusterCapacity() {
return this.clusterCapacity;
}
/**
* The latest heartbeat status between the cluster manager and the cluster.
*
*/
@Export(name="clusterConnectionStatus", refs={String.class}, tree="[0]")
private Output clusterConnectionStatus;
/**
* @return The latest heartbeat status between the cluster manager and the cluster.
*
*/
public Output clusterConnectionStatus() {
return this.clusterConnectionStatus;
}
/**
* The extended location (custom location) that represents the cluster's control plane location. This extended location is used to route the requests of child objects of the cluster that are handled by the platform operator.
*
*/
@Export(name="clusterExtendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output clusterExtendedLocation;
/**
* @return The extended location (custom location) that represents the cluster's control plane location. This extended location is used to route the requests of child objects of the cluster that are handled by the platform operator.
*
*/
public Output clusterExtendedLocation() {
return this.clusterExtendedLocation;
}
/**
* The customer-provided location information to identify where the cluster resides.
*
*/
@Export(name="clusterLocation", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> clusterLocation;
/**
* @return The customer-provided location information to identify where the cluster resides.
*
*/
public Output> clusterLocation() {
return Codegen.optional(this.clusterLocation);
}
/**
* The latest connectivity status between cluster manager and the cluster.
*
*/
@Export(name="clusterManagerConnectionStatus", refs={String.class}, tree="[0]")
private Output clusterManagerConnectionStatus;
/**
* @return The latest connectivity status between cluster manager and the cluster.
*
*/
public Output clusterManagerConnectionStatus() {
return this.clusterManagerConnectionStatus;
}
/**
* The resource ID of the cluster manager that manages this cluster. This is set by the Cluster Manager when the cluster is created.
*
*/
@Export(name="clusterManagerId", refs={String.class}, tree="[0]")
private Output clusterManagerId;
/**
* @return The resource ID of the cluster manager that manages this cluster. This is set by the Cluster Manager when the cluster is created.
*
*/
public Output clusterManagerId() {
return this.clusterManagerId;
}
/**
* The service principal to be used by the cluster during Arc Appliance installation.
*
*/
@Export(name="clusterServicePrincipal", refs={ServicePrincipalInformationResponse.class}, tree="[0]")
private Output* @Nullable */ ServicePrincipalInformationResponse> clusterServicePrincipal;
/**
* @return The service principal to be used by the cluster during Arc Appliance installation.
*
*/
public Output> clusterServicePrincipal() {
return Codegen.optional(this.clusterServicePrincipal);
}
/**
* The type of rack configuration for the cluster.
*
*/
@Export(name="clusterType", refs={String.class}, tree="[0]")
private Output clusterType;
/**
* @return The type of rack configuration for the cluster.
*
*/
public Output clusterType() {
return this.clusterType;
}
/**
* The current runtime version of the cluster.
*
*/
@Export(name="clusterVersion", refs={String.class}, tree="[0]")
private Output clusterVersion;
/**
* @return The current runtime version of the cluster.
*
*/
public Output clusterVersion() {
return this.clusterVersion;
}
/**
* The validation threshold indicating the allowable failures of compute machines during environment validation and deployment.
*
*/
@Export(name="computeDeploymentThreshold", refs={ValidationThresholdResponse.class}, tree="[0]")
private Output* @Nullable */ ValidationThresholdResponse> computeDeploymentThreshold;
/**
* @return The validation threshold indicating the allowable failures of compute machines during environment validation and deployment.
*
*/
public Output> computeDeploymentThreshold() {
return Codegen.optional(this.computeDeploymentThreshold);
}
/**
* The list of rack definitions for the compute racks in a multi-rack
* cluster, or an empty list in a single-rack cluster.
*
*/
@Export(name="computeRackDefinitions", refs={List.class,RackDefinitionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> computeRackDefinitions;
/**
* @return The list of rack definitions for the compute racks in a multi-rack
* cluster, or an empty list in a single-rack cluster.
*
*/
public Output>> computeRackDefinitions() {
return Codegen.optional(this.computeRackDefinitions);
}
/**
* The current detailed status of the cluster.
*
*/
@Export(name="detailedStatus", refs={String.class}, tree="[0]")
private Output detailedStatus;
/**
* @return The current detailed status of the cluster.
*
*/
public Output detailedStatus() {
return this.detailedStatus;
}
/**
* The descriptive message about the detailed status.
*
*/
@Export(name="detailedStatusMessage", refs={String.class}, tree="[0]")
private Output detailedStatusMessage;
/**
* @return The descriptive message about the detailed status.
*
*/
public Output detailedStatusMessage() {
return this.detailedStatusMessage;
}
/**
* The extended location of the cluster manager associated with the cluster.
*
*/
@Export(name="extendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output extendedLocation;
/**
* @return The extended location of the cluster manager associated with the cluster.
*
*/
public Output extendedLocation() {
return this.extendedLocation;
}
/**
* Field Deprecated. This field will not be populated in an upcoming version. The extended location (custom location) that represents the Hybrid AKS control plane location. This extended location is used when creating provisioned clusters (Hybrid AKS clusters).
*
*/
@Export(name="hybridAksExtendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output hybridAksExtendedLocation;
/**
* @return Field Deprecated. This field will not be populated in an upcoming version. The extended location (custom location) that represents the Hybrid AKS control plane location. This extended location is used when creating provisioned clusters (Hybrid AKS clusters).
*
*/
public Output hybridAksExtendedLocation() {
return this.hybridAksExtendedLocation;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The configuration of the managed resource group associated with the resource.
*
*/
@Export(name="managedResourceGroupConfiguration", refs={ManagedResourceGroupConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ ManagedResourceGroupConfigurationResponse> managedResourceGroupConfiguration;
/**
* @return The configuration of the managed resource group associated with the resource.
*
*/
public Output> managedResourceGroupConfiguration() {
return Codegen.optional(this.managedResourceGroupConfiguration);
}
/**
* The count of Manual Action Taken (MAT) events that have not been validated.
*
*/
@Export(name="manualActionCount", refs={Double.class}, tree="[0]")
private Output manualActionCount;
/**
* @return The count of Manual Action Taken (MAT) events that have not been validated.
*
*/
public Output manualActionCount() {
return this.manualActionCount;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The resource ID of the Network Fabric associated with the cluster.
*
*/
@Export(name="networkFabricId", refs={String.class}, tree="[0]")
private Output networkFabricId;
/**
* @return The resource ID of the Network Fabric associated with the cluster.
*
*/
public Output networkFabricId() {
return this.networkFabricId;
}
/**
* The provisioning state of the cluster.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the cluster.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The settings for cluster runtime protection.
*
*/
@Export(name="runtimeProtectionConfiguration", refs={RuntimeProtectionConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ RuntimeProtectionConfigurationResponse> runtimeProtectionConfiguration;
/**
* @return The settings for cluster runtime protection.
*
*/
public Output> runtimeProtectionConfiguration() {
return Codegen.optional(this.runtimeProtectionConfiguration);
}
/**
* The configuration for use of a key vault to store secrets for later retrieval by the operator.
*
*/
@Export(name="secretArchive", refs={ClusterSecretArchiveResponse.class}, tree="[0]")
private Output* @Nullable */ ClusterSecretArchiveResponse> secretArchive;
/**
* @return The configuration for use of a key vault to store secrets for later retrieval by the operator.
*
*/
public Output> secretArchive() {
return Codegen.optional(this.secretArchive);
}
/**
* The support end date of the runtime version of the cluster.
*
*/
@Export(name="supportExpiryDate", refs={String.class}, tree="[0]")
private Output supportExpiryDate;
/**
* @return The support end date of the runtime version of the cluster.
*
*/
public Output supportExpiryDate() {
return this.supportExpiryDate;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The strategy for updating the cluster.
*
*/
@Export(name="updateStrategy", refs={ClusterUpdateStrategyResponse.class}, tree="[0]")
private Output* @Nullable */ ClusterUpdateStrategyResponse> updateStrategy;
/**
* @return The strategy for updating the cluster.
*
*/
public Output> updateStrategy() {
return Codegen.optional(this.updateStrategy);
}
/**
* The list of workload resource IDs that are hosted within this cluster.
*
*/
@Export(name="workloadResourceIds", refs={List.class,String.class}, tree="[0,1]")
private Output> workloadResourceIds;
/**
* @return The list of workload resource IDs that are hosted within this cluster.
*
*/
public Output> workloadResourceIds() {
return this.workloadResourceIds;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Cluster(java.lang.String name) {
this(name, ClusterArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Cluster(java.lang.String name, ClusterArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Cluster(java.lang.String name, ClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:networkcloud:Cluster", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Cluster(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:networkcloud:Cluster", name, null, makeResourceOptions(options, id), false);
}
private static ClusterArgs makeArgs(ClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ClusterArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:networkcloud/v20230701:Cluster").build()),
Output.of(Alias.builder().type("azure-native:networkcloud/v20231001preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:networkcloud/v20240601preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:networkcloud/v20240701:Cluster").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Cluster get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Cluster(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy